A dropdownlist is a list of items from which user can select one or multiple values at the same time.
Every dropdownlist contain two items one is a value and another is text. Here in the given example,there is a list of student names in which Student ID is the value and student name is the text.
To get the text/value from dropdownlist, Let's take an example:
First, open your database and create a table named tblstudentdetails in which we add two columns studentId and FirstName.
StudentId is the primary key and auto incremented in this table.
Enter Dummy Data in this table to bind dropdownlist with text and value.
See the image for reference:
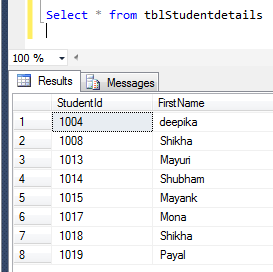
Now create a stored procedure which will select StudentId and FirstName from the table.
Stored Procedure:
Create Proc sp_GetStudentNameDetails
As
BEGIN
Select StudentId, FirstName from tblStudentdetails
END
In your mvc application, First add a class with the name StudentModel.
StudentModel:
using System;
using System.ComponentModel.DataAnnotations;
using System.Web.Mvc;
namespace MvcPracticeWebApplication.Models
{
public class StudentModel
{
[Required]
public int StudentId { get; set; }
[Required]
public string FirstName { get; set; }
}
}
Now add a class in which we create a method to get the studentdetails. I have created a StudentRepository class.
StudentRepository:
public List<StudentModel> GetStudentNameDetail()
{
List<StudentModel> GetStream = new List<StudentModel>();
Result result = new Result();
SqlDataReader dr = null;
using (SqlConnection sqlconnection = new SqlConnection(ConnectionString))
{
try
{
List<SqlParameter> sqlparam = new List<SqlParameter>();
if (sqlconnection.State == ConnectionState.Closed)
sqlconnection.Open();
dr = BaseRepository.ExecuteReader(sqlconnection, CommandType.StoredProcedure, "sp_GetStudentNameDetails", sqlparam.ToArray());
if (dr.HasRows)
{
while (dr.Read())
{
StudentModel model = new StudentModel();
model.StudentId = Convert.ToInt32(dr["StudentId"]);
model.FirstName = dr["FirstName"].ToString();
GetStream.Add(model);
}
}
}
catch (Exception ex)
{
throw ex;
}
return GetStream;
}
}
Now add a WebApi controller in your application. I have created a StudentApiController.
StudentAPIController:
public IHttpActionResult GetStudentNameDetail()
{
try
{
var info = iStudentRepository.GetStudentNameDetail();
return Ok(info);
}
catch (Exception ex)
{
return InternalServerError(ex);
}
}
Now add a controller in your application to bind your dropdown with the fetched Student details. Create a method to get the values in a list. Create an ActionResult method to call that list.This ActionResult method will return the view.
StudentController:
public List<SelectListItem> GetStudentNameDetails()
{
var Studentlist = new List<SelectListItem>();
var responseGetStudents = apiStudentController.GetStudentNameDetail ();
if (responseGetStudents.GetType() == typeof(System.Web.Http.Results.OkNegotiatedContentResult<List<StudentModel>>))
{
System.Web.Http.Results.OkNegotiatedContentResult<List<StudentModel>> Students = (System.Web.Http.Results.OkNegotiatedContentResult<List<StudentModel>>)responseGetStudents;
foreach (var item in Students.Content)
{
Studentlist.Add(new SelectListItem
{
Text = item.Student,
Value = Convert.ToString(item.StudentId)
});
}
}
return Studentlist;
}
[HttpGet]
Public ActionResult Index()
{
Viewbag.Student = GetStudentNameDetails();
return view();
}
Now Right click on the ActionMethod and add a stronglybind view.
Index View :
@model MvcPracticeWebApplication.Models.StudentModel
@{
ViewBag.Title = "Index";
}
@using (Html.BeginForm())
{
<div >
<ul >
<li>
<div >
@Html.Label("Student Name*")
<div >
@Html.DropDownList("ddlStudent", new System.Web.Mvc.SelectList(ViewBag.student, "Value", "Text"), "Please select a Student", new { @class = "required" })
</div>
</div>
<div>Selected Student:<b><label id="lbltxt"></label></b></div>
<div>Selected StudentId:<b><label id="lblval"></label></b></div>
<br />
</li>
</ul>
</div>
}
@section Scripts {
@Scripts.Render("~/bundles/jqueryval")
<script type="text/javascript" language="javascript">
$('#ddlStudent').change(function () {
$("#lbltxt").text($("#ddlStudent option:selected").text());
$("#lblval").text($("#ddlStudent").val());
return false;
});
$("#ddlform").validate({
rules: {
ddlStudent: "required",
},
messages: {
ddlStudent: "Please select Student Name",
},
});
</script>
}
Debug the application and see the view, you'll get the dropdown filled with studentnames.
See the image for reference:
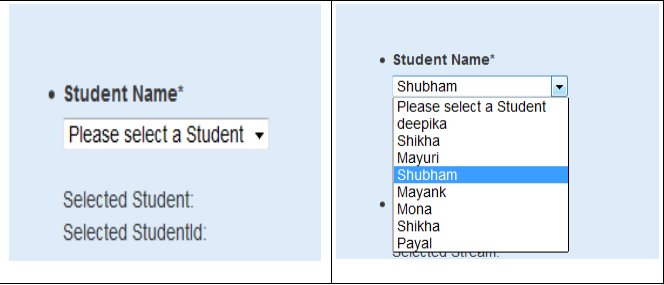
Now Select the value from the dropdown, you will get the respective text and value.
See the image for reference:
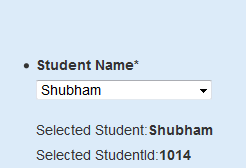
0 Comment(s)