Creating multiple ng-app in single page
Consider a program with multiple ng-app in html page :-
<!DOCTYPE html>
<html>
<head>
<title>In a page only one ng-app works</title>
</head>
<body>
<div ng-app="primaryApp">
<div ng-controller="primaryController">
<p> {{ name }} </p>
</div>
</div>
<div ng-app="secondaryApp">
<div ng-controller="secondaryController">
<p> {{ name }} </p>
</div>
</div>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.4.8/angular.min.js"></script>
<script type="text/javascript">
var primaryApp = angular.module('primaryApp', []);
primaryApp.controller('primaryController', function($scope) {
$scope.name = "Within Primary App";
});
var secondaryApp = angular.module('secondaryApp', []);
secondaryApp.controller('secondaryController', function($scope) {
$scope.name = "Within Secondary App";
});
</script>
</body>
</html>
In above program two ng-app named primaryApp and secondaryApp with their own controller named primaryController and secondaryController are created. These controllers have their own scope variable "name". When above program runs then output is as follows :
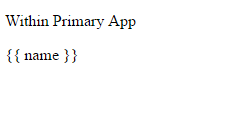
This shows that only the first ng-app will work as a result "primaryApp" work as expected whereas "secondaryApp" does not. There are various ways for running multiple ng-app in an HTML document.
Method 1: Inject modules in the root app as dependencies
In this method a root ng-app is created within <html> or <body> tag then define other multiple ng-app and inject them in root ng-app as dependencies.
<!DOCTYPE html>
<html>
<head>
<title>Injecting modules as dependencies in root app </title>
</head>
<body ng-app="rootApp">
<div id="primaryApp">
<div ng-controller="primaryController">
<p> {{ name }}</p>
</div>
</div>
<div id="secondaryApp">
<div ng-controller="secondaryController">
<p> {{ name }}</p>
</div>
</div>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.4.8/angular.min.js"></script>
<script type="text/javascript">
// dependencies in root module contains defined modules
var rootApp = angular.module('rootApp', ['primaryApp','secondaryApp']);
var primaryApp = angular.module('primaryApp', []);
primaryApp.controller('primaryController', function ($scope) {
$scope.name = "Within Primary App";
});
var secondaryApp = angular.module('secondaryApp', []);
secondaryApp.controller('secondaryController', function ($scope) {
$scope.name = "Within Secondary App";
});
</script>
</body>
</html>
Output :
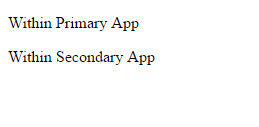
Method 2 : Bootstrap the defined second module manually.
In this method, first ng-app is auto-bootstrapped by Angular and the second ng-app module is bootstrapped manually.
<!DOCTYPE html>
<html>
<head>
<title>Bootstrapping the second module manually</title>
</head>
<body>
<div ng-app="primaryApp">
<div ng-controller="primaryController">
<p> {{ name }}</p>
</div>
</div>
<div id="secondaryApp">
<div ng-controller="secondaryController">
<p> {{ name }}</p>
</div>
</div>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.4.8/angular.min.js"></script>
<script type="text/javascript">
var primaryApp = angular.module('primaryApp', []);
primaryApp.controller('primaryController', function($scope) {
$scope.name = "Within Primary App";
});
var secondaryApp = angular.module('secondaryApp', []);
secondaryApp.controller('secondaryController', function($scope) {
$scope.name = "Within Secondary App";
});
// manually boostrapping the "secondaryApp"
var secondmodule = document.getElementById('secondaryApp');
angular.element(document).ready(function() {
angular.bootstrap(secondmodule, ['secondaryApp']);
});
</script>
</body>
</html>
Output :
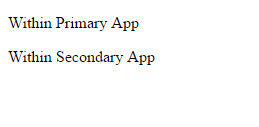
Method 3 : Both the defined modules can be Bootstrapped manually as in above program.
0 Comment(s)