Google map utility library is free and open source library of classes which provides advance features on google map like customization, clustering, importing geoJson to map, importing KML to map and encoding /decoding polylines.
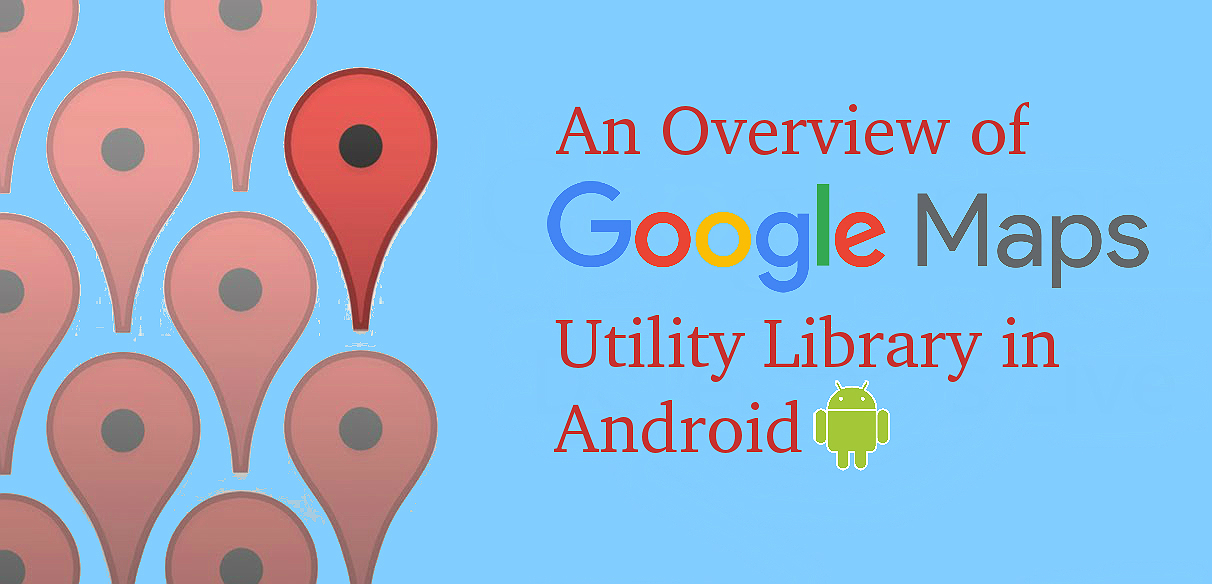
SETUP:
Add the following dependency to your app's Gradle build file:
dependencies {
compile 'com.google.maps.android:android-maps-utils:0.5+'
}
FEATURES
- Cluster Management : Cluster management helps you to manage markers at different zoom levels ,i.e you can place multiple markers in map and make map readable easily,Clustering behaves differently for zoom level (High/Low) zoom. On high Zoom all markers will be visible and low zo0m markers disappears indicating number of markers on map
To use Clustering in google map read below code
private void setUpClusterer() {
// Position the map.
mMap.moveCamera(CameraUpdateFactory.newLatLngZoom(new LatLng(51.503186, -0.126446), 10));
// Initialize the manager with the context and the map.
// (Activity extends context, so we can pass 'this' in the constructor.)
mClusterManager = new ClusterManager<MyItem>(this, mMap);
// Point the map's listeners at the listeners implemented by the cluster
// manager.
mMap.setOnCameraIdleListener(mClusterManager);
mMap.setOnMarkerClickListener(mClusterManager);
// Add cluster items (markers) to the cluster manager.
addItems();
}
private void addItems() {
// Set some lat/lng coordinates to start with.
double lat = 51.5145160;
double lng = -0.1270060;
// Add ten cluster items in close proximity, for purposes of this example.
for (int i = 0; i < 10; i++) {
double offset = i / 60d;
lat = lat + offset;
lng = lng + offset;
MyItem offsetItem = new MyItem(lat, lng);
mClusterManager.addItem(offsetItem);
}
}
//you class must implement clusterItem
public class MyItem implements ClusterItem {
private LatLng mPosition;
private String mTitle;
private String mSnippet;
public MyItem(double lat, double lng) {
mPosition = new LatLng(lat, lng);
}
public MyItem(double lat, double lng, String title, String snippet) {
mPosition = new LatLng(lat, lng);
mTitle = title;
mSnippet = snippet;
}
@Override
public LatLng getPosition() {
return mPosition;
}
@Override
public String getTitle() {
return mTitle;
}
@Override
public String getSnippet() {
return mSnippet;
}
}
2. Custom marker using bubble icons:
Now you can add makers with different Style, colors, you can add makers like info window, change the orientation. Add a IconGenerator class
will display snippets of information on your markers
//create icon factory,set color ,set style,set orientation
IconGenerator iconFactory = new IconGenerator(this);
addIcon(iconFactory, "Default", new LatLng(-33.8696, 151.2094));
iconFactory.setColor(Color.CYAN);
addIcon(iconFactory, "other color", new LatLng(-33.9360, 151.2070));
iconFactory.setRotation(90);
iconFactory.setStyle(IconGenerator.STYLE_RED);
addIcon(iconFactory, "90 degrees", new LatLng(-33.8858, 151.096));
// add custom marker to map using icon generator class
private void addIcon(IconGenerator iconFactory, CharSequence text, LatLng position) {
MarkerOptions markerOptions = new MarkerOptions().
icon(BitmapDescriptorFactory.fromBitmap(iconFactory.makeIcon(text))).
position(position).
anchor(iconFactory.getAnchorU(), iconFactory.getAnchorV());
mMap.addMarker(markerOptions);
}
3. Add HeatMap to google map:
HeatMap will make easy to understand the intensity of distribution of markers, heatmaps use different color and different shapes to show the distribution of the data. Creating a HeatmapTileProvider
, passing it a collection of LatLng
objects on the map.
// add the demo json in your raw folder
[
{"lat" : -37.1886, "lng" : 145.708 } ,
{"lat" : -37.8361, "lng" : 144.845 } ,
{"lat" : -38.4034, "lng" : 144.192 } ,
{"lat" : -38.7597, "lng" : 143.67 } ,
{"lat" : -36.9672, "lng" : 141.083 }
]
Find the code snippet
private ArrayList<LatLng> readItems(int resource) throws JSONException {
ArrayList<LatLng> list = new ArrayList<LatLng>();
InputStream inputStream = getResources().openRawResource(resource);
String json = new Scanner(inputStream).useDelimiter("\\A").next();
JSONArray array = new JSONArray(json);
for (int i = 0; i < array.length(); i++) {
JSONObject object = array.getJSONObject(i);
double lat = object.getDouble("lat");
double lng = object.getDouble("lng");
list.add(new LatLng(lat, lng));
}
return list;
}
private void addHeatMap() {
List<LatLng> list = null;
// Get the data: latitude/longitude positions of police stations.
try {
list = readItems(R.raw.test);
} catch (JSONException e) {
Toast.makeText(this, "Problem reading list of locations.", Toast.LENGTH_LONG).show();
}
// Create the gradient.
int[] colors = {
Color.rgb(102, 225, 0), // green
Color.rgb(255, 0, 0) // red
};
float[] startPoints = {
0.2f, 1f
};
Gradient gradient = new Gradient(colors, startPoints);
// Create a heat map tile provider, passing it the latlngs of the police stations.
HeatmapTileProvider mProvider = new HeatmapTileProvider.Builder()
.data(list)
.gradient(gradient)
.build();
// Add a tile overlay to the map, using the heat map tile provider.
TileOverlay mOverlay = mMap.addTileOverlay(new TileOverlayOptions().tileProvider(mProvider));
}
Note: Make sure map is setup in your application
If you have any question, please feel free to write in comment section below.
Happy coding
0 Comment(s)