The important announcement by Google in 2017 for Android Developers is that kotlin is now an official language for Android App Development. For more on introduction and basic you can visit previous blog "Getting Started with Kotlin for Android App Development - An Introduction and Basics".
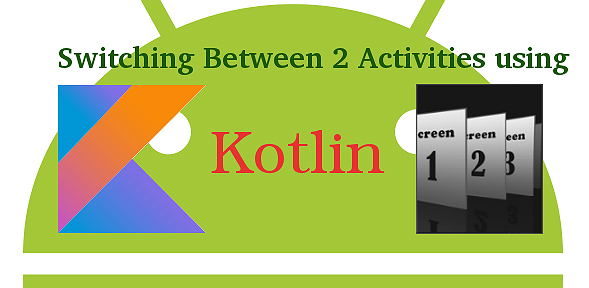
Coming to the topic, today in this tutorial, I will guide you about how we can navigate/switch and sending data between two activities. So, being an android developer you must have sent data between two activities but in kotlin the syntax's are different.
Let's see an example of two activities, in the first one, Data is sent to another activity and in next one Data is received:
Activity 1: Send data to another activity
package com.multipleactivities.android.activity
import android.content.Intent
import android.os.Bundle
import android.support.v7.app.AppCompatActivity
import android.widget.Button
import android.widget.EditText
import com.multipleactivities.android.R
import com.multipleactivities.android.constants.AppConstants
class FirstActivity : AppCompatActivity() {
private var btnNavigate: Button? = null
private var etWriteText: EditText? = null
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_first)
// initializing button
initView()
// button click
define()
}
private fun initView() {
btnNavigate = findViewById(R.id.btn_navigate) as Button
etWriteText = findViewById(R.id.et_write_text) as EditText
}
private fun define() {
btnNavigate!!.setOnClickListener {
val intent = Intent(this@FirstActivity, SecondActivity::class.java)
intent.putExtra(AppConstants.VALUE, etWriteText!!.text.toString())
startActivity(intent)
}
}
}
In above code, you can see the kotlin syntax, we are sending user input to the another activity through intent.
Activity 2: Receiving Data
package com.multipleactivities.android.activity
import android.os.Bundle
import android.support.v7.app.AppCompatActivity
import android.widget.TextView
import com.multipleactivities.android.R
import com.multipleactivities.android.constants.AppConstants
class SecondActivity : AppCompatActivity() {
private var tvReceivedText: TextView? = null
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_second)
// initializing views
initView()
// receiving intent values
receivingValue()
}
private fun receivingValue() {
val intent = intent
val value = intent.getStringExtra(AppConstants.VALUE)
tvReceivedText!!.text = value
}
private fun initView() {
tvReceivedText = findViewById(R.id.tv_received_text) as TextView
}
}
In above code, you can see we getting the data using get intent and displaying it in textView.
0 Comment(s)