Grouping operators generates a categories or groups of an item on the basis of given key. These type of operators implements IEnumerable collection of type IGrouping<Key, SourceItem> where Key is a key value, on the basis of which grouping is being done and SourceItem is the collection of items.
In linq, We have a two types of grouping operators:
1. GroupBy
2. ToLookup
1. GroupBy:- It takes a collection of items and returns a group of items from the given collection based on some key value.
Query syntax :-
Lets take an example we have a collection of an employees of a different departments. We need to make a groups of an employee of a same department.
Code:-
IList<Employee> empList = new List<Employee>() {
new Employee() { EmpID = Guid.NewGuid().ToString(), EmpName = "John", Dept = "Electronic Department" } ,
new Employee() { EmpID = Guid.NewGuid().ToString(), EmpName = "Steve", Dept = "Computer Department" } ,
new Employee() { EmpID = Guid.NewGuid().ToString(), EmpName = "Bill", Dept = "Geographical Department" } ,
new Employee() { EmpID = Guid.NewGuid().ToString(), EmpName = "Ram" , Dept = "Computer Department" } ,
new Employee() { EmpID = Guid.NewGuid().ToString(), EmpName = "Abram" , Dept = "Geographical Department" }
};
var result = from e in empList
group e by e.Dept;
foreach (var deptGroup in result)
{
Console.WriteLine("Department Group: {0}", deptGroup .Key);
foreach(Employee e in deptGroup )
{
Console.WriteLine("Employee Name: {0}", e.EmpName);
}
}
Output:-
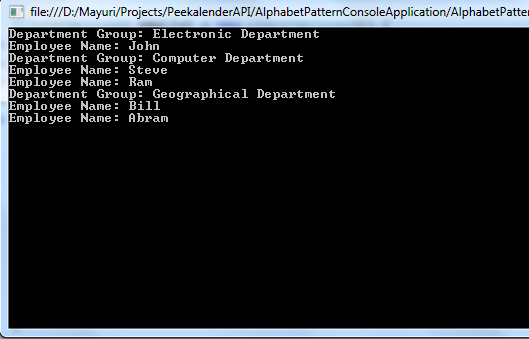
Method syntax for above code:-
IList<Employee> empList = new List<Employee>() {
new Employee() { EmpID = Guid.NewGuid().ToString(), EmpName = "John", Dept = "Electronic Department" } ,
new Employee() { EmpID = Guid.NewGuid().ToString(), EmpName = "Steve", Dept = "Computer Department" } ,
new Employee() { EmpID = Guid.NewGuid().ToString(), EmpName = "Bill", Dept = "Geographical Department" } ,
new Employee() { EmpID = Guid.NewGuid().ToString(), EmpName = "Ram" , Dept = "Computer Department" } ,
new Employee() { EmpID = Guid.NewGuid().ToString(), EmpName = "Abram" , Dept = "Geographical Department" }
};
var result = empList .GroupBy(e => e.Dept);
foreach (var deptGroup in result)
{
Console.WriteLine("Department Group: {0}", deptGroup.Key);
foreach (Employee e in deptGroup)
{
Console.WriteLine("Employee Name: {0}", e.EmpName);
}
}
2. ToLookup:-
ToLookup operator is similar to a GroupBy operator but their execution way is different because GroupBy operator executes delay while ToLookup operator executes instantly. ToLookup also not supports a query syntax, it supports only a method syntax.
Example:-
Let's take an example, we have a collection of employees of a different departments. We need to make a group of an employee of a same department.
Code:-
IList<Employee> empList = new List<Employee>() {
new Employee() { EmpID = Guid.NewGuid().ToString(), EmpName = "John", Dept = "Electronic Department" } ,
new Employee() { EmpID = Guid.NewGuid().ToString(), EmpName = "Steve", Dept = "Computer Department" } ,
new Employee() { EmpID = Guid.NewGuid().ToString(), EmpName = "Bill", Dept = "Geographical Department" } ,
new Employee() { EmpID = Guid.NewGuid().ToString(), EmpName = "Ram" , Dept = "Computer Department" } ,
new Employee() { EmpID = Guid.NewGuid().ToString(), EmpName = "Abram" , Dept = "Geographical Department" }
};
var result = empList .ToLookup(e=>e.Dept);
foreach (var deptGroup in result)
{
Console.WriteLine("Department Group: {0}", deptGroup.Key);
foreach (Employee e in deptGroup)
{
Console.WriteLine("Employee Name: {0}", e.EmpName);
}
}
Output:-
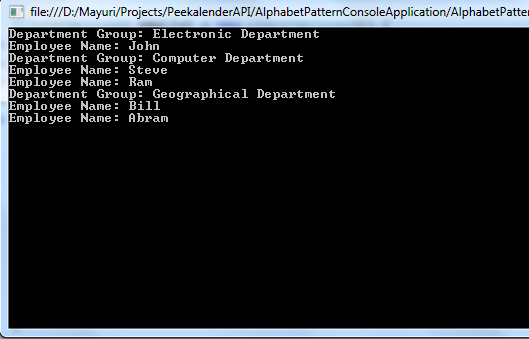
0 Comment(s)