Hello Readers!!
Today we are going to discuss about the AdMob SDK intergration with Unity3D. Its very easy and straight forward.
You can download the latest AdMob plugin from here :-
https://github.com/googleads/googleads-mobile-unity/releases/tag/v3.0.6
Once you download the package then follow following steps:-
- Create Ad On AdMob Account:- You need to create a app on the AdMob account. You can create Banner, interstitial or Rewarded Ads.
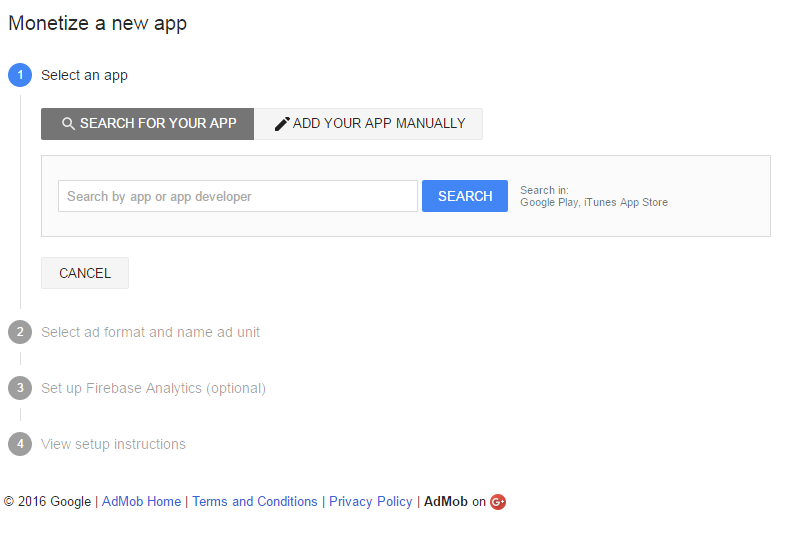
- Get AppID :- Now fetch App ID from the AdMob Account.

- Create New Add Source:- We can add more add source to get more eCPM. Below is the example to add more source,
-
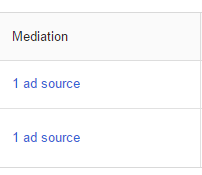
This can increase your eCPM.
Now next is your Unity Side code. Here is the source code:-
using UnityEngine;
using System.Collections;
using System;
using System.Collections.Generic;
using admob;
public class admobdemo : MonoBehaviour {
// Use this for initialization
void Start ()
{
Admob ad = Admob.Instance();
Admob.Instance().bannerEventHandler += onBannerEvent;
Admob.Instance().interstitialEventHandler += onInterstitialEvent;
Admob.Instance().rewardedVideoEventHandler += onRewardedVideoEvent;
}
void OnGUI()
{
if (GUI.Button (new Rect (0, 0, 100, 60), "initadmob"))
{
Admob ad = Admob.Instance();
ad.initAdmob("ca-app-pub-xxxxxxxxxx/xxxxxxx", "ca-app-pub-XXXX/XXXXXX");
ad.loadRewardedVideo("ca-app-pub-6414775745116351/5906861026");
ad.setTesting(true);
}
if (GUI.Button(new Rect(120, 0, 100, 60), "showInterstitial"))
{
Admob ad = Admob.Instance();
if (ad.isInterstitialReady())
{
ad.showInterstitial();
}
else
{
ad.loadInterstitial();
}
}
if (GUI.Button(new Rect(240, 0, 100, 60), "showRewardVideo"))
{
Admob ad = Admob.Instance();
if (ad.isRewardedVideoReady())
{
ad.showRewardedVideo();
}
else
{
try
{
Admob.Instance().rewardedVideoEventHandler += onRewardedVideoEvent;
ad.loadRewardedVideo("ca-app-pub-xxxxxx/xxxxx");
}
catch(Exception e){_l += "\nLoaded......"+e.Message;}
}
}
if (GUI.Button(new Rect(240, 100, 100, 60), "showbanner"))
{
Admob.Instance().showBannerRelative(AdSize.Banner, AdPosition.BOTTOM_CENTER, 0);
}
if (GUI.Button(new Rect(240, 200, 100, 60), "showbannerABS"))
{
Admob.Instance().showBannerAbsolute(AdSize.Banner, 0, 30);
}
if (GUI.Button(new Rect(240, 300, 100, 60), "hidebanner"))
{
Admob.Instance().removeBanner();
}
}
void onInterstitialEvent(string eventName, string msg)
{
Debug.Log("handler onAdmobEvent---" + eventName + " " + msg);
if (eventName == AdmobEvent.onAdLoaded)
{
Admob.Instance().showInterstitial();
}
}
void onBannerEvent(string eventName, string msg)
{
Debug.Log("handler onAdmobBannerEvent---" + eventName + " " + msg);
}
void onRewardedVideoEvent(string eventName, string msg)
{
Debug.Log("\nhandler onRewardedVideoEvent---" + eventName + " " + msg);
}
}
You can use this code and have fun.
Till then keep coding..
0 Comment(s)