Many a time we need to take a screenshot of a screen on an iOS device. So In this blog, we will discuss how to capture a screenshot of a particular screen through an application and with the help of an example this concept can easily be understood.
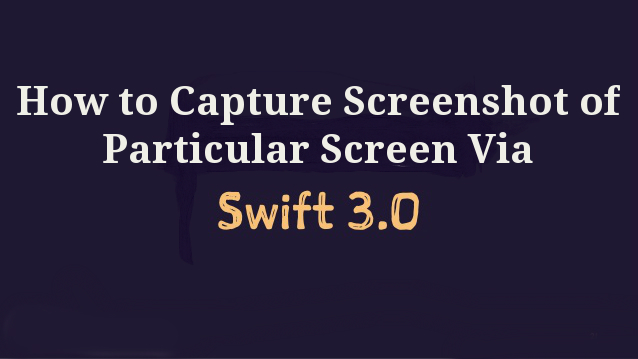
In this example, in one screen we are going to take 3 buttons with the different functionality of each and every single button. First button functionality includes the start of capturing screenshot of that particular screen only, the second button is to stop the application to capture the screenshots and the third button is to show all the screenshot taken on the next screen.
Step 1: Below is the code of the first controller in which we have taken all the three button and their functionality. In the code, all the three buttons are mentioned with the particular functionality.
First button functionality includes calling of function which is used to capture the screenshot of the same screen multiple times until and unless we press the stop button. After stopping the capture screenshot functionality user can view the all captured screenshot on next screen.
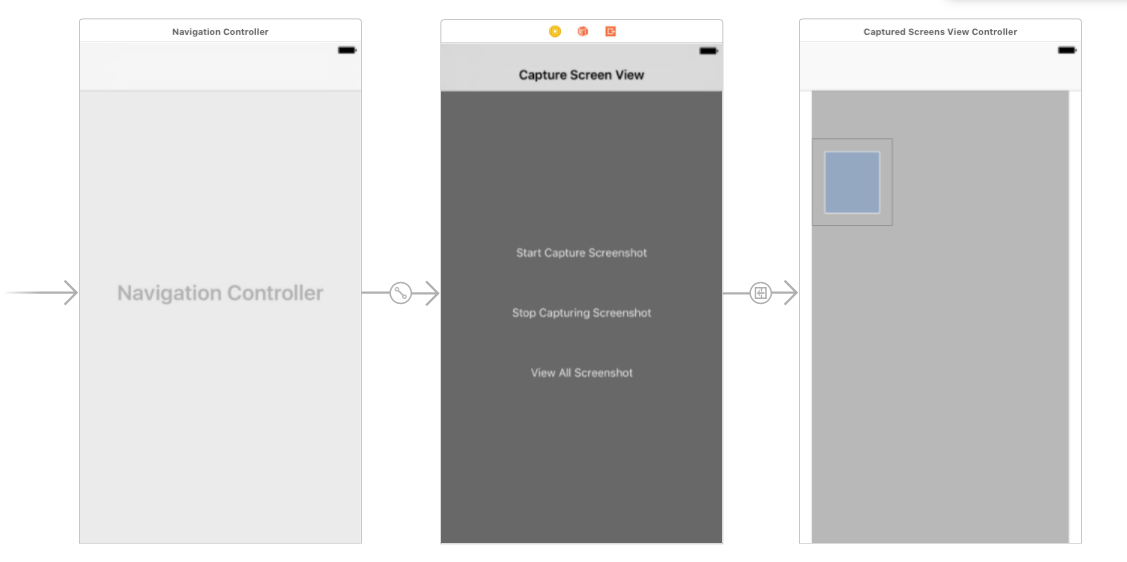
//
// ViewController.swift
// CaptureScreenShot
//
// Created by elcaptain1 on 8/22/17.
// Copyright 2017 Tanuja. All rights reserved.
//
import UIKit
class ViewController: UIViewController {
var timer = Timer()
var array = [UIImage]()
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view, typically from a nib.
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
func scheduledTimerWithTimeInterval(){
// Scheduling timer to Call the function "updateCounting" with the interval of 1 seconds
timer = Timer.scheduledTimer(timeInterval: 1, target: self, selector: #selector(self.captureScreen), userInfo: nil, repeats: true)
}
func captureScreen() -> UIImage? {
UIGraphicsBeginImageContextWithOptions(view.bounds.size, false, UIScreen.main.scale)
view.layer.render(in: UIGraphicsGetCurrentContext()!)
let image = UIGraphicsGetImageFromCurrentImageContext()
UIGraphicsEndImageContext()
array.append(image!)
print(image)
return image
}
@IBAction func btnStartCapture(_ sender: AnyObject) {
scheduledTimerWithTimeInterval()
}
@IBAction func btnStopTimer(_ sender: AnyObject) {
timer.invalidate()
}
@IBAction func btnViewAllScreenshot(_ sender: AnyObject) {
let storyboard = UIStoryboard(name: "Main", bundle: nil)
let vc = storyboard.instantiateViewController(withIdentifier: "CapturedScreensViewController") as! CapturedScreensViewController
vc.arrayOfCapturedImage = array
self.present(vc, animated: true, completion: nil)
}
}
Step 2: Take CollectionView on next controller where we can see all the captured screenshots and take imageview inside cell and create an outlet of imageview as given below:-
//
// CollectionViewCell.swift
// CaptureScreenShot
//
// Created by elcaptain1 on 8/22/17.
// Copyright 2017 Tanuja. All rights reserved.
//
import UIKit
class CollectionViewCell: UICollectionViewCell {
@IBOutlet weak var screenShotImageView: UIImageView!
}
Step 3: Now in CapturedScreensViewController use CollectionView datasource and delegate methods to show all the captured screenshots.
//
// CapturedScreensViewController.swift
// CaptureScreenShot
//
// Created by elcaptain1 on 8/22/17.
// Copyright 2017 Tanuja. All rights reserved.
//
import UIKit
class CapturedScreensViewController: UIViewController,UICollectionViewDelegate,UICollectionViewDataSource {
var arrayOfCapturedImage = [UIImage]()
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view.
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
// tell the collection view how many cells to make
func collectionView(_ collectionView: UICollectionView, numberOfItemsInSection section: Int) -> Int {
return arrayOfCapturedImage.count
}
// make a cell for each cell index path
func collectionView(_ collectionView: UICollectionView, cellForItemAt indexPath: IndexPath) -> UICollectionViewCell {
// get a reference to our storyboard cell
let cell = collectionView.dequeueReusableCell(withReuseIdentifier: "cell", for: indexPath as IndexPath) as! CollectionViewCell
// Use the outlet in our custom class to get a reference to the UILabel in the cell
cell.screenShotImageView.image = arrayOfCapturedImage[indexPath.row]
return cell
}
}
In this way you can easily implement the concept of capture screenshot from the particular list. Output screenshots are given below:-
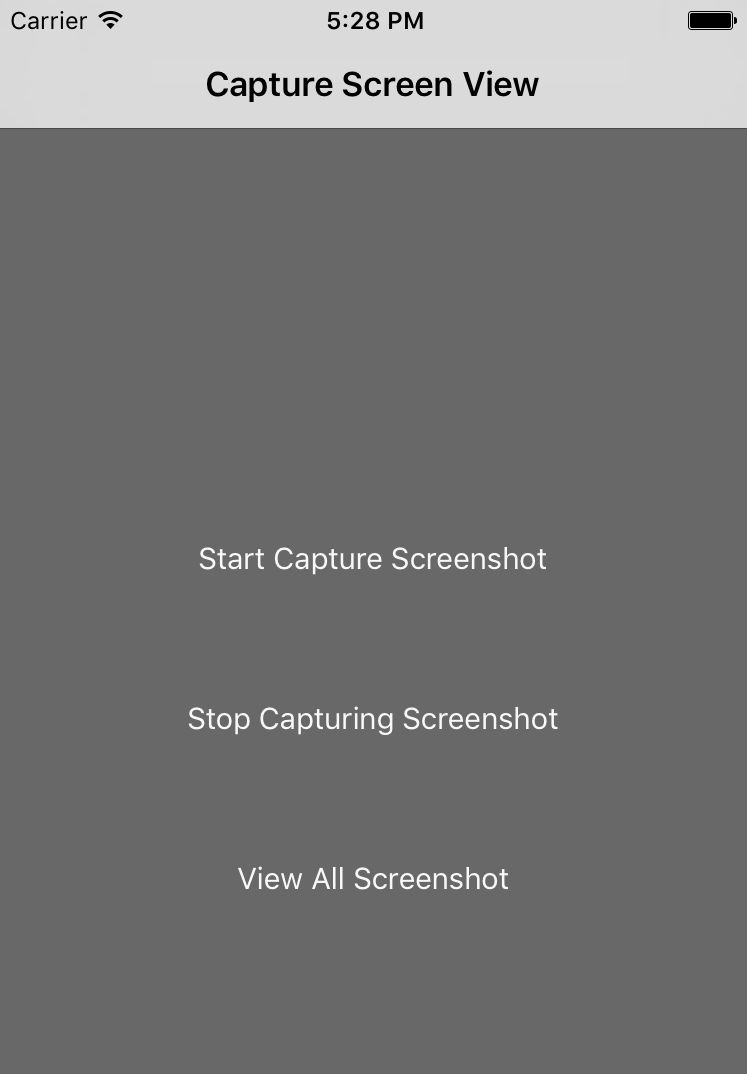
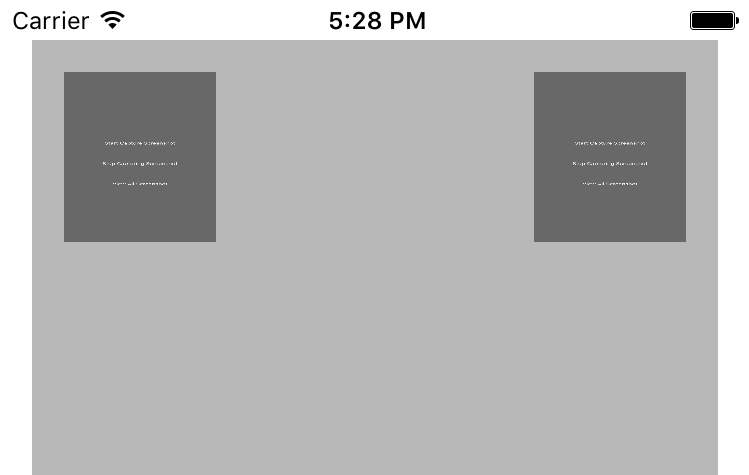
You can also download the sample code from the link given below, for any questions feel free to write in comment.
0 Comment(s)