Swift is faster than Objective-C and programmers prefer Swift for iOS development. Apple has aimed big goals with Swift but some programmers are still using Objective-C.
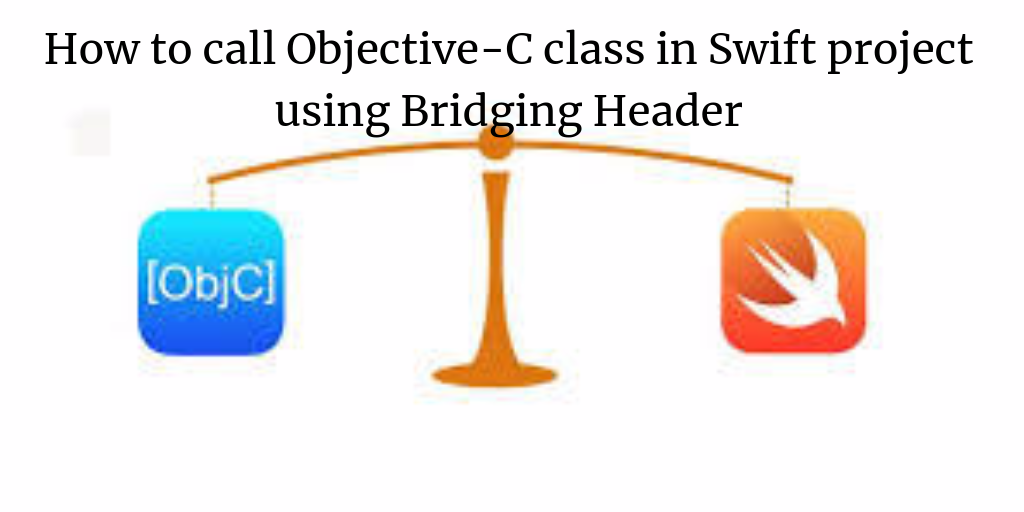
Here is an example of calling Objective-C code in Swift. According to the Apple's doc, we can use both languages in one project using Bridging Header. Please follow the steps as mentioned below.
Step 1- Add Objective-C class (.m file) into your Swift project. Name it 'CustomClass.m'
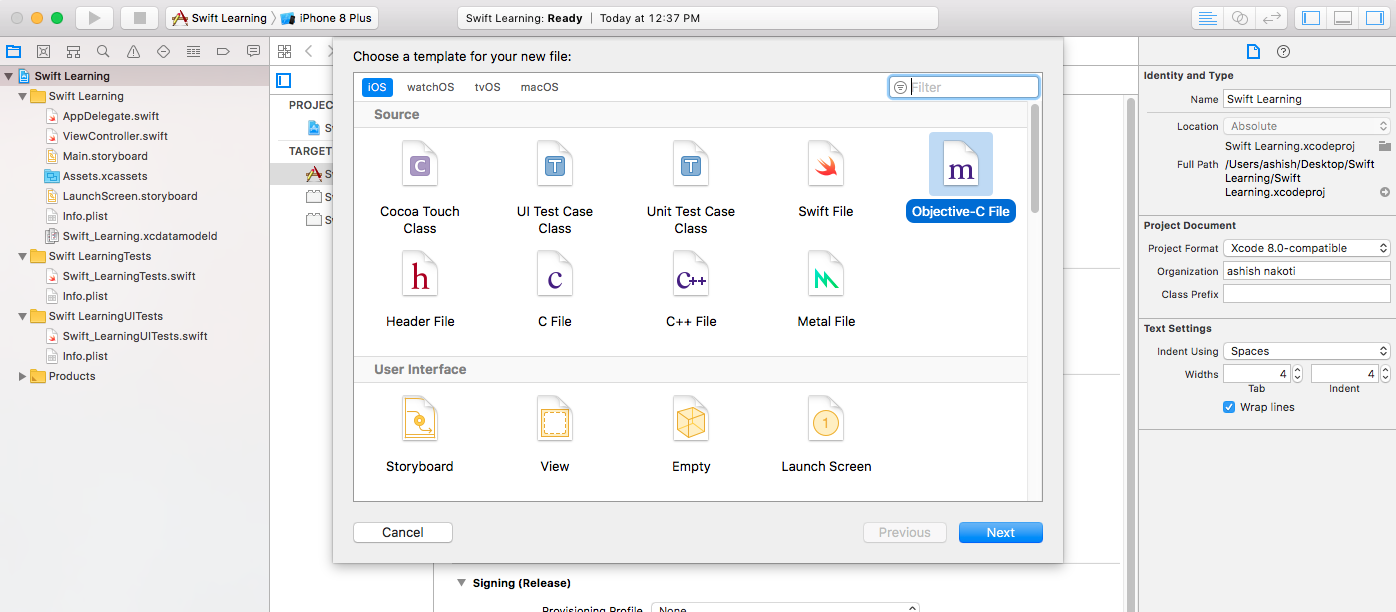
Step 2- Once you create an Objective-C file, XCode prompts you to configure a Bridging Header. ('Would you like to configure an Objective-C bridging header?')
Now click 'Create Bridging Header'
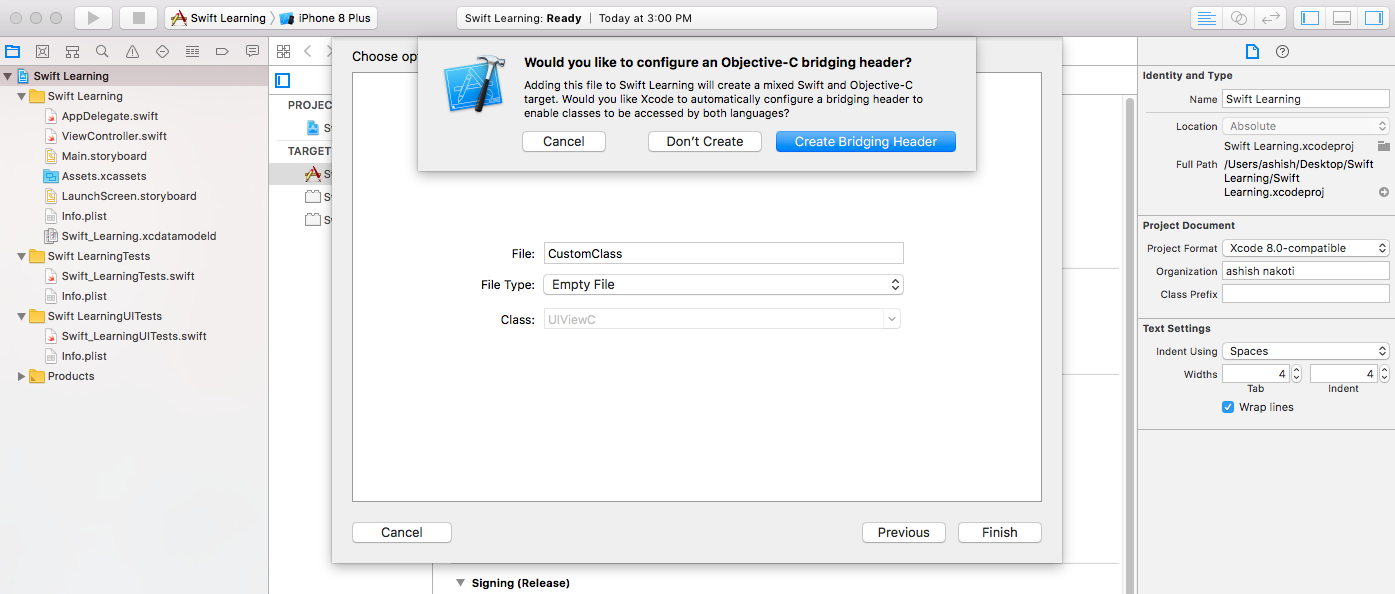
Step 3- Now create header (.h file) and name it 'CustomClass.h'.
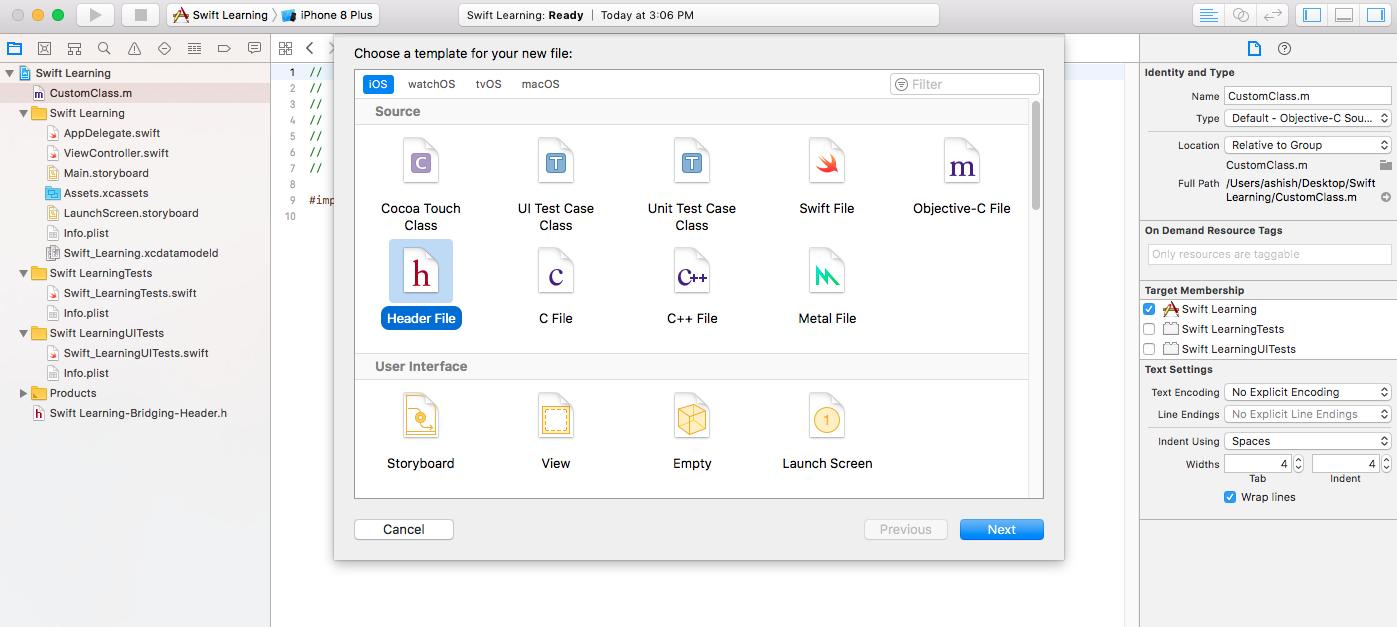
Step 4- In the Bridging Header file import Objective-C class now. Like I mentioned below.
//
// Use this file to import your target's public headers that you would like to expose to Swift.
//
#import "CustomClass.h"
Step -5 Now write some Objective-C code in your CustomClass files. Lets begin with header (CustomClass.h).
This is how your header file look like...
#import <Foundation/Foundation.h>
@interface CustomClass : NSObject
@property (strong, nonatomic) id objcProperty;
- (void) objcMethod;
@end
Step 6- In 'CustomClass.m' write below code line.
#import <Foundation/Foundation.h>
#import "CustomClass.h"
@implementation CustomClass
- (void) objcMethod {
NSLog(@"Objc class Method called by swift class.");
}
@end
Step 7- In your Swift class call Objective-C method now. Write below code in your Swift controller. I am calling Objective-C class method (objcMethod) in 'ViewDidLoad' method of the swift class.
import UIKit
class ViewController: UIViewController {
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view, typically from a nib.
let instanceOfCustomClass = CustomClass()
instanceOfCustomClass.objcProperty = "Hello Objective-C"
instanceOfCustomClass.objcMethod()
}
Please test the above code by applying breakpoints in xcode project. Feel free to ask questions in the comments section.
Happy Coding!!!
0 Comment(s)