Callback is a way to inform a class synchronous/ asynchronous when an event occurs. A simple way to create a generic callback is to use interface with a method in which JSON is passed.
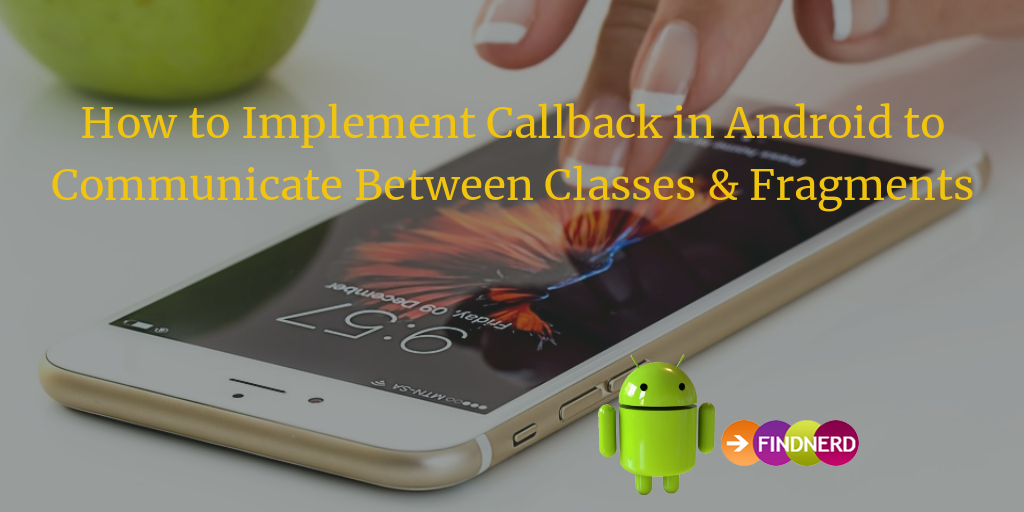
So, In this tutorial, I will guide you about how to implement callback in Android. You can use this callback to communicate between classes, fragments or adapters.
Let's see with example:-
public interface CallBacks {
void callbackObserver(JSONObject obj);
}
Now, we are going to use this callback in our class as follow:-
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
loginButtonClicked(new Callbacks() {
@Override
public void callbackObserver(JSONObject obj) {
//Do work after login
}
});
}
void loginButtonClicked(Callbacks callback){
//Api request to login or any kind of work
JSONObject object = new JSONObject();
try {
object.put("name", "Jagandeep Singh");
}catch (JSONException exception){
exception.printStackTrace();
}
callback.callbackObserver(object);
}
}
In above example, we have passed a callback as a parameter to function and after some task or after an event, we are calling the callback method with some information.
0 Comment(s)