Compare is an attribute in MVC which is used to compare any of the two properties with one another. In the application, it can be used to compare emailId and password. If both the fields are not having the same value then this attribute will display an error message to the client. Let's understand this attribute with an example.
First add a model by right clicking on Model folder. Employee.cs model is added. Now add the fields you want to compare,as we need to compare email and confirm email and same in the case of password as we are comparing password with compare password field. In this model, you have to add a namespace System.ComponentModel.DataAnnotations to get the Compare attribute in your application. we are using compare with confirm and compare field and giving some error message what we want to display to the client.
using System;
using System.ComponentModel.DataAnnotations;
namespace MvcWebApplication.Models
{
public class Employee
{
[Required]
public string Email { get; set; }
[Required]
[Compare("Email",ErrorMessage ="Email and ConfirmEmail are not same")]
public string confirmEmail { get; set; }
[Required]
public string password { get; set; }
[Required]
[Compare("password", ErrorMessage = "Password and ComparePassword are not same")]
public string comparePassword { get; set; }
}
}
Now add a controller in your application by right clicking on controller folder.
public class EmployeeController : Controller
{
// GET: Employee
public ActionResult Index()
{
return View();
}
}
Now right click on the actionresult and add an Index view. Index view is a strongly typed view which uses all the properties of employee model and uses the template as Create.
@model MvcWebApplication.Models.Employee
@{
ViewBag.Title = "Index";
Layout = "~/Views/Shared/_Layout.cshtml";
}
@using (Html.BeginForm())
{
<div class="form-horizontal">
<h4>Employee</h4>
<hr />
<div class="form-group">
@Html.LabelFor(model => model.Email, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.Email, new { htmlAttributes = new { @class = "form-control" } })
@Html.ValidationMessageFor(model => model.Email, "", new { @class = "text-danger" })
</div>
</div>
<div class="form-group">
@Html.LabelFor(model => model.confirmEmail, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.confirmEmail, new { htmlAttributes = new { @class = "form-control" } })
@Html.ValidationMessageFor(model => model.confirmEmail, "", new { @class = "text-danger" })
</div>
</div>
<div class="form-group">
@Html.LabelFor(model => model.password, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.PasswordFor(model => model.password, new { htmlAttributes = new { @class = "form-control" } })
@Html.ValidationMessageFor(model => model.password, "", new { @class = "text-danger" })
</div>
</div>
<div class="form-group">
@Html.LabelFor(model => model.comparePassword, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.PasswordFor(model => model.comparePassword, new { htmlAttributes = new { @class = "form-control" } })
@Html.ValidationMessageFor(model => model.comparePassword, "", new { @class = "text-danger" })
</div>
</div>
<div class="form-group">
<div class="col-md-offset-2 col-md-10">
<input type="submit" value="Create" class="btn btn-default" />
</div>
</div>
</div>
}
Now Debug your application and see the result by inserting the values in all the fields.
Screenshot for the reference is given below:
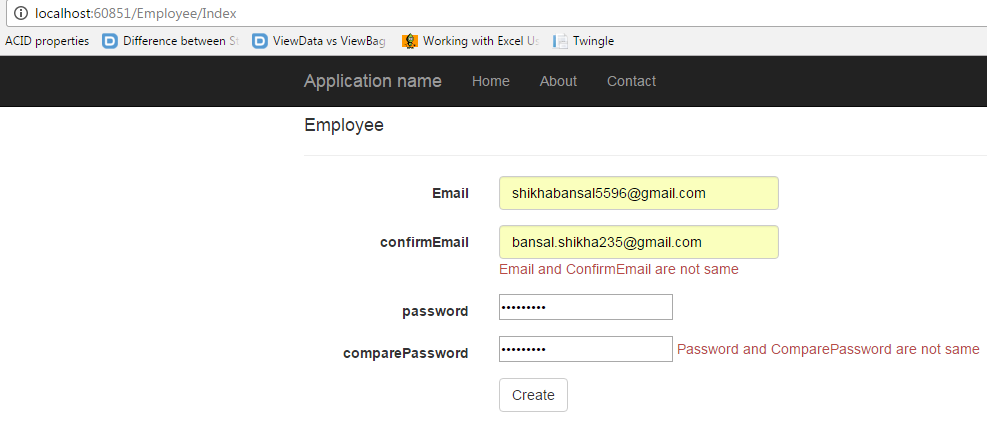
0 Comment(s)