Remote Validation in ASP.NET MVC
Remote Validation is similar to an ajax call used for validating the user's input. The main purpose of remote validation is execution of back end query without performing a full server postback.
For example : In a form we want user to enter unique emailid then in this case remote validation is useful as uniqueness of emailid can be obtained without posting entire data of the form.
Create a MVC project with name "Student_Management_System". After creating the project create a model named "Student".
namespace Student_Management_System.Models
{
public class Student
{
[Key]
public int student_id { get; set; }
[Required]
[Display(Name = "First Name")]
public string Fname { get; set; }
[Required]
[Display(Name = "Last Name")]
public string Lname { get; set; }
[Required]
[Remote("ValidateStudentEmail", "Students", ErrorMessage = "Email already taken! Please enter Email again")]
public string Email { get; set; }
}
}
In above code it can be seen that remote attribute is used with Email. The first parameter in Remote attribute is action name, second parameter is the controller name and third parameter is the error message.
Implementation of "ValidateStudentEmail" action within "students" controller.
using System.Web.Mvc;
using Student_Management_System.DAL;
using Student_Management_System.Models;
namespace Student_Management_System.Controllers
{
public class StudentsController : Controller
{
private studentcontext db = new studentcontext();
public ActionResult ValidateStudentEmail(string email)
{
bool flag=true;
IQueryable<Student> students = db.students.AsQueryable<Student>().Where(x => x.Email.Contains(email));
if (students.ToList().Count() > 0)
flag = false;
return Json(flag, JsonRequestBehavior.AllowGet);
}
// GET: Students/Create
public ActionResult Create()
{
return View();
}
// POST: Students/Create
[HttpPost]
[ValidateAntiForgeryToken]
public ActionResult Create([Bind(Include = "student_id,Fname,Lname,Email")] Student student)
{
if (ModelState.IsValid)
{
db.students.Add(student);
db.SaveChanges();
return RedirectToAction("Index");
}
return View(student);
}
}
}
In above code studentcontext is a class which is actually interacting with tha database. The following is implementation of studentcontext class.
using System.Data.Entity;
using Student_Management_System.Models;
namespace Student_Management_System.DAL
{
public class studentcontext : DbContext
{
public studentcontext() : base("StudentConnString") { }
public DbSet<Student> students { get; set; }
}
}
Whenever the application runs with "students/create" following screen appears.
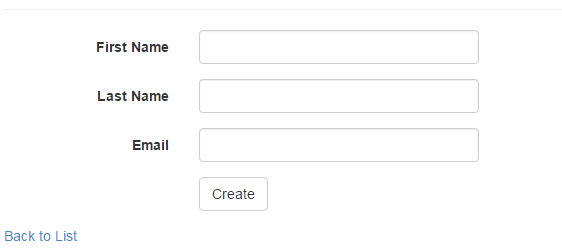
A student entry is created, when email of student is entered and cursor is clicked outside textbox of email then "ValidateStudentEmail" action run verifying the uniqueness of the entered email with that of email in database. IQueryable is used where using the “SQL” query filter is applied on the database therefore whenever a request is send by the client on the server side, on database a select query is fired and only required/filtered data is returned.
The list of student in database :
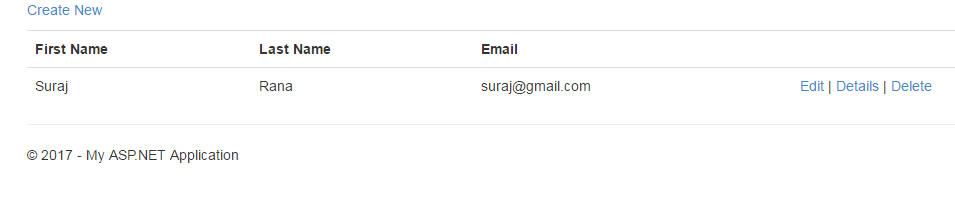
If an entry of Email is already found in database then an error message "Email already taken! Please enter Email again" occur,thereby validating email without posting the entire data of form.
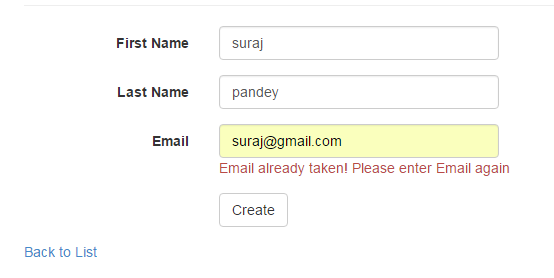
1 Comment(s)