Hello Readers! In this blog we are going to create an api for inserting the data into database and then passing the data to the device in JSON format using php.
Step 1 : Firstly you are required to create a database. Here we have created a sample database named “rest_api”.
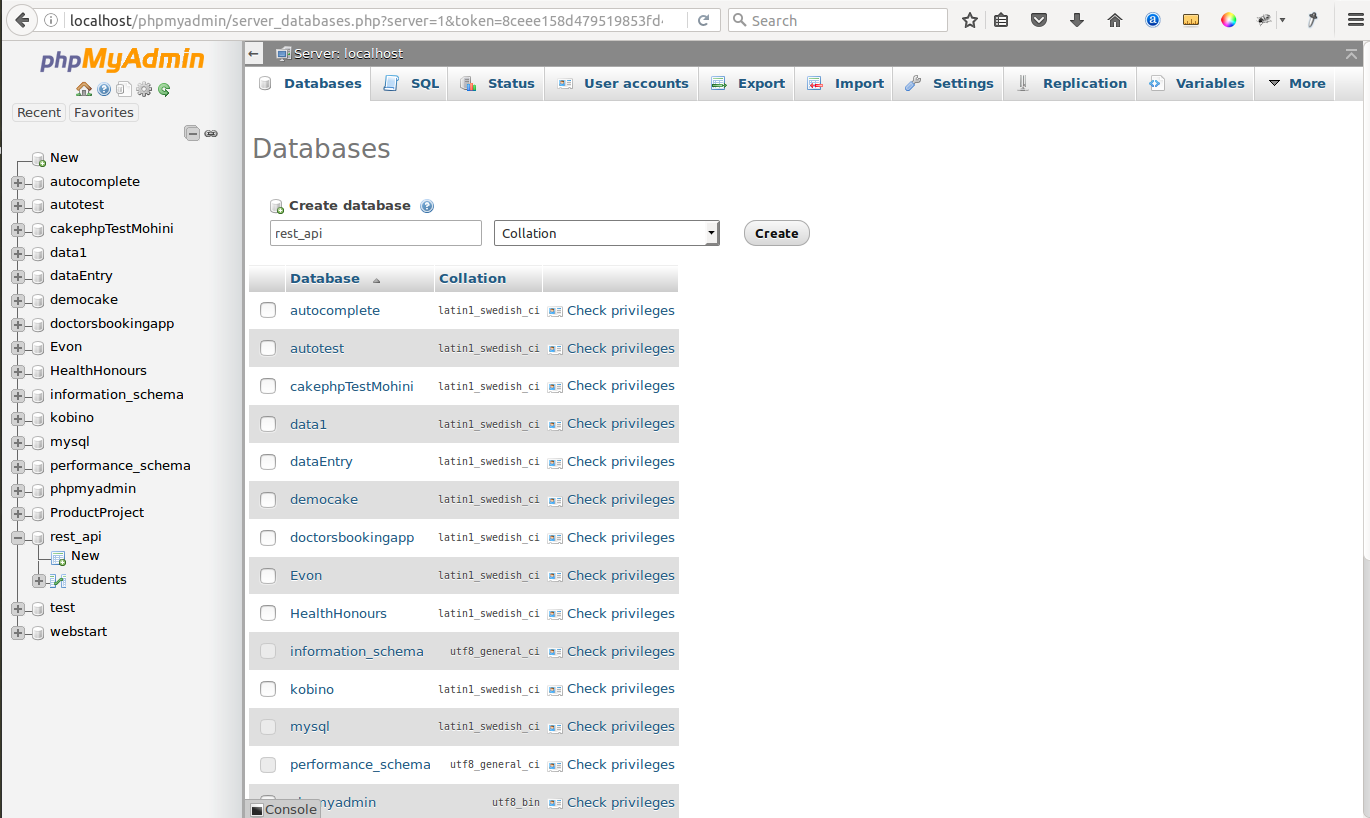
Step 2: Next, create a table named “students” with fields “id”, “first_name”, “last_name”, “phone_no”.
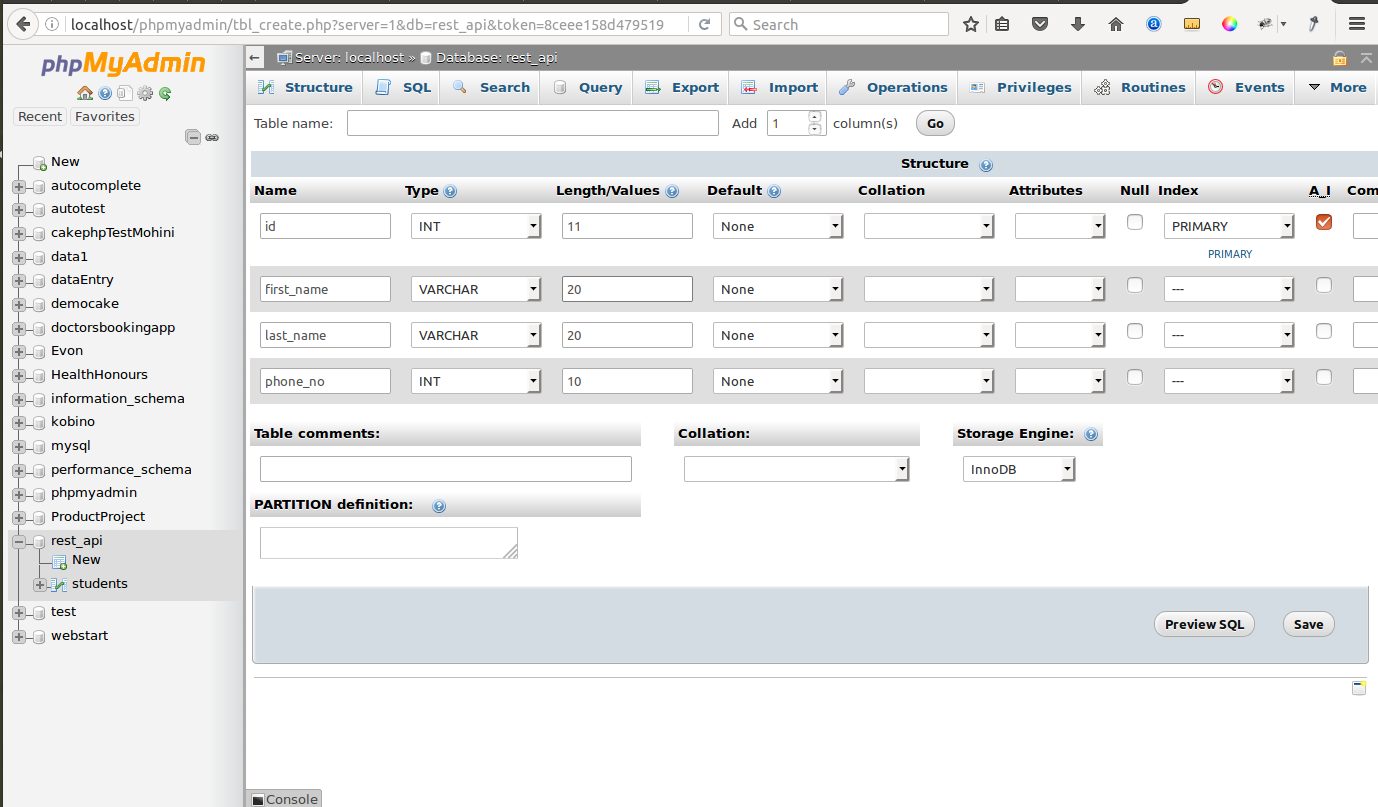
Step 3 : Establish connection to mysql database with the help of mysqli_connect() function.
$conn = mysqli_connect('localhost',"root","","rest_api");
here, “localhost” is the host name, “root” is mysql username, “” is the password which is blank in this case and “rest_api” is the database to be used.
Step 4: Create a function to insert data
function insert_data()
{
ini_set("display_errors", 1);
$posts = array();
$first_name = $_REQUEST['first_name'];
$last_name = $_REQUEST['last_name'];
$phone_no= $_REQUEST['phone_no'];
$sql = "INSERT INTO students(first_name, last_name, phone_no) VALUES ('$first_name' , '$last_name', '$phone_no)";
// sql query to insert values in the table
$query=mysql_query($sql);
if($query)
{
$posts['response'] = array("success" => "1", "msg" => "Inserted Successfully");
}
else
{
$posts['response'] = array("success" => "0", "msg" => "Not Inserted");
}
echo json_encode($posts);
}
Here we have created a function named “insert_data” . $_REQUEST is a super global variable which is used to collect the data when we hit the function. Next we will check whether the data is inserted successfully or not then we will pass the message through json using the function json_encode() function. json_encode() function is used to convert the php array into json string.
Here is the complete php file code:
<?php
$conn = mysqli_connect('localhost',"root","","rest_api");
if ($_SERVER["REQUEST_METHOD"] === 'POST')
{
$first_name = $_REQUEST["first_name"];
$last_name = $_REQUEST["last_name"];
$phone_no = $_REQUEST["phone_no"];
$query = "INSERT INTO students (`first_name`,`last_name`,`phone_no`) VALUES('$first_name','$last_name','$phone_no')";
$result = $conn->query($query);
if ($result == 1)
{
$data["message"] = "data saved successfully";
$data["status"] = "Ok";
}
else
{
$data["message"] = "data not saved successfully";
$data["status"] = "error";
}
}
else
{
$data["message"] = "Format not supported";
$data["status"] = "error";
}
echo json_encode($data);
Happy Coding :)
1 Comment(s)