STEP1:
To enable paging in your mvc project,First you need to install PagedList.mvc
Go To Tools=>Select Nuget Package Manager=>then Select Package Manager Console.
See the Screenshot for reference.

You will get a nuget window down the view.
Write the command :
Install-Package PagedList.Mvc
Click Enter.
Refer screenshot given below.

STEP2: In the view page, we need to change @model declaration.
Recently used model declaration is:
@model Ienumerable<MvcPracticeWebApplication.Models.StudentInfoModel>
But change it in PagedList format which will look like this:
@model PagedList.IPagedList<MvcPracticeWebApplication.Models.StudentInfoModel>
STEP3: Now for the Controller,you have to add Optional page Number and PageSize Parameters to the Paged ActionResult.
Controller:
[AllowAnonymous]
public ActionResult StudentDetails(int? page)
{
int pageSize = 3;
int pageNumber = (page ?? 1);
var response = apiStudentController.GetStudentDetails();
if (response.GetType() == typeof(System.Web.Http.Results.OkNegotiatedContentResult<List<StudentInfoModel>>))
{
List<StudentInfoModel> model = new List<StudentInfoModel>();
System.Web.Http.Results.OkNegotiatedContentResult<List<StudentInfoModel>> value = (System.Web.Http.Results.OkNegotiatedContentResult<List<StudentInfoModel>>)response;
model = value.Content;
return View(model.ToPagedList(pageNumber, pageSize));
}
else
{
return View("Error");
}
}
STEP4:
@Html.PagedListPager()
Now use above mentioned html helper into your view.
Refer the below view code to include the Paging.
@model PagedList.IPagedList<MvcPracticeWebApplication.Models.StudentInfoModel>
@using PagedList.Mvc;
@{
ViewBag.Title = "StudentDetails";
}
<h2>StudentDetails</h2>
<div>
<table class="table">
<tr>
<th>
@Html.DisplayName("Firstname")
</th>
<th>
@Html.DisplayName("Lastname")
</th>
<th>
@Html.DisplayName("DOB")
</th>
<th>
@Html.DisplayName("EmailId")
</th>
<th></th>
</tr>
@foreach (var item in Model)
{
<tr>
<td>
@Html.DisplayFor(modelItem => item.FirstName)
</td>
<td>
@Html.DisplayFor(modelItem => item.LastName)
</td>
<td>
@Html.DisplayFor(modelItem => item.DOB)
</td>
<td>
@Html.DisplayFor(modelItem => item.EmailId)
</td>
<td>
@Html.ActionLink("Edit", "Edit", new { Id = item.StudentId }) |
@Html.ActionLink("Details", "DisplayStudentDetails", new { Id = item.StudentId }) |
@Html.ActionLink("Delete", "Delete", new { id = item.StudentId }, new { onclick = "return confirm('Are you sure you want to delete this student?');" })
</td>
</tr>
}
</table>
<div class="pager">
Page @(Model.PageCount < Model.PageNumber ? 0 : Model.PageNumber) of @Model.PageCount
@Html.PagedListPager(Model, page => Url.Action("StudentDetails",new { page }))
</div>
<p>
@Html.ActionLink("Create New", "Index", "Student")
</p>
</div>
Debug the application and see the result:Refer screenshot
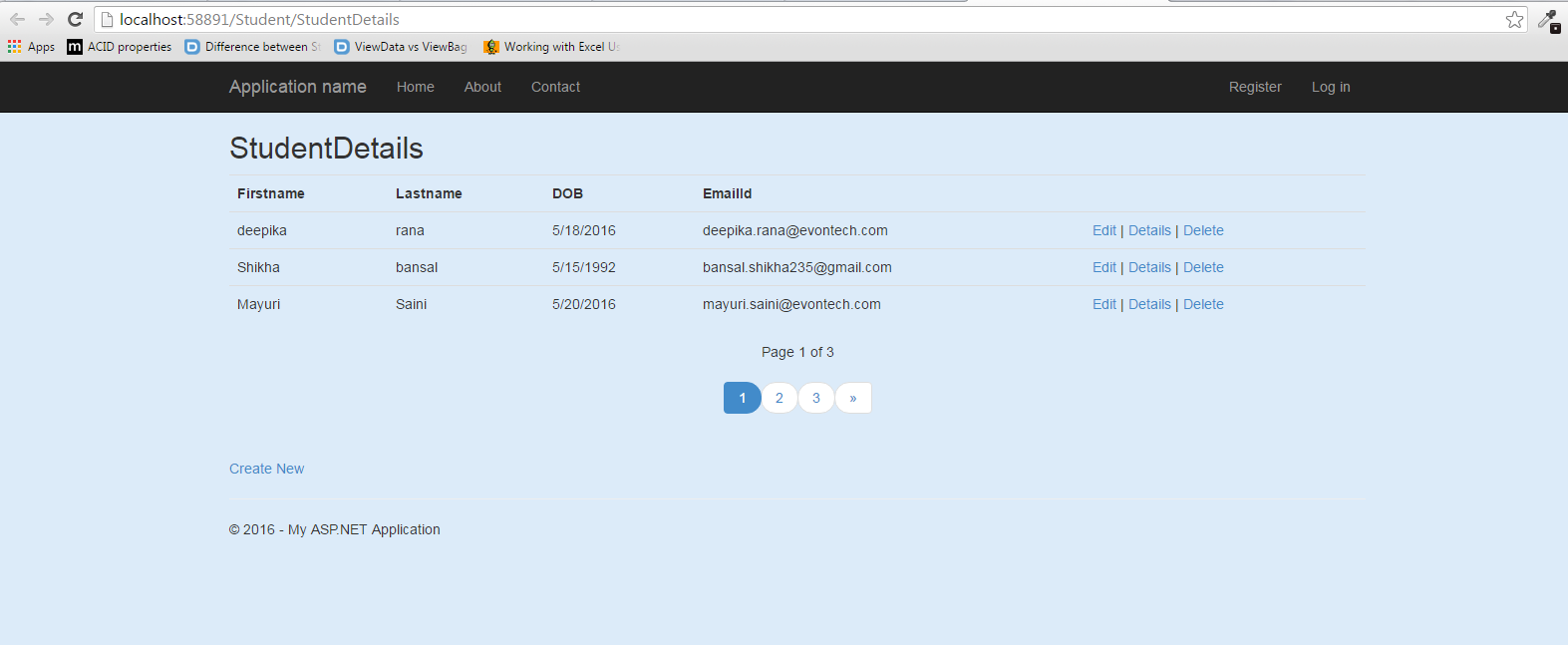
0 Comment(s)