In this blog we will learn to create a simple shopping cart application using cakephp. This application is very basic and simple you can download the zip file attached at the end of the blog and customize it as per your requirements. In this example we will be displaying list of products from database.
Firstly user is required to register before they can actually view the products list.
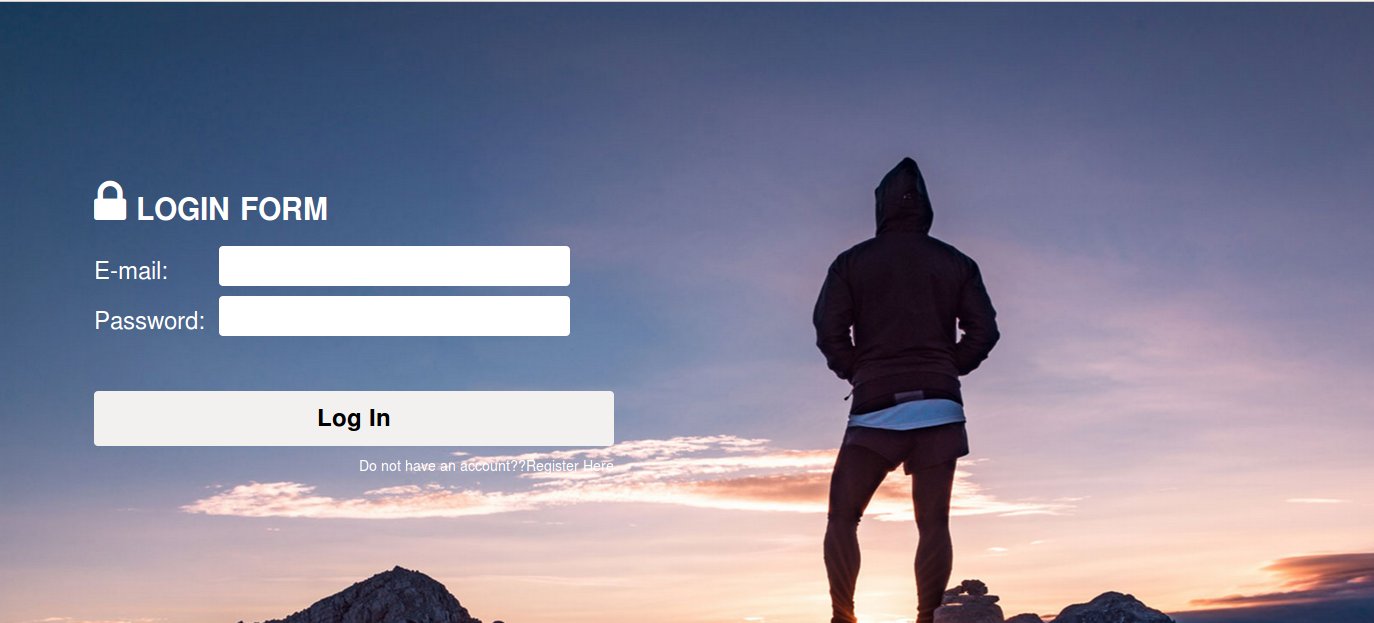
With valid user id they can access the product list. Here we have three products namely, P1, P2, P3. For each product, we have option for selecting product quantity and adding it to cart.
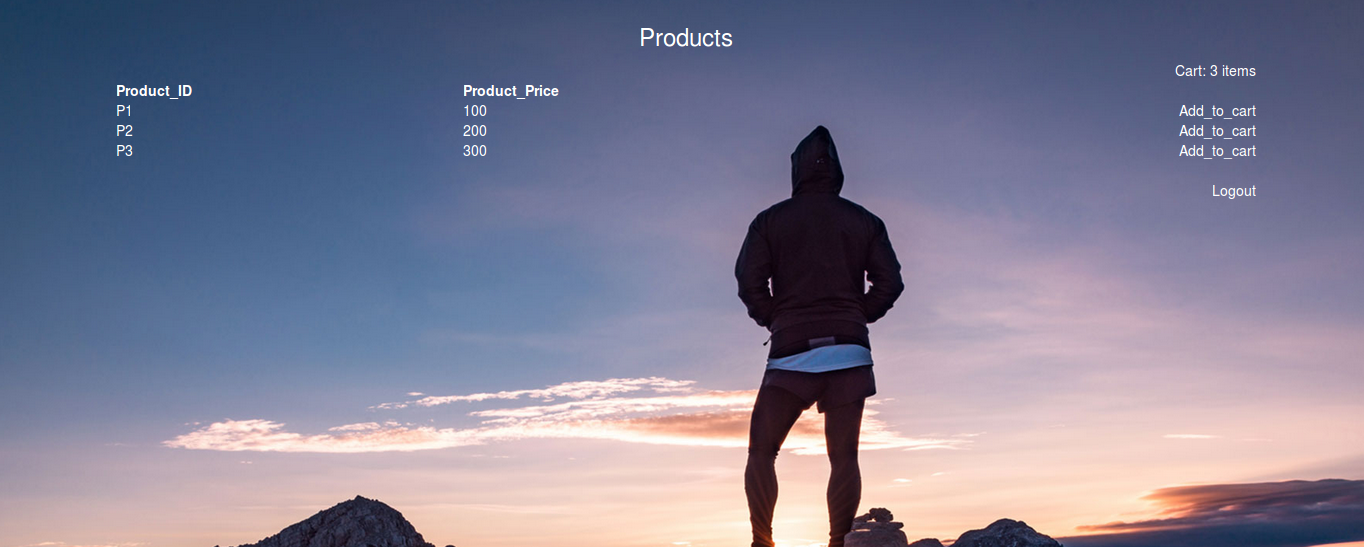
Add_to_cart : Following code is used to fetch the list of products from the database and displays products with add to cart option.
<table align="center">
<tr>
<th>Product_ID</th>
<th>Product_Price</th>
</tr>
<?php
//link to cart with number of items
echo $this->Html->link('Cart: '. $totalItems . ' items', array('action'=> 'showcart'));
?>
<?php foreach($products as $product): ?>
<tr>
<td><?php echo $product['Product']['product_id'];?></td>
<td><?php echo $product['Product']['product_price'];?></td>
<td><?php echo $this->Html->link('Add_to_cart',array('action'=>'add_to_cart',$product['Product']['id']));?></td>
</tr>
<?php endforeach; ?>
</table>
<br>
<?php echo $this->Html->link('Logout',array('controller'=>'users','action'=>'/logout')); ?>
Whenever user clicks on the add to cart option following code of lines is used to check if the selected product already exists in cart, then the quantity will increment by 1.
$check = $this->Cart->find("first", array(
"conditions"=>array(
"Cart.user_id"=>$userId,
"Cart.product_id"=>$id
)
)
);
$data =array();
if (!empty($check))
{
// update quantity with increment of 1 ...
$data["Cart"]["id"] = $check["Cart"]["id"];
$data["Cart"]["quantity"] = $check["Cart"]["quantity"] + 1;
}
else
{
// insert new Cart
$data["Cart"]["user_id"] = $userId;
$data["Cart"]["product_id"] = $id;
$data["Cart"]["quantity"] = 1;
$data["Cart"]["price"] = $product["Product"]["product_price"];
}
if ($this->Cart->save($data))
{
$this->Session->setFlash("Item Added successfully");
$this->redirect(array("action"=>'product'));
}
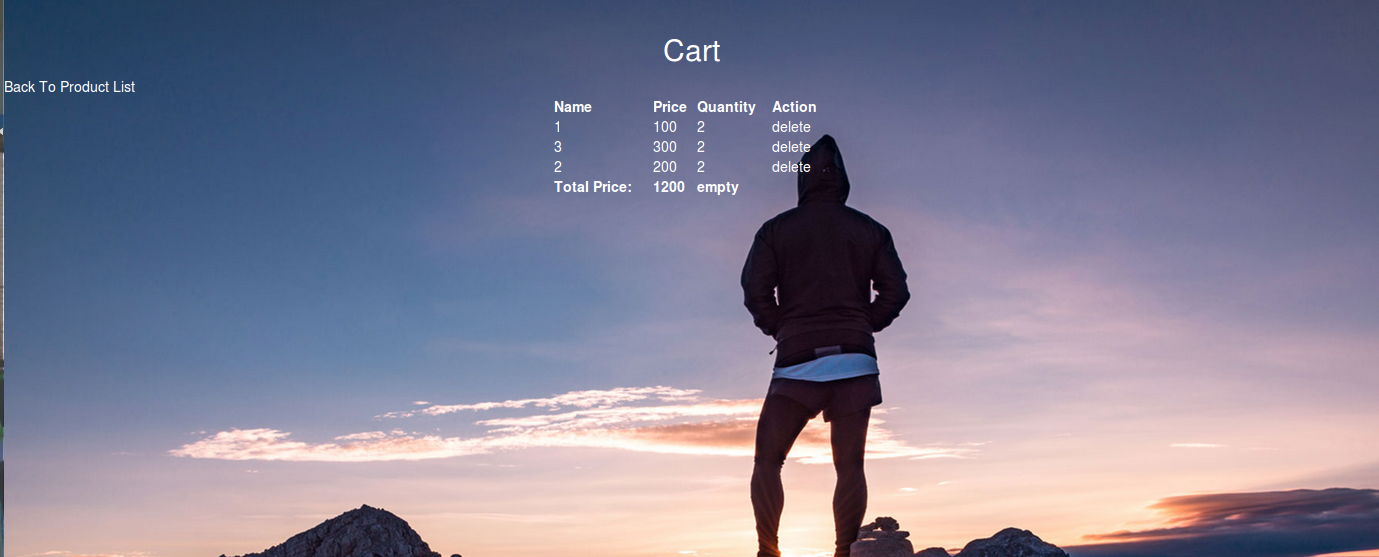
Code to increment the quantity counter :
function cart($id = null)
{
$this->Product->id = $id;
if(!$this->Product->exists())
{
throw new NotFoundException(__('Invalid product'));
}
$productIds = $this->Product->find("list", array("fields"=>'Product.product_id'));
//$product = $this->Product->read(null, $id);
// unset($_SESSION['Cart']);
$productsInCart = $this->Session->read('Cart');
//pr($productsInCart);die;
$amount=count($productsInCart);
$this->Session->write('Cart.', $this->Product->read(null, $id));
//pr($ans);die;
$this->Session->write('Counter', $amount + 1);
$this->Session->setFlash(__('Product added to cart'));
$this->redirect('showcart');
}
Code to get the total amount of the added products :
public function product(){
//find all products
$products = $this->Product->find('all');
$countCart = $carts = $this->Cart->find("count", array(
"conditions" => array(
"Cart.user_id" => $this->Auth->user('id')
)
)
);
$this->set("totalItems", $countCart);
//set counter to display number of products in a cart
$counter = 0;
if ($this->Session->read('Counter')) {
$counter = $this->Session->read('Counter');
}
//pass variable to view
$this->set(compact('products', 'counter'));
}
Delete or Empty : next user has two options either they can delete product from the list or they can empty the cart.
public function delete($id = null)
{
// echo($id);die();
if (is_null($id))
{
throw new NotFoundException(__l('Invalid request'));
}
$del=$this->Cart->deleteAll(array('Cart.id'=>$id));
//echo $del;die;
return $this->redirect(array('action' => 'showcart'));
}
public function empty_cart()
{
//delete cart with all elements and counter
$del=$this->Cart->deleteAll(array('1 = 1'));
$this->redirect(array('controller' => 'users', 'action' => 'showcart'));
}
For complete code package click here.
Happy Coding!
0 Comment(s)