This article demonstrate different methods to create an active form with different input fields in Yii 2. Yii 2 provides yii\widgets\ActiveForm
class which is the best way to create forms based upon models. Another helper class yii\helpers\Html
used to generate different html input fields. The article mainly defines the methods to generate different input fields in Yii 2.
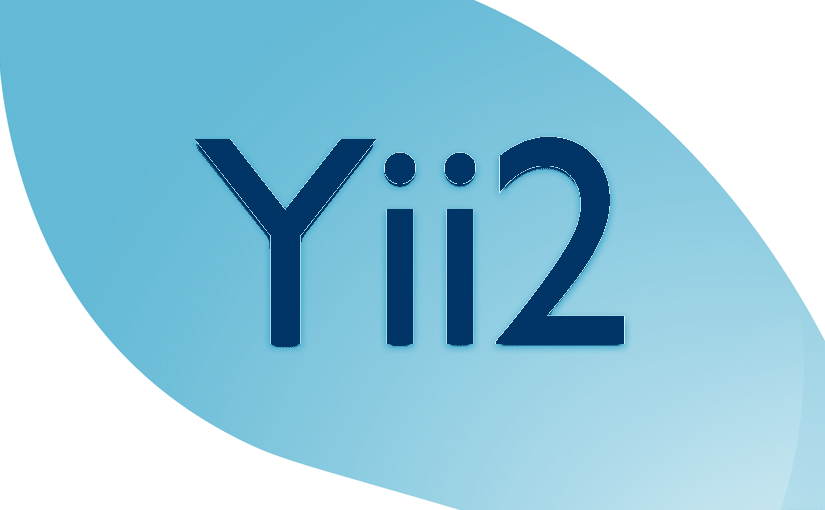
Note : The FieldName inside field() function specified the model fields (field names of database table or the variables defined in model).
The first step is to include both classes :
use yii\helpers\Html;
use yii\widgets\ActiveForm;
Create Form
There are two methods to open and close a form using yii\widgets\ActiveForm
class.
/*default initialization*/
<? $form = ActiveForm::begin(); ?>
OR
/*initialization with different parameters*/
<?php $form = ActiveForm::begin([
'id' => 'form_id',
'options' => [
'class' => 'form-horizontal',
'enctype' => 'multipart/form-data',
],
]); ?>
<?php ActiveForm::end(); ?>
Creating Input Fields
The methods to generate different input fields for example textbox, textarea, radio button etc is given below :
1. Text Input Field
- The simplest way to create a text input field is :
<?= $form->field($model,'FieldName') ?>
OR
<?= $form->field($model,'FieldName')->input('text') ?>
OR
<?= $form->field($model, 'FieldName')->textInput() ?>
- Create text input with label and hint text :
<?= $form->field($model,'FieldName')->textInput()->hint('Hint Text')->label('LabelName') ?>
2. Password Input Field
- The simplest way to create a password input field is :
<?= $form->field($model, 'FieldName')->passwordInput() ?>
OR
<?= $form->field($model, 'FieldName')->input('password') ?>
- Another way to create text input with label and hint text :
<?= $form->field($model, 'FieldName')->passwordInput()->hint('Password should not be less than 6 characters')->label('Password') ?>
3. Creating TextArea
<?= $form->field($model, 'FieldName')->textarea() ?>
<?= $form->field($model, 'FieldName')->textarea()->label('Label Name') ?>
<?= $form->field($model, 'FieldName')->textarea(array('rows'=>2,'cols'=>5)) ?>
4. HTML 5 Email Field
<?= $form->field($model, 'FieldName')->input('email') ?>
5. File Input Field
<?= $form->field($model, 'FieldName')->fileInput() ?>
- For multiple file upload :
<?= $form->field($model, 'FieldName[]')->fileInput(['multiple'=>'multiple']) ?>
6. Radio Button
<?= $form->field($model, 'FieldName')->radio() ?>
<?= $form->field($model, 'FieldName')->radio(array('label'=>''))->label('Label Name') ?>
<?= $form->field($model, 'FieldName')->radio(array(
'label'=>'',
'labelOptions'=>array('style'=>'padding : 3px;')))
->label('Label Name') ?>
Create radio group
<?= $form->field($model, 'FieldName')->radioList(array('1'=>'Value1', 2=>'value2')) ?>
7. Checkbox
<?= $form->field($model, 'FieldName')->checkbox() ?>
<?= $form->field($model, 'FieldName')->checkbox(array(
'label'=>'',
'labelOptions'=>array('style'=>'padding : 3px;'),
'disabled'=>true
))
->label('Label Name') ?>
Creating Checkbox list
<?= $form->field($model, 'FieldName[]')->checkboxList(['1' => 'Value1', '2' => 'Value2', '3' => 'Value3']) ?>
8. Dropdown(Select) list
<?= $form->field($model, 'FieldName')->dropDownList(['1' => 'Value1', '2' => 'Value2', '3' => 'Value3']) ?>
<?= $form->field($model, 'FieldName')->dropDownList($listData, ['prompt'=>'--Select--']) ?>
Creating Submit Button
Submit Button can be generated using submitButton() method of Html helper class.
<?= Html::submitButton('Submit', ['class'=> 'btn btn-primary']) ?>
0 Comment(s)