RecyclerView has a many good features which took it over listview but the one feature that was missing in RecyclerView is Fast Scrolling. So, now with Support library 26, we can also use fast scrolling with recyclerview like listview.
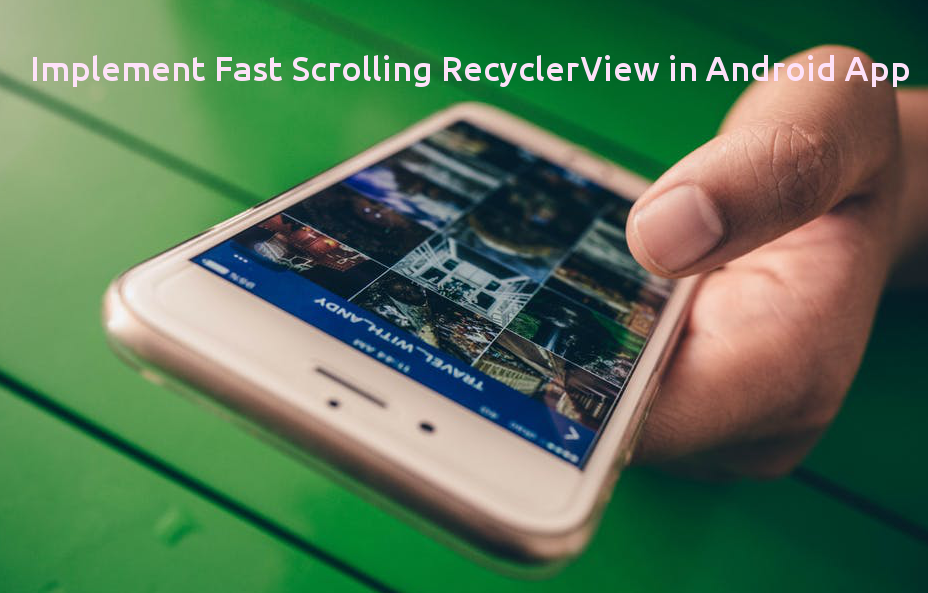
Fastscrolling gives a pace to the scroller which make the user to easily navigate the page up and down more faster
This is being used in listview.
listView = (ListView) findViewById(R.id.listView);
listView.setFastScrollEnabled(true);
Make sure to use the Support Library 26. Add dependency in build.gradle file like:
dependencies {
compile 'com.android.support:design:26.0.1'
compile 'com.android.support:recyclerview-v7:26.0.1'
}
Support Library 26 has moved to Google’s maven repository center, add it in build.gradle
buildscript {
repositories {
google()
}
}
//recyclerview xml
<?xml version="1.0" encoding="utf-8"?>
<android.support.constraint.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
app:layout_behavior="@string/appbar_scrolling_view_behavior"
tools:context="com.shaishavgandhi.fastscrolling.MainActivity"
tools:showIn="@layout/activity_main">
<android.support.v7.widget.RecyclerView
android:id="@+id/recyclerView"
android:layout_width="match_parent"
android:layout_height="wrap_content">
</android.support.v7.widget.RecyclerView>
</android.support.constraint.ConstraintLayout>
In the above code: app:fastScrollEnabled="true" //this line will enable the fast scroll for listview.
app:fastScrollHorizontalThumbDrawable="@drawable/thumb_drawable"
//thumb drawable will be shown using fastScrollHorizontalThumbDrawable.
let’s enable the fast scrolling feature. The xml file looks like:
<?xml version="1.0" encoding="utf-8"?>
<android.support.constraint.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
app:layout_behavior="@string/appbar_scrolling_view_behavior"
tools:context="com.shaishavgandhi.fastscrolling.MainActivity"
tools:showIn="@layout/activity_main">
<android.support.v7.widget.RecyclerView
android:id="@+id/recyclerView"
android:layout_width="match_parent"
android:layout_height="wrap_content"
app:fastScrollEnabled="true"
app:fastScrollHorizontalThumbDrawable="@drawable/thumb_drawable"
app:fastScrollHorizontalTrackDrawable="@drawable/line_drawable"
app:fastScrollVerticalThumbDrawable="@drawable/thumb_drawable"
app:fastScrollVerticalTrackDrawable="@drawable/line_drawable">
</android.support.v7.widget.RecyclerView>
</android.support.constraint.ConstraintLayout>
In the above code:
- fastScrollVerticalTrackDrawable: A Drawable that will be used to draw the line that will represent the scrollbar on vertical axis.
- fastScrollEnabled: boolean to enable the fast scrolling.
- fastScrollHorizontalThumbDrawable: A Drawable that will be used to show the thumb
//different drawable used in recycler view
//line_drawable.xml
<?xml version="1.0" encoding="utf-8"?>
<selector xmlns:android="http://schemas.android.com/apk/res/android">
<item
android:state_pressed="true"
android:drawable="@drawable/line"/>
<item
android:drawable="@drawable/line"/>
</selector>
//line.xml
<?xml version="1.0" encoding="utf-8"?>
<shape xmlns:android="http://schemas.android.com/apk/res/android"
android:shape="rectangle">
<solid android:color="@android:color/darker_gray" />
<padding
android:top="2dp"
android:left="3dp"
android:right="4dp"
android:bottom="5dp"/>
</shape>
//thumb_drawable.xml
<?xml version="1.0" encoding="utf-8"?>
<selector xmlns:android="http://schemas.android.com/apk/res/android">
<item
android:state_pressed="true"
android:drawable="@drawable/tumb"/>
<item
android:drawable="@drawable/tumb"/>
</selector>
//thub.xml
<?xml version="1.0" encoding="utf-8"?>
<shape xmlns:android="http://schemas.android.com/apk/res/android"
android:shape="rectangle">
<corners
android:topLeftRadius="22dp"
android:topRightRadius="22dp"
android:bottomLeftRadius="22dp" />
<padding
android:paddingLeft="11dp"
android:paddingRight="11dp" />
<solid android:color="@color/colorPrimaryDark" />
</shape>
That's all about fast scrolling feature, If you have any questions, please feel free to post in comments.
Happy coding;)
0 Comment(s)