Welcome to FindNerd. Today we are going to discuss custom modules in Nodejs. As we have discussed the in-build modules as well as other modules which are provided by npm. We can also create our own modules. Kindly use the following steps.
Step1: Create a folder with any name for your application files. Here we are creating folder named node_learning
Step2 : Create a folder for your module with any name. Here we are creating folder named train.
Step3 : Create package.json file inside train folder and set the dependencies. You can use the below command for file creation.
npm init
Kindly check the screen-shot below.
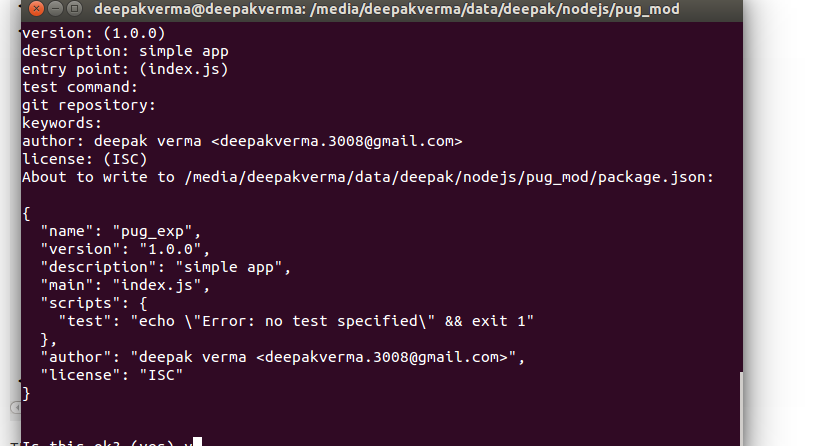
//package.json
{
"name": "train",
"version": "0.0.0",
"description": "A module for keeping track of a train",
"main": "index.js",
"scripts": {
"test": "echo \"Error: no test specified\" && exit 1"
},
"author": "Deepak <deepakverma.3008@gmail.com>",
"license": "BSD-2-Clause"
}
With this file other users can install the dependencies for your module if they are using your module in their applications.
Step 4 : Create index.js file and write the code for your module. Here we are dealing with train informations such as number origin,destination, arrival, departs, actualArrival, actualDeparts. Kindly check the code below.
// index.js
var Train = function(){
this.data = {
number: null,
origin: null,
destination: null,
departs:null,
arrival:null,
actualArrival:null,
actualDeparts:null
};
this.fill = function(info){
for(var prop in this.data){
if(this.data[prop] !== 'undefined'){
this.data[prop] = info[prop];
}
}
};
this.triggerDepart = function(){
this.data.actualDeparts = Date.now();
};
this.triggerArrival = function(){
this.data.actualArrival = Date.now();
};
this.getInformation = function()
{
return this.data;
};
};
module.exports = Train;
In above code we created a function Train and created property or object named data and other functions such as fill, triggerDepart, triggerArrival, getInformation. In the end pass the function to module.exports.
We have created a simple module for train information. Now we will load this module in our application.
Step 5: Kindly check below code.
//main.js
var train = require('./train');
var train_info = {
'number':3434,
'origin':'basre',
'destination':'dfk'
};
var tn = new train();
tn.fill(train_info);
tn.triggerDepart();
console.log(tn.getInformation());
In above code we have loaded the module train using require function and created a object with train information. We call the functions such as fill function to set the train information, triggerDepart to set the actual departure time, triggerArrival function to set the actual arrival of the train, getInformation function to display the flight data on console.
Step 6: You can run the program by using below command.
// go to application folder
node main.js
These are the basic steps to create and load the custom module.
Thank you for being with us!
0 Comment(s)