Android Support Library 23.2, Google has given support for Bottom Sheets. AS per Material Design, Bottom Sheets are elements displayed only as a result of a user-initiated action, used to reveal more content from the bottom upwards. They slide from the bottom, they’re also integrated with the app to display supporting content (like Google Maps).
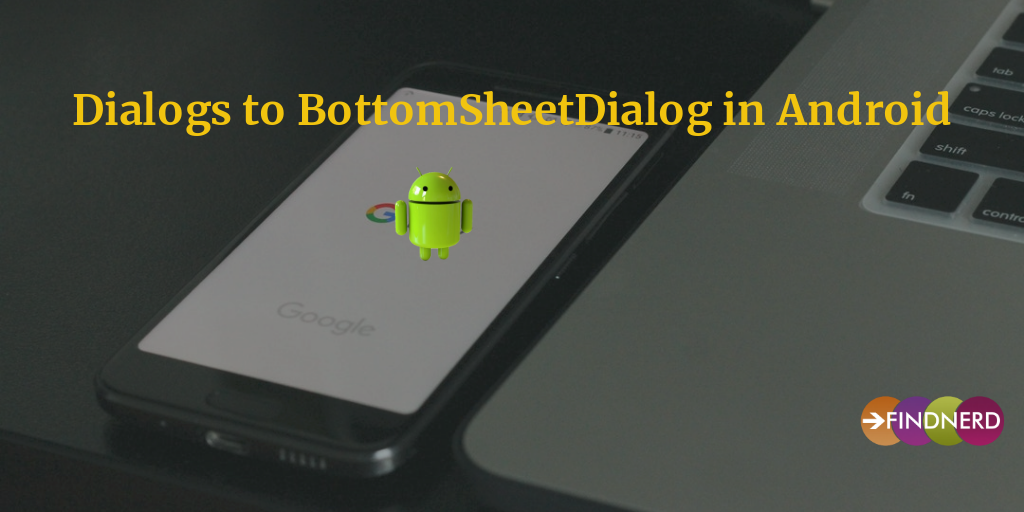
Below is the Activity class where I have implemented BottomSheetDialogs
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
init();
}
private void init() {
Button btOpen = findViewById(R.id.bt_open);
btOpen.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
openBottomSheet();
}
});
}
private void openBottomSheet() {
View view = getLayoutInflater().inflate(R.layout.content_bottom_sheet, null);
final BottomSheetDialog dialog = new BottomSheetDialog(this, R.style.CustomBottomSheetDialogTheme);
dialog.requestWindowFeature(Window.FEATURE_NO_TITLE);
dialog.getWindow().setBackgroundDrawable(new ColorDrawable(Color.TRANSPARENT));
dialog.setContentView(view);
TextView refresh = view.findViewById(R.id.btn_refresh);
TextView delete = view.findViewById(R.id.btn_delete);
TextView save = view.findViewById(R.id.btn_save);
TextView cancel = view.findViewById(R.id.btn_cancel);
refresh.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
show("Refresh");
dialog.dismiss();
}
});
delete.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
show("Delete");
dialog.dismiss();
}
});
save.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
show("Save");
dialog.dismiss();
}
});
cancel.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
dialog.dismiss();
}
});
dialog.show();
}
private void show(String text) {
Toast.makeText(this, text, Toast.LENGTH_SHORT).show();
}
}
Designed code of BottomSheetDialog is here -
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_gravity="center_horizontal"
android:background="@android:color/transparent"
android:gravity="center_horizontal"
android:orientation="vertical"
android:paddingLeft="30dp"
android:paddingRight="30dp"
android:paddingTop="@dimen/ten_dp"
app:layout_anchorGravity="top"
app:layout_behavior="android.support.design.widget.BottomSheetBehavior">
<TextView
android:id="@+id/btn_refresh"
style="@style/action_button_bs"
android:layout_width="match_parent"
android:layout_gravity="center"
android:layout_marginTop="2dp"
android:background="@drawable/rounded_button"
android:gravity="center"
android:text="@string/refresh" />
<TextView
android:id="@+id/btn_delete"
style="@style/action_button_bs"
android:layout_width="match_parent"
android:layout_marginTop="2dp"
android:background="@drawable/rounded_button"
android:gravity="center"
android:text="@string/delete"
android:textAllCaps="false" />
<TextView
android:id="@+id/btn_save"
style="@style/action_button_bs"
android:layout_width="match_parent"
android:layout_marginBottom="8dp"
android:text="@string/save"
android:layout_marginTop="2dp"
android:background="@drawable/rounded_button"
android:gravity="center" />
<TextView
android:id="@+id/btn_cancel"
style="@style/action_button_bs"
android:layout_width="match_parent"
android:text="@string/cancel"
android:textColor="@android:color/holo_red_dark"
android:layout_marginBottom="8dp"
android:layout_marginTop="@dimen/ten_dp"
android:background="@drawable/rounded_button"
android:gravity="center" />
</LinearLayout>
You can also download the sample code zip file at the bottom.
0 Comment(s)