Here is a small example of custom search bar
1. Go to storyboard, take TextField place it at below navigation bar (we will type text here to find any string)
2. Set delegate of TextField
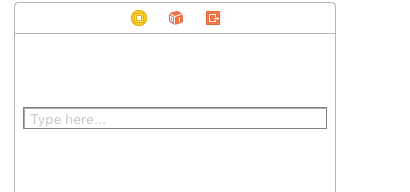
Come to ViewController class now
Create IBOutlet of TextField eg -
@IBOutlet weak var searchText: UITextField!
Now declare 2 array globally one for storing all element and second for storing result of filtered data
var filteredArray:NSArray = [] //to store filtered data
var shoppingList:NSArray = [] //to store whole data
In “viewDidLoad” define your array
override func viewDidLoad() {
super.viewDidLoad()
shoppingList = ["Eggs","Fruits","milk","Rice","Vegetables","Milk","oil","apple","pear","banana",
"raspberry]//you can place dynamic data instead of this
// Do any additional setup after loading the view.
}
After this we will do some code inside “shouldChangeCharactersInRange” to know string type by user
func textField(textField: UITextField, shouldChangeCharactersInRange range: NSRange, replacementString string: String) -> Bool{
let string1 = string
let string2 = searchText.text
var finalString = ""
if string.characters.count > 0 { // if it was not delete character
finalString = string2! + string1
}
else if string2?.characters.count > 0{ // if it was a delete character
finalString = String(string2!.characters.dropLast())
}
filteredArray(finalString)// pass the search String in this method
return true
}
func filteredArray(searchString:NSString){// we will use NSPredicate to find the string in array
let predicate = NSPredicate(format: "SELF contains[c] %@",searchString) // This will give all element of array which contains search string
//let predicate = NSPredicate(format: "SELF BEGINSWITH %@",searchString)// This will give all element of array which begins with search string (use one of them)
filteredArray = shoppingList.filteredArrayUsingPredicate(predicate)
print(filteredArray)
}
By above lines of code we will get our matched result, Now it’s time to display this searched result in controller
For this , go to storyboard
1. Now take a tableView and set a prototype cell in it and place it below the TextFiled(we will show search result here) .
2. Set Delegate and DataSource of TableView.
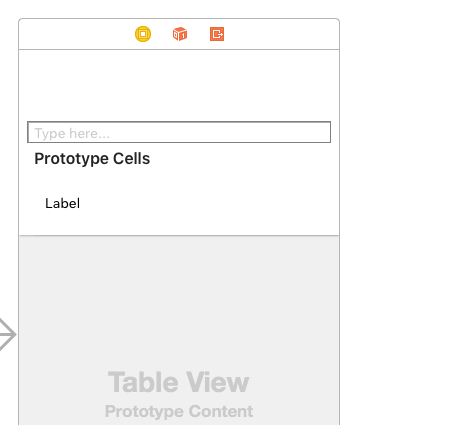
3. Place a label inside your prototype cell, and give IBOutlet of this label in your UITableViewCell (ShoppingDataViewCell in this case)
eg: @IBOutlet weak var searchResultTxt: UILabel!
Now come to your ViewController class
Take IBOutlet of your tableViewCell , eg:
@IBOutlet weak var tableView: UITableView!
Now do some line of code in DataSource method of TableView
func tableView(tableView: UITableView, cellForRowAtIndexPath indexPath: NSIndexPath) -> UITableViewCell {
let cell:ShoppingDataViewCell = tableView.dequeueReusableCellWithIdentifier("ShoppingDataViewCell", forIndexPath: indexPath)as! ShoppingDataViewCell
cell.searchResultTxt.text = filteredArray[indexPath.row] as! String
return cell
}
func tableView(tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
return filteredArray.count;
}
And don’t forget to reload you TableView inside this “filteredArray(searchString:NSString)” function
func filteredArray(searchString:NSString){// we will use NSPredicate to find the string in array
let predicate = NSPredicate(format: "SELF contains[c] %@",searchString) // This will give all element of array which contains search string
//let predicate = NSPredicate(format: "SELF BEGINSWITH %@",searchString)// This will give all element of array which begins with search string
filteredArray = shoppingList.filteredArrayUsingPredicate(predicate)
tableView.reloadData()
print(filteredArray)
}
Now run your app and you will see output like below.
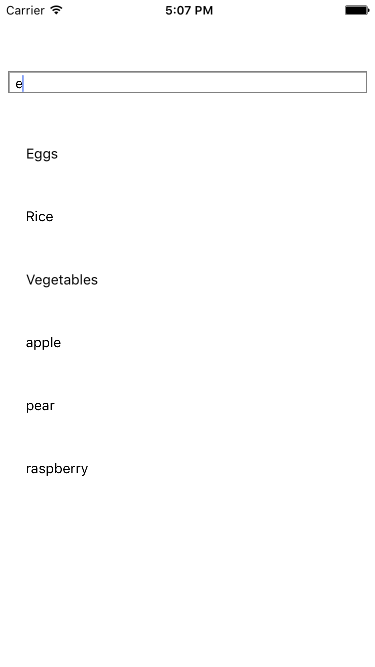
0 Comment(s)