In this tutorial we will learn to create a custom Video Player that plays youtube videos in background also. To do that we will be using youtube-ios-player-helper.
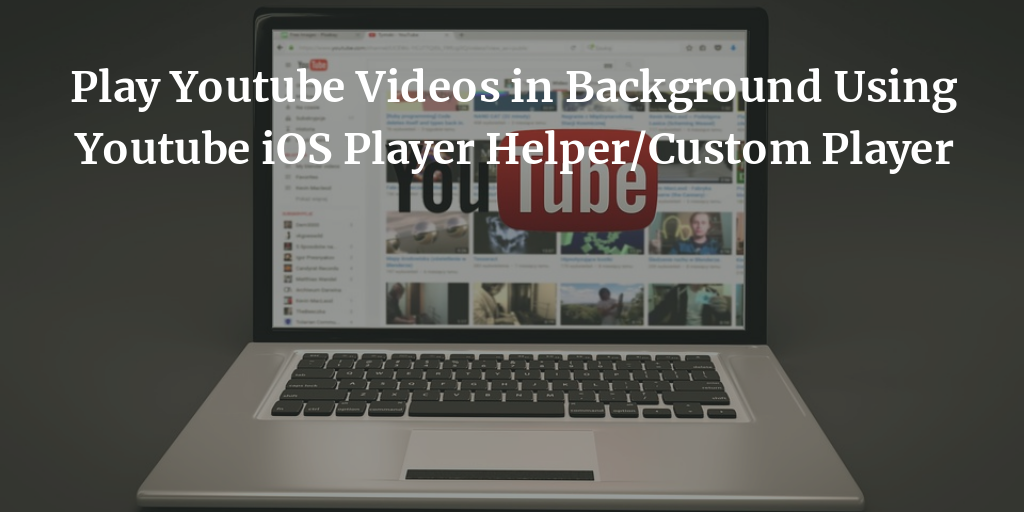
Let's first know what youtube-ios-player-helper is? It is an open source library for iOS developers to help them embeding a YouTube iframe player into an iOS application or we can say that, it is a helper library for those iOS developers who are looking to add YouTube video playback in their applications via the iframe player in a UIWebView.
Now, follow these steps:
1- We can achieve this using "youtube-ios-player-helper".
2- Basic setup for project- Create a new project in Xcode
3- Now we have to install the pods in our project for that jump to project directory.
4- Run the followings commands
a) pod init
b) open podfile
c) Your empty podfile will look like this
# Uncomment the next line to define a global platform for your project
# platform :ios, '9.0'
target 'YourProjectName' do
# Comment the next line if you're not using Swift and don't want to use dynamic frameworks
use_frameworks!
# Pods for YourProjectName
end
Now add the line pod "youtube-ios-player-helper" , '0.1.6' below user_frameworks!
now your podfile will look like this
# Uncomment the next line to define a global platform for your project
# platform :ios, '9.0'
target 'YourProjectName' do
# Comment the next line if you're not using Swift and don't want to use dynamic frameworks
use_frameworks!
pod "youtube-ios-player-helper" , '0.1.6'
# Pods for YourProjectName
end
d) now run pod install
5- Close your current project and open yourProject.xcworkspace and now we are ready to write code and setup UI.
6- In the main.storyboard add a UIView and set the autolayout for that according to your need.
7- Now, on the Identity Inspector of UIView class to YTPlayerView
8- Move to viewController.swift file and add these lines
import UIKit
import youtube_ios_player_helper
class VideoViewController:UIViewController , YTPlayerViewDelegate{
@IBOutlet weak var videoPlayerView: YTPlayerView! //outlet for view
override func viewDidLoad() {
super.viewDidLoad()
videoPlayerView.delegate = self // setting delegate for VideoPlayer
//playervars are the supported parameters for videoview so that it not play directly on fullscreen on load
videoPlayerView.load(withVideoId: "xxxxxxxxx", playerVars: ["playsinline": 1])
}
above code will run the video having the VideoId :- "xxxxxxxxx" on running the app by tapping on video.
9. To play the video directly after loading the view we have to add the following method
//Invoked when the player view is ready to receive API calls.
func playerViewDidBecomeReady(_ playerView: YTPlayerView) {
videoView.playVideo()
}
10. Now, to play video on background we have to add the following in our controller
func playInBackground(){
DispatchQueue.main.asyncAfter(deadline: .now()) {
self.videoPlayerView.playVideo()
}
}
and for calling the function we have to add below line in viewDidLoad(). After which code will be like
NotificationCenter.default.addObserver(self, selector: #selector(VideoViewController.playInBackground), name: Notification.Name.UIApplicationDidEnterBackground, object: nil)
11. Run your project.
Your final snapshot for the controller will look like this
import UIKit
import youtube_ios_player_helper
class VideoViewController:UIViewController , YTPlayerViewDelegate{
@IBOutlet weak var videoPlayerView: YTPlayerView! //outlet for view
override func viewDidLoad() {
super.viewDidLoad()
// calling method when app is in background
NotificationCenter.default.addObserver(self, selector: #selector(VideoViewController.playInBackground), name: Notification.Name.UIApplicationDidEnterBackground, object: nil)
videoPlayerView.delegate = self // setting delegate for VideoPlayer
//playervars are the supported parameters for videoview so that it not play directly on fullscreen on load
videoPlayerView.load(withVideoId: "xxxxxxxxx", playerVars: ["playsinline": 1])
videoView.playVideo()
}
//Invoked when the player view is ready to receive API calls.
func playerViewDidBecomeReady(_ playerView: YTPlayerView) {
videoPlayerView.playVideo()
}
func playInBackground(){
DispatchQueue.main.asyncAfter(deadline: .now() + .milliseconds(0)) {
self.videoPlayerView.playVideo()
}
}
}
0 Comment(s)