Upload multiple images using jQuery Ajax / Drag & Drop Images
Hello friends, welcome to findnerd. Today I am going to tell you how to upload multiple images using jQuery and Ajax in Cakephp 3. You can also drag and drop images and upload them. Lets start by creating uploads layout in directory src/Template/Layout/
uploads.ctp
<!DOCTYPE html>
<html>
<head>
<title>How to Upload multiple images jQuery Ajax</title>
<meta http-equiv="Content-Type" content="text/html;charset=utf-8" />
<style type="text/css">
img {border-width: 0}
* {font-family:'Lucida Grande', sans-serif;}
</style>
<?php echo $this->Html->css('uploadfilemulti.css'); ?>
<?php echo $this->Html->script(['jquery-1.8.0.min','jquery.fileuploadmulti.min']); ?>
</head>
<body>
<?php echo $this->fetch('content'); ?>
<script>
$(document).ready(function()
{
var settings = {
url: "uploads",
method: "POST",
allowedTypes:"jpg,png,gif,doc,pdf,zip",
fileName: "myfile",
multiple: true,
onSuccess:function(files,data,xhr)
{
$("#status").html("<font color='green'>Upload is success</font>");
},
afterUploadAll:function()
{
alert("all images uploaded!!");
},
onError: function(files,status,errMsg)
{
$("#status").html("<font color='red'>Upload is Failed</font>");
}
}
$("#mulitplefileuploader").uploadFile(settings);
});
</script>
</body>
</html>
Now create UploadsController at src/Controller/
. Write the following lines and save the file.
<?php
namespace App\Controller;
use App\Controller\AppController;
use Cake\Event\Event;
/**
* Uploads Controller
*
* @property \App\Model\Table\UsersTable $Users
*/
class UploadsController extends AppController
{
public function beforeFilter(Event $event)
{
parent::beforeFilter($event);
// Allow users to register and logout.
$this->Auth->allow(['add', 'logout']);
}
/**
* Index method
*
* @return \Cake\Network\Response|null
*/
public function index()
{
$this->viewBuilder()->layout('uploads');
//If directory doesnot exists create it.
$output_dir = WWW_ROOT ."files/";
if(isset($_FILES["myfile"]))
{
$ret = array();
$error =$_FILES["myfile"]["error"];
{
if(!is_array($_FILES["myfile"]['name'])) //single file
{
$RandomNum = time();
$ImageName = str_replace(' ','-',strtolower($_FILES['myfile']['name']));
$ImageType = $_FILES['myfile']['type']; //"image/png", image/jpeg etc.
$ImageExt = substr($ImageName, strrpos($ImageName, '.'));
$ImageExt = str_replace('.','',$ImageExt);
$ImageName = preg_replace("/\.[^.\s]{3,4}$/", "", $ImageName);
$NewImageName = $ImageName.'-'.$RandomNum.'.'.$ImageExt;
move_uploaded_file($_FILES["myfile"]["tmp_name"],$output_dir. $NewImageName);
//echo "<br> Error: ".$_FILES["myfile"]["error"];
$ret[] = $output_dir.$NewImageName;
}
else
{
$fileCount = count($_FILES["myfile"]['name']);
for($i=0; $i < $fileCount; $i++)
{
$RandomNum = time();
$ImageName = str_replace(' ','-',strtolower($_FILES['myfile']['name'][$i]));
$ImageType = $_FILES['myfile']['type'][$i]; //"image/png", image/jpeg etc.
$ImageExt = substr($ImageName, strrpos($ImageName, '.'));
$ImageExt = str_replace('.','',$ImageExt);
$ImageName = preg_replace("/\.[^.\s]{3,4}$/", "", $ImageName);
$NewImageName = $ImageName.'-'.$RandomNum.'.'.$ImageExt;
$ret[$NewImageName]= $output_dir.$NewImageName;
move_uploaded_file($_FILES["myfile"]["tmp_name"][$i],$output_dir.$NewImageName );
}
}
}
echo json_encode($ret);
}
}
}
Now create a view file for uploading images at: src/Template/Uploads/index.ctp
<h2>Upload multiple images using jQuery Ajax</h2>
<div id="mulitplefileuploader">Upload</div>
<div id="status"></div>
You can download css and javascript files and paste them in the following directory:
i) webroot/css/uploadfilemulti.css
ii) webroot/js/jquery.fileuploadmulti.min.js
iii) webroot/js/jquery-1.8.0.min.js
Now you can run this example by accessing the url: http://localhost/your_project/uploads
. You will get the following output:
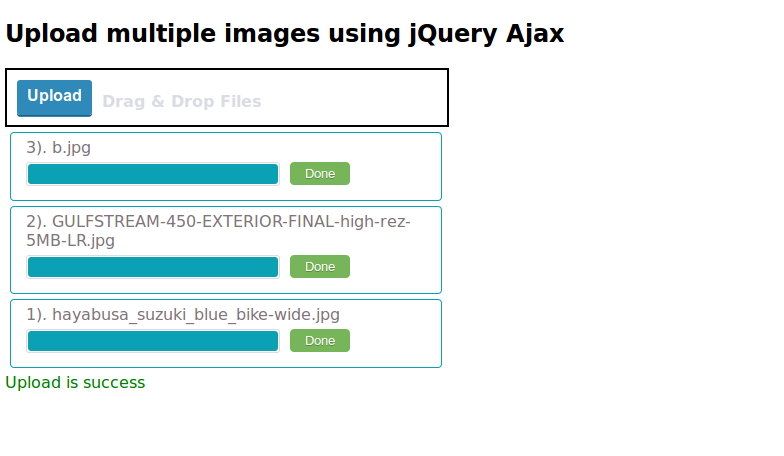
**Note: Don't forget to give the read and write permissions to the "webroot/files/
" directory. Here images will be uploaded.
All done!
Thanks for reading the blog.
1 Comment(s)