"Custom Error pages in Asp.Net MVC"
In this article we will learn how to make custom error pages in Asp.Net MVC.
Getting Started:
By default if we get any exception while running our code, we are redirected to a Yellow Screen Of Death, containing the details of the error.
Example:
public class HomeController : Controller
{
// ...
public ActionResult About()
{
throw new Exception("This is not good. Something bad happened.");
}
}
By default this code will give us the following Yellow Screen Of Death.
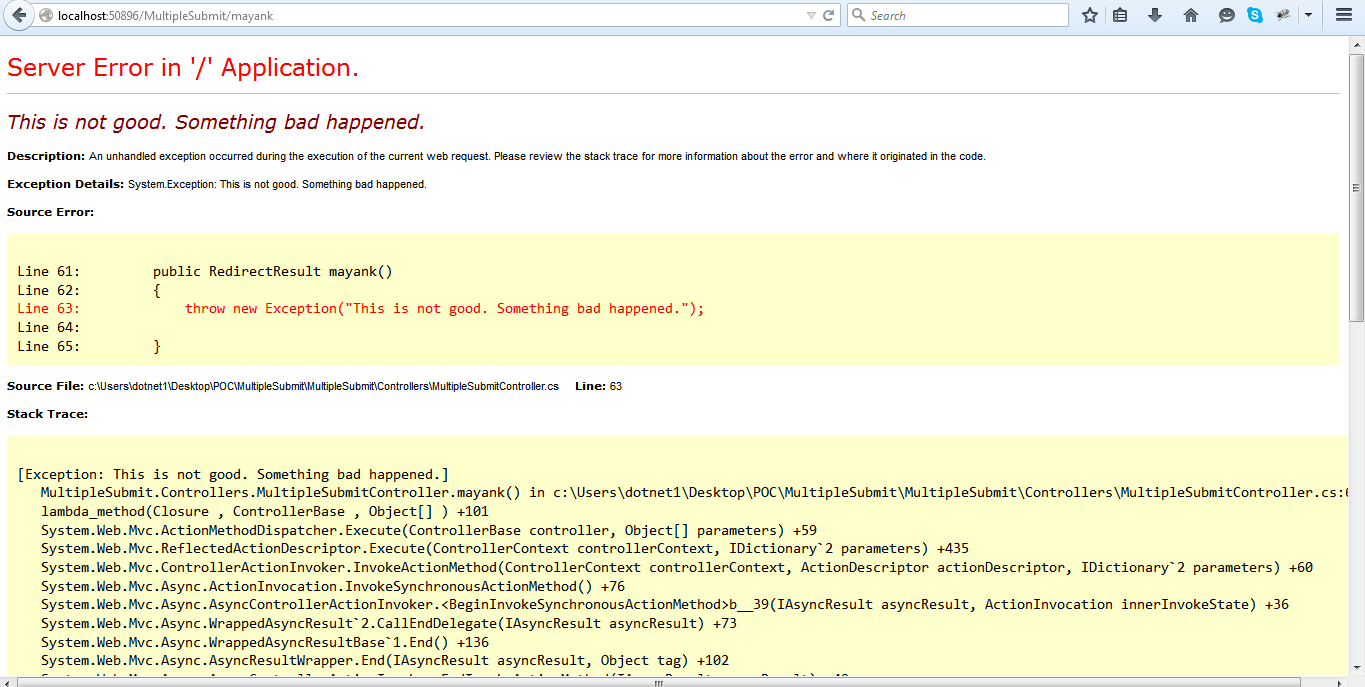
But if we wan't to display a Custom Error page then:
Step 1: Write the following code in your web.config file:
<system.web>
<customErrors mode="On" defaultRedirect=~/BadError.htm"/>
</system.web>
This will set your custom error mode 'ON' and will always redirect you to "~/Views/Shared/Error.cshtml " on every exception.
Step 2: Now we can write our own content at "~/Views/Shared/Error.cshtml". By default the
'Error.cshtml' will look like:
@model System.Web.Mvc.HandleErrorInfo
@{
ViewBag.Title = "Error";
}
<h1 class="text-danger">Error.</h1>
<h2 class="text-danger">An error occurred while processing your request.</h2>
We can write the description of the exception as follows:
@model System.Web.Mvc.HandleErrorInfo
@{
ViewBag.Title = "Error";
}
<h2>
Sorry, an error occurred while processing your request.
</h2>
<p>Controller = @Model.ControllerName</p>
<p>Action = @Model.ActionName</p>
<p>Message = @Model.Exception.Message</p>
<p>StackTrace :</p>
<pre>@Model.Exception.StackTrace</pre>
Now incase of exceptions it will be redirected to the "~/Views/Shared/Error.cshtml", but incase of errors such as "404 page not found", etc. it will be redirected to the path defaultRedirect=~/BadError.htm" taken from <customErrors mode="On" defaultRedirect = ~/BadError.htm"/>
Note:-> If we wan't to redirect to different error pages for errors with different status code then write the following code in your Web.Config file:
<system.web>
<customErrors mode="On" defaultRedirect=~/BadError.htm">
<error redirect="~/MultipleSubmit/FileUpload" statusCode="404" />
//...
</customErrors>
</system.web>
This will redirect to "~/MultipleSubmit/FileUpload" for Error with status code 404.Similarly we can declare redirect actions for other status codes also.
But if we don't want to redirect to "~/Views/Shared/Error.cshtml" and wan't to go some other view then:
Step 1: Write the following code in your web.config file:
<system.web>
<customErrors mode="On"/>
</system.web>
Step 2: Goto FilterConfig.cs in App_Start folder, you will find the following code:
using System.Web;
using System.Web.Mvc;
namespace MultipleSubmit
{
public class FilterConfig
{
public static void RegisterGlobalFilters(GlobalFilterCollection filters)
{
filters.Add(new HandleErrorAttribute());
}
}
}
Edit the above code as :
using System.Web;
using System.Web.Mvc;
namespace MultipleSubmit
{
public class FilterConfig
{
public static void RegisterGlobalFilters(GlobalFilterCollection filters)
{
filters.Add(new HandleErrorAttribute{ View = "Name of the view" });
}
}
}
Now whenever the exception will occur, it will be redirected to the View mentioned in the above code and not to the "~/Views/Shared/Error.cshtml".
Hope it helps....Happy Coding!
0 Comment(s)