Json.Net is a popular high-performance JSON framework for .NET
Features of Json.NET :
1. It provide JSON serializer for converting between .NET objects and JSON
2. It supports Binary JSON,XML to JSON, JSON to XML, LINQ to JSON conversions.
3. In ASP.NET Web API ,now JSON.NET is uses as a default serializer.
4. JSON.NET is a high performance JSON Framewrork faster than JavaScriptSerializer and DataContarctSerializer.
You can install JSON.NET using NuGet Package from 2 ways :
1. Package Manager Console
Click tools->NuGet Package Manager->Click Package Manager Console.
Then type the given code:
PM> Install-Package Newtonsoft.Json
2. Solution Explorer
Right click on Project in Solution Explorer ->Click Manage NuGet Packages->Select Browse->Search "Newtonsoft.Json" ->Click install.
Here, below is the example of serialize Json and Deserialize Json:
//Adding namespace:
using Newtonsoft.Json;
//create Class
class EmployeeInfo
{
public string Age { get; set; }
public string EmailId { get; set; }
public string FirstName { get; set; }
public string LastName { get; set; }
}
var employeeInfo = new EmployeeInfo
{
FirstName = "Mona",
LastName = "Jain",
Age = "25",
EmailId = "test@test.com"
};
// This will produce a JSON String
var serialized = JsonConvert.SerializeObject(employeeInfo);
// This will produce a copy of the instance you created earlier
var deserialized = JsonConvert.DeserializeObject<EmployeeInfo>(serialized);
OUTPUT :
serialize return :
{
"Age":"25",
"EmailId":"test@test.com",
"FirstName":"Mona",
"LastName":"Jain"
}
deserialized return :
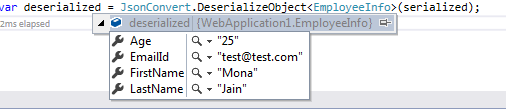
Hope this will help you. Thanks
0 Comment(s)