Using a segue, we can pass data between view controllers.Here,the destination view controller will receive the data and will be able to operate on it.
When a transition happens,the prepare(for:sender) method is being called.
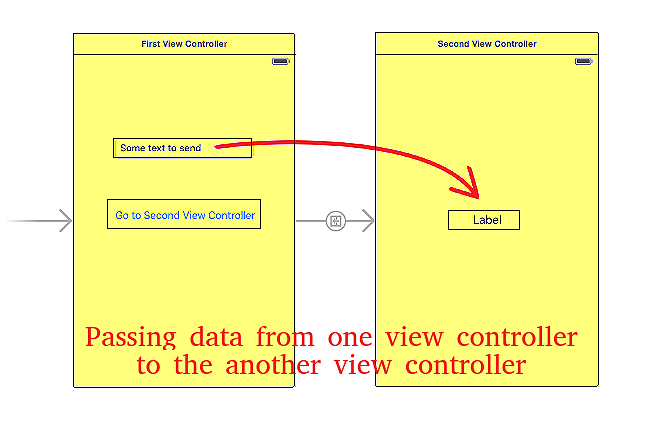
Let's take an example. Suppose we have two view controllers namely SourceViewController and DestinationViewController. Here, our intention is to provide the DestinationViewController a way so that it can operate on some data provided by the SourceViewController.
Here, The main motive is to set the DestinationViewController's model so it can operate on that model and perform its intended purpose.
As we all know, Segues always create a new instance of an view controller. When a segue happens, the source view controller gets a chance to prepare the destination view controller (may be setting up its model and display characteristics). But remember, at the time of preparing,the destination view controller's outlets aren't being set.
struct Data {}
class SourceViewController: UIViewController {}
class DestinationViewController: UIViewController {}
class DestinationViewController: UIViewController {
var data: Data?
}
The triggered segue, an object in itself, is passed as a parameter to prepare(for:sender) method. The source view controller can access the destination view controller using the destinationViewController property.
class SourceViewController: UIViewController {
override func prepare(for segue: UIStoryboardSegue, sender: Any?) {
let data = Data()
if let destinationViewController = segue.destination as? DestinationViewController {
destinationViewController.data = data
}
}
}
Now, the DestinationViewController will have data to operate upon. To differntiate between different segues when there are more than one, we can either use an optional binding like in the example or the identifier property of the segue. The identifier is a string you we can set for each segue in the storyboard.
After preparing a destination view controller and once it is being loaded up in the memory,the following is the order:
- Its outlets gets set.
- viewDidLoad method gets called.(this method is the best place to put set up code i.e initialization settings needed for the destination view controller.Also, don't initialize things that are geometry dependent here because the geometry of our view (its bounds) is not set here,we don't know on which screen we are going to be.).
- These method will be called each time our controller's view goes on/off screen.They are viewDidAppear, viewWillAppear, viewDidDisappear and viewWillDisappear.
Thanks for reading. For any question, feel free to write in comments.
0 Comment(s)