Jquery traversing means moving through one HTML element to another based on their relations to other elements. In a family tree, starting from the selected (current) element, we can easily move up (ancestors), move down (descendants), and sideways (siblings). In other words, jquery traversing are used to find (or select) HTML elements based on their relation to other elements.
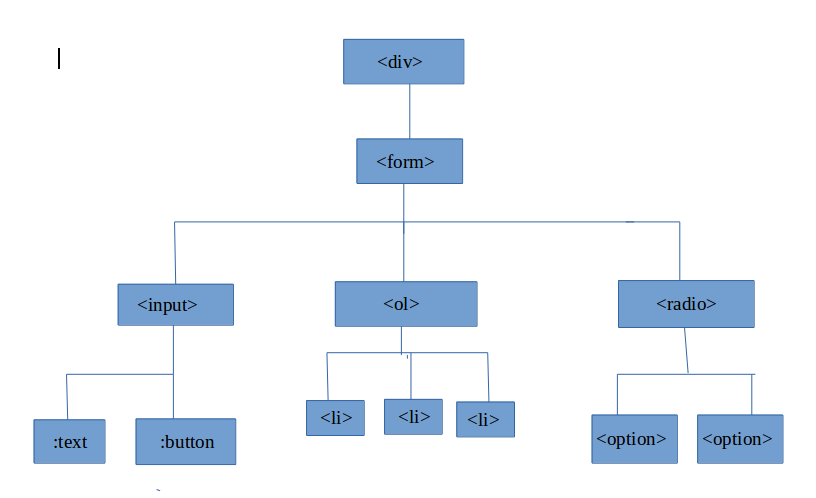
- The <div> element is the parent of <form>, and an ancestor of everything inside it.
- The <form> element is the parent of <input>, <ol>, and <radio>, and a child of <div>.
- <input>, <ol>, and <radio> are siblings (they share the same parent).
Finding elements by Index:
Every element has its own index, can be located directly by using eq(index) method. Every child element starts its index from zero.
Example: Program which adds the color to the second list item.
<html>
<head>
<title>Traversing (by index)</<title>
<script type = "text/javascript"
src ="http://ajax.googleapis.com/ajax/libs/jquery/2.1.3 /jquery.min.js"></script>
<script type = "text/javascript" language = "javascript">
$(document).ready(function() {
$("li").eq(2).addClass("selected");
});
</script>
<style>
.selected { font-weight:bold; }
</style>
</head>
<body>
<div>
<form>
<ul>
<li>List item 1</li>
<li>List item 2</li>
<li>List item 3</li>
<li>List item 4</li>
<li>List item 5</li>
</ul>
</form>
</div>
</body>
</html>
Output:
- List item 1
- List item 2
- List item 3
- List item 4
- List item 5
Locating descendant elements: To find all the descendant elements of a particular selected element find(selector) method is used.
DOM filter methods:
Method
|
Description
|
eq(index)
|
Reduce the set of matched elements to a single element.
|
filter(selector)
|
Removes all elements from the set of matched elements that do not match the specified selector(s).
|
filter(fn)
|
Removes all elements from the set of matched elements that do not match the specified function.
|
is(selector)
|
Checks the current selection against an expression and returns true, if at least one element of the selection fits the given selector.
|
map(callback)
|
Translate a set of elements in the jQuery object into another set of values in a jQuery array (which may, or may not contain elements).
|
not(selector)
|
Removes elements matching the specified selector from the set of matched elements.
|
slice(start,[end])
|
Selects a subset of the matched elements.
|
DOM traversing methods:
Method
|
Description
|
parent([selector])
|
Returns the direct parent element of the selected element
|
parents([selector])
|
Returns all ancestor elements of the selected element, all the way up to the document's root element (<html>).
|
offsetParent()
|
Returns a jQuery collection with the positioned parent of the first matched element
|
find([selector])
|
Returns descendant elements of the selected element, all the way down to the last descendant.
|
children([selector])
|
Returns all direct children of the selected element.
|
add([selector])
|
Adds more elements, matched by the given selector, to the set of matched elements.
|
andSelf()
|
Add the previous selection to the current selection.
|
closest(selector)
|
Get a set of elements containing the closest parent element that matches the specified selector, the starting element included.
|
contents()
|
Find all the child nodes inside the matched elements (including text nodes), or the content document, if the element is an iframe.
|
next([selector])
|
Returns the next sibling element of the selected element.
|
end()
|
Revert the most recent 'destructive' operation, changing the set of matched elements to its previous state.
|
nextAll([selector])
|
Returns the next sibling element of the selected element.
|
siblings()
|
Returns all sibling elements of the selected element.
|
prev([selector])
|
Get a set of elements containing the unique previous siblings of each of the matched set of elements.
|
prevAll([selector])
|
Find all sibling elements in front of the current element.
|
0 Comment(s)