Google Map provides a facility to display maps on web page of web application. We used Google map API to show and customize the gmap on our web page.
The GMap API is a kind of JavaScript library. Here we will learn how to implementation and customization the the gmap.
In order to display the gmap, there are two things required i.e. HTML page and JavaScript to invoke Google API
HTML page : which is contained the Google Map <div> tag with unique id
<!DOCTYPEhtml>
<html>
<head>
<title>Google Map</title>
</head>
<body>
<h1>Google Map Integration</h1>
<div id="gmap_wrapper" style="height:400px; width:650px;">
<div id="gmap_canvas" ></div>
</div>
</body>
</html>
Here we taken a <div> with ID 'gmap_canvas' in which we display the Google markers
JavaScript Code : which is used to invoke Google API and customize function to override the API methods
<script type="text/javascript">
jQuery(function($) {
// Asynchronously load the googlemap API on webpage
var js = document.createElement('script');
js.src = "//maps.googleapis.com/maps/api/js?sensor=false&callback=initialize";
document.body.appendChild(js);
});
function initialize() {
var gmap;
var bounds = new google.maps.LatLngBounds();
var mapOptions = {
mapTypeId: 'roadmap'
};
// Display a google map on the web page
gmap = new google.maps.Map(document.getElementById("gmap_canvas"), mapOptions);
gmap.setTilt(45);
// Multiple location Markers
var myLocations = [
['New delhi, INDIA', 28.610746852715387,77.21122741699219, 'New delhi, INDIA'],
['UPSC New delhi, INDIA', 28.60622574490014,77.22908020019531, 'Union Service Public New delhi, INDIA'],
['National Zoological Park, New Delhi India', 28.6730542, 77.3238588, 'National Zoological Park, Mathura Road, New Delhi, Delhi 110003, India'],
['India Gate,New delhi India',28.610295,77.230797,'India Gate,New delhi India']
];
// Info Window Content
var infoPopupWindowContent = [];
var temp =''
for( i = 0; i < myLocations.length; i++ ) {
temp = ['<div class="info_content">' + '<h3>'+myLocations[i][0]+'</h3>' + '<p>'+myLocations[i][3]+'</p>' + '</div>'];
infoPopupWindowContent.push(temp);
}
// Show multiple location markers on the google map
var infoPopupWindow = new google.maps.InfoWindow(), marker, i;
// generate the markers & place each one on the google map
for( i = 0; i < myLocations.length; i++ ) {
var position = new google.maps.LatLng(myLocations[i][1], myLocations[i][2]);
bounds.extend(position);
marker = new google.maps.Marker({
position: position,
map: gmap,
title: myLocations[i][0]
});
// Set each location marker to a infowindow
google.maps.event.addListener(marker, 'click', (function(marker, i) {
return function() {
infoPopupWindow.setContent(infoPopupWindowContent[i][0]);
infoPopupWindow.open(gmap, marker);
}
})(marker, i));
// Ot Fitting Automatically center the screen
gmap.fitBounds(bounds);
}
// Customize google map zoom level once fitBounds run
var boundsListener = google.maps.event.addListener((gmap), '', function(event) {
this.setZoom(12);
google.maps.event.removeListener(boundsListener);
});
}
</script>
Now we are explaining the above JavaScript code
jQuery(function($) {
var js = document.createElement('script');
js.src = "//maps.googleapis.com/maps/api/js?sensor=false&callback=initialize";
document.body.appendChild(js);
});
Above code is creating our <script>
element on 'onload' event and this will be called when the script has been loaded by the browser and append it as the last child of a body. This code asynchronously load the Google map API on web page.
function initialize() {
var gmap;
var bounds = new google.maps.LatLngBounds();
var mapOptions = {
mapTypeId: 'roadmap'
};
The above initialize method is create an objects and variables which is used to define the map properties.
In initialize methods we use mapTypeId: 'roadmap'. This line of code is tell to display the Road Type of Google map .There are four types of maps in google...
ROADMAP: Display normal 2D road map
SATELLITE: Dispaly photographic map
HYBRID: Display photographic map with roads & city names
TERRAIN: Its used to display map with mountains,rivers
// Display a google map on the web page
gmap = new google.maps.Map(document.getElementById("gmap_canvas"), mapOptions);
gmap.setTilt(45);
This line create new instance for a map with two params
<div>
as a container for map and mapOptions which holds Type of map( we can also use other params like map center map icons etc..)
"gmap.setTilt(45);" is used to enable of 45° view of map
// Multiple location Markers
var myLocations = [
['New delhi, INDIA', 28.610746852715387,77.21122741699219, 'New delhi, INDIA'],
['UPSC New delhi, INDIA', 28.60622574490014,77.22908020019531, 'Union Service Public New delhi, INDIA'],
['National Zoological Park, New Delhi India', 28.6730542, 77.3238588, 'National Zoological Park, Mathura Road, New Delhi, Delhi 110003, India'],
['India Gate,New delhi India',28.610295,77.230797,'India Gate,New delhi India']
];
The above code creating an array of marker locations.
// Set HTML content of POPup Info Window
var infoPopupWindowContent = [];
var temp =''
for( i = 0; i < myLocations.length; i++ ) {
temp = ['<div class="info_content">' + '<h3>'+myLocations[i][0]+'</h3>' + '<p>'+myLocations[i][3]+'</p>' + '</div>'];
infoPopupWindowContent.push(temp);
}
Here we are creating a info content of locations which display on click of location marker in map
// Set each location marker to a infowindow
google.maps.event.addListener(marker, 'click', (function(marker, i) {
return function() {
infoPopupWindow.setContent(infoPopupWindowContent[i][0]);
infoPopupWindow.open(gmap, marker);
}
})(marker, i));
In this above code we set the HTML info content to each marker in Google map
// Fitting Automatically center the screen
gmap.fitBounds(bounds);
The above code responsible for fitting the center for lat & lng into Google map.
Finally, Google map looks on web page as below...
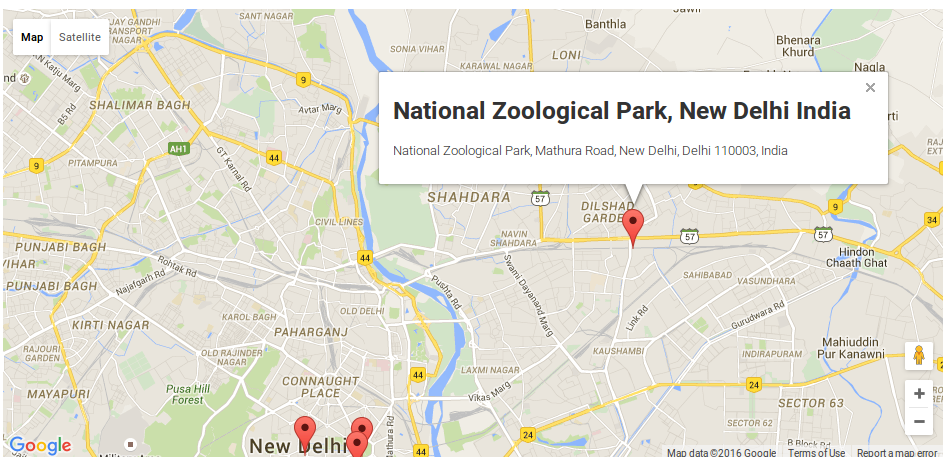
0 Comment(s)