To add an event to iOS calendar we need to access calendar of the iPhone without accessing calendar we can not add events in iPhone.
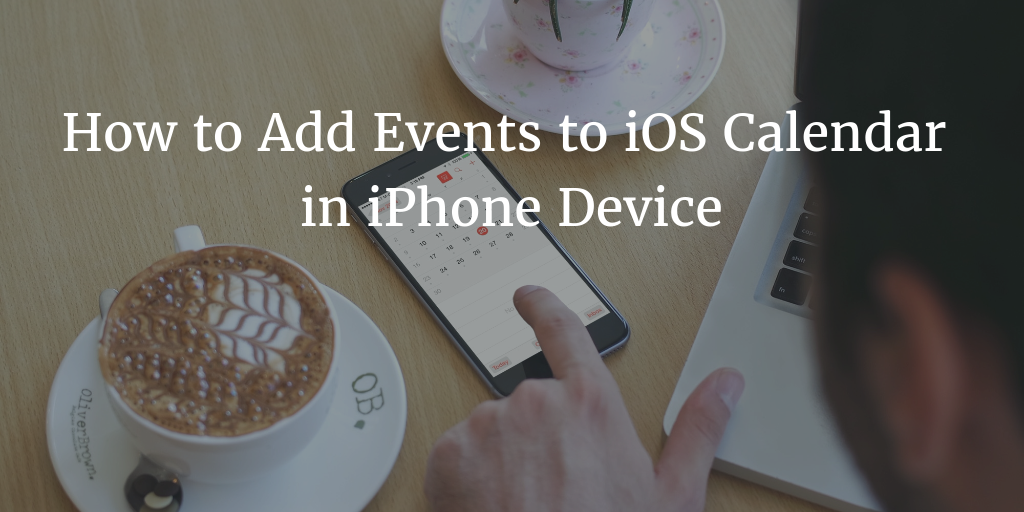
For accessing iPhone calendar we have to ask for permissions first. Here permission means allowing the particular app or functionality officially to do a particular thing on your device/system like setting up event and alert on your device or accessing your data, location etc.
So for requesting permissions in iPhone you can use below code example:
func isCalendarAccesible(completion: @escaping (( _ isAllowed:Bool , _ error:Error?)->Void)) {
let status = EKEventStore.authorizationStatus(for: .event)
switch (status){
case .notDetermined:
// This happens on first-run
requestAccessToCalendar(completion: completion)
case .authorized:
// User has access
print("User has access to calendar")
completion(true, nil)
case EKAuthorizationStatus.restricted, EKAuthorizationStatus.denied:
// We need to help them give us permission
noPermission()
completion(false, nil)
}
}
func noPermission() {
print("User has to change settings...goto settings to view access")
}
func requestAccessToCalendar(completion: @escaping (( _ isAllowed:Bool , _ error:Error?)->Void)) {
EventHelper.appleEventStore.requestAccess(to: .event, completion: { (granted, error) in
if (granted) && (error == nil) {
DispatchQueue.main.async {
print("User has access to calendar")
completion(true, nil)
}
} else {
DispatchQueue.main.async{
self.noPermission()
completion(false, error)
}
}
})
}
func addAppleEvents(titile:String,startDate:Date,endDate:Date,notes:String) {
let event:EKEvent = EKEvent(eventStore: EventHelper.appleEventStore)
event.title = titile
event.startDate = startDate
event.endDate = endDate
event.notes = notes
event.calendar = EventHelper.appleEventStore.defaultCalendarForNewEvents
event.calendar.title = "AppTitle"
do {
let interval:TimeInterval = 0;
let alarm = EKAlarm(relativeOffset: interval)
event.addAlarm(alarm)
try EventHelper.appleEventStore.save(event, span: .thisEvent)
print("events added with dates:")
} catch let e as NSError {
print("Error in saving events" + e.description)
return
}
print("Saved Event")
}
Once above code is excuted you have to call the below method, to call this method by using the below code:
isCalendarAccesible { (allowed, error) in
//first we will check if permission is granted to app for accessing our iPhone calendar. If it is true then we can create our event from following code
if allowed == true {
addAppleEvents(titile: "birthday Testing event for my app", startDate: YOUR_EVENT_START_DATE , endDate:YOUR_EVENT_END_DATE,notes: "birthday testing event")
}
}
Output: After calling the method by above process, you will get output as in below screenshot, to see the output you have to open your iPhone calendar
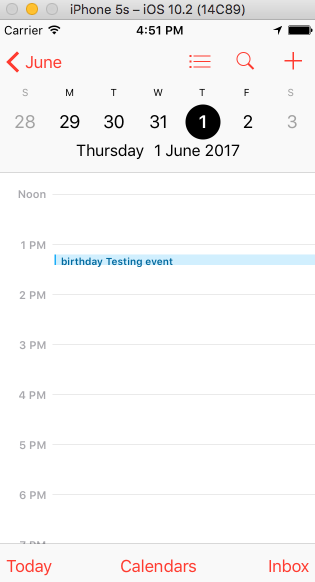
0 Comment(s)