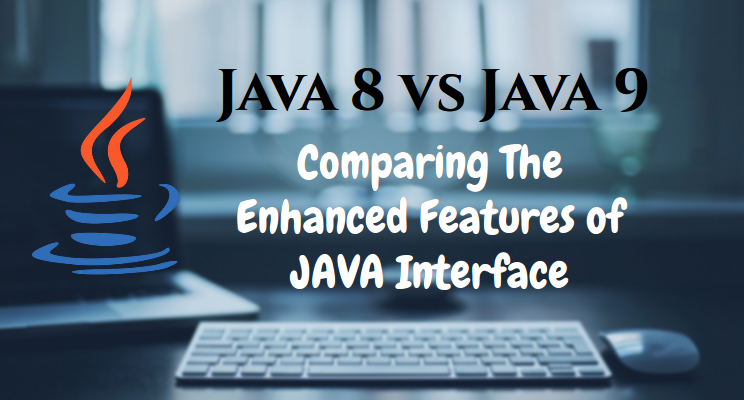
Java 8 came with the enhanced functionality to the Interfaces. Prior to Java 8, interfaces were contracted only with the abstract methods and subclass was obliged to implement the contract. Child class has to implement the abstract functions in order to fulfill the contract. Java 8 onwards, Interface got more powerful with the static and default methods.
interface Alpha{
default void bar(){
System.out.println("Default bar of A!!");
}
}
class Clazz implements Alpha{
// Java8 compiler is ok, We can create Object of Clazz
//Because of default method provided, we do not have to override the bar method
}
In this discussion, we will get through some Java 8 Interface features/enhancements and also the strength given to the Interfaces with Java 9.
With Java 8, We have a new feature with the default implementation of methods in interfaces. Now we can provide the default implementation for methods which we do not want to be overridden by every class, default implementation can be provided by writing the default keyword for the method. Jave compiler will not force to override that method declared as default. We can override that method in any of the implementation class but It would not be necessary to provide an implementation for the default method.
What about the diamond problem?
As Default Method and Multiple Inheritance comes up with the Ambiguity Problems. Java does not provide Multiple Inheritance to avoid the diamond problem. Here with the default implementation, we can be caught with the same issue. Any class implementing 2 interfaces and have the same method as default, we are caught in the Ambiguity issue.
Diamond Problem:-
interface Alpha{
default void bar(){
System.out.println("Default bar of A!!");
}
}
interface Beta{
default void bar(){
System.out.println("Default bar of B!!");
}
}
class Clazz implements Alpha,Beta{
// Code fails to compile with the following result:
// error: class Clazz inherits unrelated defaults for bar() from types Alpha and Beta
}
To fix that, in Clazz.class, we have to override the method bar to resolve the conflicts
class Clazz implements Alpha,Beta{
public void bar(){
System.out.println("Overridden bar!!");
}
}
Also we can get it done through another way. Suppose out of the 2 default implementation of bar in the interfaces Alpha and Beta, We want to use the default one of Alpha then we can have the following workaround also:-
class Clazz implements Alpha,Beta{
public void bar(){
Alpha.super.bar();
}
}
In Java 9, Interface is getting more enhanced and now more strengthen, Java 9 interface can declare private methods, keeping intact the feature of the default method introduced by Java 8. Now we can define private methods as well in the interfaces. Let us walk through the importance and need of private methods in Interfaces.
With the introduction of private method from Java9, We have the following advantages:-
No need to write Duplicate Code i.e. Code Reusability.
We can expose only our intended methods to clients.
In the below use case, ReportInterface is responsible for the generation of different format of reports i.e excel, pdf, csv. Check out the implementation taking the advantage of private method of interfaces.
package com.evon.interfaces;
public interface ReportInterface {
default void getFormattedReport(String reportType){
if(reportType.equals("Excel")){
generateExcelReport();
}else if(reportType.equals("Pdf")){
generatePDFReport();
}else if(reportType.equals("Csv")){
generateCSVReport();
}
}
private void generateExcelReport(){
//generateExcelReport code goes here
}
private void generatePDFReport(){
//generatePDFReport code goes here
}
private void generateCSVReport(){
//generateCSVReport code goes here
}
}
2 Comment(s)