Draw a circle - Create a circle on a google map
Following javascript code will create circles on the map and it will represent populations in respected town. For that first you need to create an object containing latitude and longitude and population for each city:
var citymap = {
chicago: {
center: {lat: 41.878, lng: -87.629},
population: 2714856
},
newyork: {
center: {lat: 40.714, lng: -74.005},
population: 8405837
},
losangeles: {
center: {lat: 34.052, lng: -118.243},
population: 3857799
},
vancouver: {
center: {lat: 49.25, lng: -123.1},
population: 603502
}
};
Initialize the Map and draw circle:
function initMap() {
// Create the map.
var map = new google.maps.Map(document.getElementById('map'), {
zoom: 4,
center: {lat: 37.090, lng: -95.712},
mapTypeId: google.maps.MapTypeId.TERRAIN
});
// Construct the circle for each value in citymap.
// Note: We scale the area of the circle based on the population.
for (var city in citymap) {
// Add the circle for this city to the map.
var cityCircle = new google.maps.Circle({
strokeColor: '#FF0000',
strokeOpacity: 0.8,
strokeWeight: 2,
fillColor: '#FF0000',
fillOpacity: 0.35,
map: map,
center: citymap[city].center,
radius: Math.sqrt(citymap[city].population) * 100
});
}
}
Code with Javascript and HTML both (complete code). Copy and paste it on your page and run it.
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="initial-scale=1.0, user-scalable=no">
<meta charset="utf-8">
<title>Circles</title>
<style>
html, body {
height: 100%;
margin: 0;
padding: 0;
}
#map {
height: 100%;
}
</style>
</head>
<body>
<div id="map"></div>
<script>
var citymap = {
chicago: {
center: {lat: 41.878, lng: -87.629},
population: 2714856
},
newyork: {
center: {lat: 40.714, lng: -74.005},
population: 8405837
},
losangeles: {
center: {lat: 34.052, lng: -118.243},
population: 3857799
},
vancouver: {
center: {lat: 49.25, lng: -123.1},
population: 603502
}
};
function initMap() {
// Create the map.
var map = new google.maps.Map(document.getElementById('map'), {
zoom: 4,
center: {lat: 37.090, lng: -95.712},
mapTypeId: google.maps.MapTypeId.TERRAIN
});
// Construct the circle for each value in citymap.
// Note: We scale the area of the circle based on the population.
for (var city in citymap) {
// Add the circle for this city to the map.
var cityCircle = new google.maps.Circle({
strokeColor: '#FF0000',
strokeOpacity: 0.8,
strokeWeight: 2,
fillColor: '#FF0000',
fillOpacity: 0.35,
map: map,
center: citymap[city].center,
radius: Math.sqrt(citymap[city].population) * 100
});
}
}
</script>
<script async defer
src="https://maps.googleapis.com/maps/api/js?key=YOUR_API_KEY&callback=initMap">
</script>
</body>
</html>
Create API KEY and replace the key "YOUR_API_KEY" with your respective key created by google project..
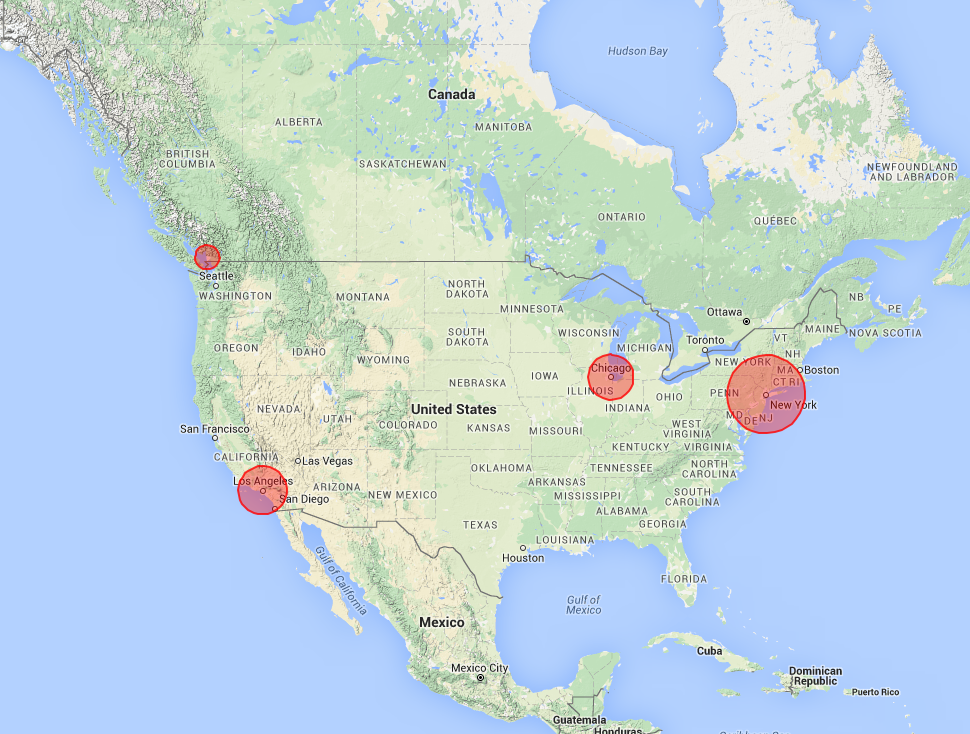
Thanks for reading the blog.
0 Comment(s)