Custom Filters in AngularJS
The data can be modified through filters in Angularjs. AngularJS not only provides built in filters but also provides with the capability of creating custom filters. Custom filters can be created through ".filter" method.
Syntax of creating custom filter
var app = angular.module('Applicationname',[])
app.filter('', function () {
return function () {
return;
};
});
Whenever angular calls the filter the returned function in above syntax gets called i.e there is two-way binding for filters. Whenever a change is made by the user the filter will run and will provide the updates as necessary.
Example to create the uppercase custom filter:-
<html>
<head>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.4.8/angular.min.js"></script>
</head>
<body ng-app="mainapp" ng-controller="maincontroller">
<p>The Url of the page is:</p>
{{localurl | Uppercase }}
<script>
var app = angular.module("mainapp",[]);
app.filter('Uppercase', function () {
return function (item) {
return item.toUpperCase();
};
});
app.controller("maincontroller",function($scope,$location){
$scope.localurl = $location.absUrl();
})
</script>
</body
</html>
Explanation of above program :-
In above program within script section a custom filter named "Uppercase" is defined which takes an argument named item and convert it into uppercase and returns the converted data. Now this defined filter can be used with any data in order to make the data into uppercase. In above example an inbuilt service "$location" is used which when uses method "absUrl()" returns information related to current web page location. This information is then converted into uppercase through created filter "Uppercase"
Output :-
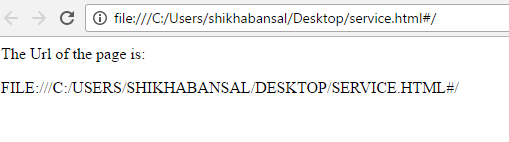
Creation of custom filter for ng-repeat
<html>
<head>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.4.8/angular.min.js"></script>
</head>
<body ng-app="mainapp" ng-controller="maincontroller">
<input type="text" ng-model="initialletter" placeholder="Enter initialletter to filter">
<ul>
<li ng-repeat="state in states|startsWithInitialLetter:initialletter">
<p>{{state}}</p>
</li>
</ul>
<script>
var app = angular.module("mainapp",[]);
app.filter('startsWithInitialLetter', function () {
return function (items, initialletter) {
var filtereddata = [];
var matchingLetter = new RegExp(initialletter, 'i');
for (var i = 0; i < items.length; i++) {
var item = items[i];
if (matchingLetter.test(item.name.substring(0, 1))) {
filtereddata.push(item);
}
}
return filtereddata;
};
});
app.controller("maincontroller",function($scope){
$scope.states = [{
name : 'Dehradun'
},{
name : 'Goa'
},{
name : 'Arunachal Pradesh'
},{
name : 'Gujarat'
}
]
})
</script>
</body
</html>
Explanation of above program :-
In above program a custom filter named "startsWithInitialLetter" is defined which takes items,initialletter and filter these items according to initial letter i.e only those words with starting letter in input box will be displayed. An array of states is defined within controller then this array is looped through "ng-repeat" but when looped are passed through custom filter "startsWithInitialLetter" along with initial letter on basis of which items in array will filter out. When there is no letter in input box all items in array are displayed.
Output 1: When no letter is entered in input box
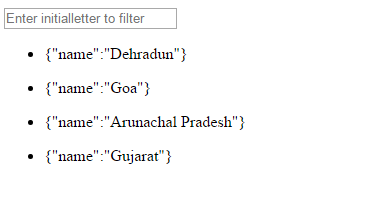
Output 2: When a letter is entered in input box to filter out items
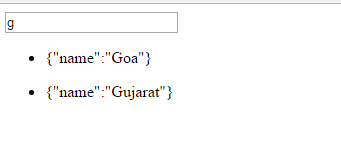
0 Comment(s)