Filters in MVC are used to perform some action or task before the program gets executed.
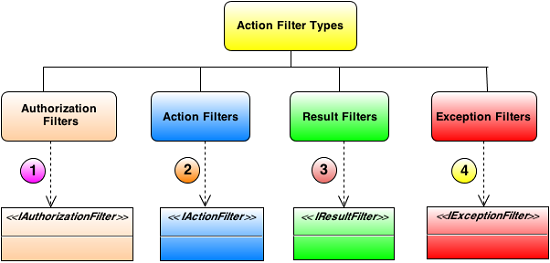
Types of filters in MVC
-
Authentication filters (New in ASP.NET MVC5)
-
Authorization filters
-
Action filters
-
Result filters
-
Exception filters
Authentication Filter : This filter is used to create custom authentication in your application.
public interface IAuthenticationFilter
{
void OnAuthentication(AuthenticationContext filterContext);
void OnAuthenticationChallenge(AuthenticationChallengeContext filterContext);
}
You can create custom authentication filter by inheriting IAuthenticationFilter interface
public class CustomAuthenticationAttribute : ActionFilterAttribute, IAuthenticationFilter
{
public void OnAuthentication(AuthenticationContext filterContext)
{
//Logic for authenticating a user
}
//Runs after the OnAuthentication method
public void OnAuthenticationChallenge(AuthenticationChallengeContext filterContext)
{
//TODO: Additional tasks on the request
}
}
Authorization Filter : This is used to create custom authorization/role checking in your MVC application.
public class AuthorizeAttribute : FilterAttribute, IAuthorizationFilter
{
protected virtual bool AuthorizeCore(HttpContextBase httpContext);
protected virtual void HandleUnauthorizedRequest(AuthorizationContext filterContext);
public virtual void OnAuthorization(AuthorizationContext filterContext);
protected virtual HttpValidationStatus OnCacheAuthorization(HttpContextBase httpContext);
}
Action Filter : Before and after the execution of the action the action filter is used. The IActionFilter is used to implement the action filter which uses two methods one is OnActionExecuting and another is OnActionExecuted. OnActionExecuting runs before the Action and gives an chance to cancel the Action call.
public interface IActionFilter
{
void OnActionExecuting(ActionExecutingContext filterContext);
void OnActionExecuted(ActionExecutedContext filterContext);
}
Result Filter : Before or after the action execution of result the Result filters will be executed. It can be ViewResult, PartialViewResult, RedirectToRouteResult, RedirectResult, ContentResult, JsonResult, FileResult and EmptyResult it is derived from the ActionResult class.
public interface IResultFilter
{
void OnResultExecuted(ResultExecutedContext filterContext);
void OnResultExecuting(ResultExecutingContext filterContext);
}
Exception Filter : Exception filter are executed when an exception occurs with action or with action result.
public interface IExceptionFilter
{
void OnException(ExceptionContext filterContext);
}
0 Comment(s)