Before Creating a Simple Twitter Bot with Node.js let us first know what twitter bot is and what it does?
The Twitter bot is a software program you write that listen to something to happen and carry out the action in response. It will listen on basis of hashtag and then retweet, favourites, and replies to other users if they follow it. In other words, we can say that It is such software program created to do the automated task, like sending tweets on the particular time schedule or sending reply on message or to retweet, which makes the user work easy.
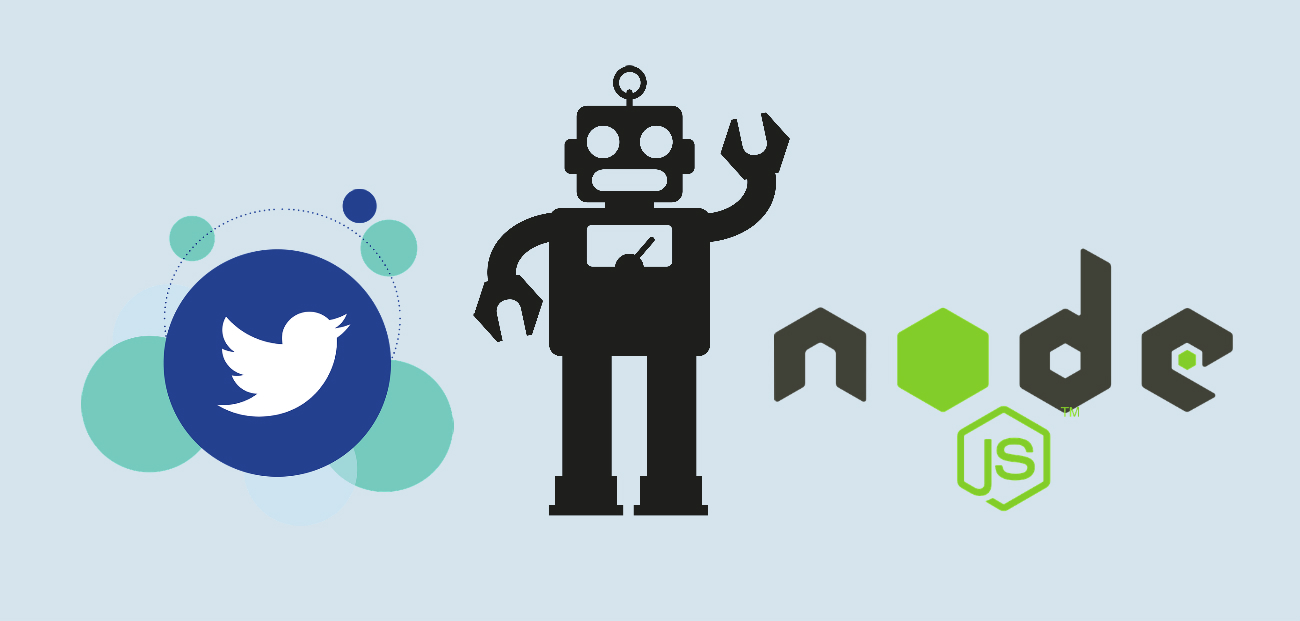
Now lets create a simple twitter bot
To create bot first we need the following two thing,
1. Twitter Account (You must have twitter account or create twitter account first)
2. Node.js (Nodejs should be installed in your system)
Let's started configuring our bot-
Step 1: Create an empty directory and initialize it with
$npm init
Fill required field. It will create your package.json file. Your package.json file will look like:
{
"name": "twitter-bot",
"version": "1.0.0",
"description": "twitter bot",
"main": "app.js",
"scripts": {
"test": "echo \"Error: no test specified\" && exit 1"
},
"author": "neelam",
"license": "ISC",
"dependencies": {
"twitter": "^1.7.0"
}
}
Step 2: Create two javascript file config.js and app.js. The app.js have code for twitter bot and config.js file will contain configuration data.
Step 3: Your Directory structure will look like:
root/project-name
|-bot.js
|-config.js
|-package.json
Step 4: Create an application by link https://apps.twitter.com/app/new, and fill details required. Once you created application, look for ‘Keys and Access Tokens under nav-panes and
Click on ‘Generate Token Actions’ and then copy:
· Consumer Key
· Consumer Secret
· Access Token
· Access Token Secret
Paste and edit these values in config.js
// config.js
module.exports = {
consumer_key: '',
consumer_secret: '',
access_token_key: '',
access_token_secret: ''
}
Step 5: Install dependency that will be needed in our application
$ npm install --save twit
Step 6: Defined required dependency in config.js file
var Twit = require('twit');
var config = require('./config.js');
Step 7: Now Creating retweet bot, that will find the latest news. We will take params object that will take properties initialize by us.
var params = {
q: '#nodejs',
count: 10,
result_type: 'recent',
lang: 'en'
}
In the above code, q is the required parameter which is used to store search query. It will search for tweets containing #nodejs. Where count is the number of tweets, result_type:’recent’ will return the most recent results and lang:’en’ returns English results.
Step 8: Paste the below code in the file app.js.
//Require dependencies
var Twitter = require('twit');
var config = require('./config.js');
//putting our configuration details in twitter
var T = new Twitter(config);
// q is the required parameter and used to store search query. It will search for tweets containing #nodejs. Where count is the number of tweets, result_type:recent will return the most recent results and lang:en returs English results.
var params = {
q: '#nodejs',
count: 10,
result_type: 'recent',
lang: 'en'
}
// attach the search parameters into the get request to find tweets
// we make a get request to search/tweets and pass in our search .
T.get('search/tweets', params, function(err, data, response) {
// If there is no error, proceed
if(!err){
// Loop through the returned tweets
//our request will return an array of multiple tweets via the data.statuses object.
for(let i = 0; i < data.statuses.length; i++){
// Get the tweet Id from the returned data
let id = { id: data.statuses[i].id_str }
// Try to Favorite the selected Tweet
T.post('favorites/create', id, function(err, response){
// If the favorite fails, log the error message
if(err){
console.log(err[0].message);
}
// If the favorite is successful, log the url of the tweet
else{
let username = response.user.screen_name;
let tweetId = response.id_str;
console.log('Favorited: ', `https://twitter.com/${username}/status/${tweetId}`)
}
});
}
} else {
console.log(err);
}
})
Step 9: Now, your Twitter bot is ready which is app.js, to make it perform the task for which it is created you have to run the app.js file in your terminal.
node app.js
Isn't it easy! For any queries or feedback please feel free to post in comment section.
0 Comment(s)