Let us start with the simple CRUD (Create, Read, Update and Delete) Application. Before we begin let me split the processes we are going to do this this blog into the following steps:
- Creating the Database
- Create models
- Create controllers & views for Read data
- Create controllers & views for Display data
- Create controllers & views for Edit data
- Create controllers & views for Delete data
Step 1: Create the database
Create a database with name “Evon” then create a table in the database and name it employees. Then give the table some fields. Then put the following configuration parameters into the app/Config/database.php file.
<?php
class DATABASE_CONFIG {
public $default = array(
'datasource' => 'Database/Mysql',
'persistent' => false,
'host' => 'localhost',
'login' => 'root',
'password' => '',
'database' => 'Evon',
'prefix' => '',
//'encoding' => 'utf8',
);
public $test = array(
'datasource' => 'Database/Mysql',
'persistent' => false,
'host' => 'localhost',
'login' => 'user',
'password' => 'password',
'database' => 'test_database_name',
'prefix' => '',
//'encoding' => 'utf8',
);
}
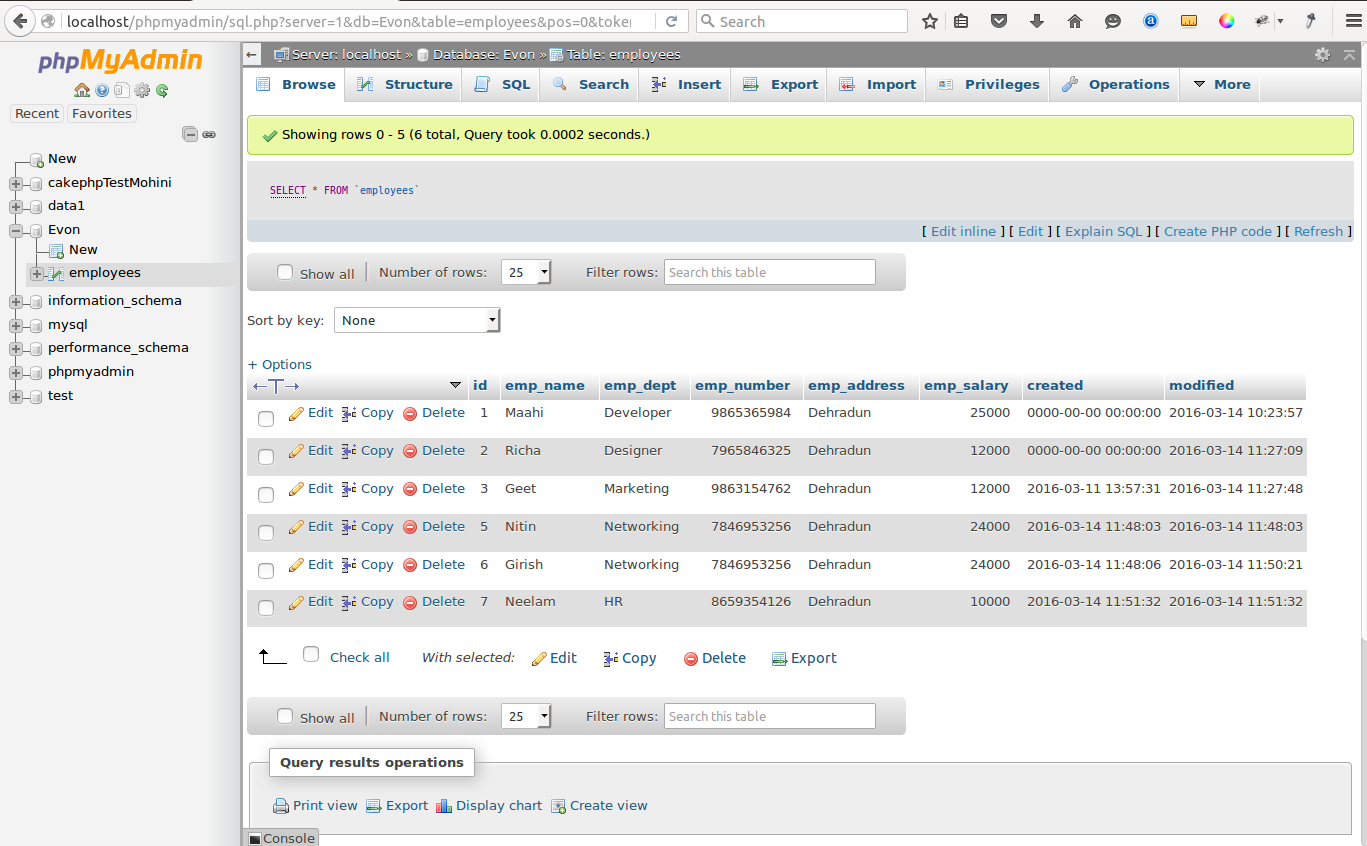
Step 2: Create Models
Now we have table in the database, next we need model file to communicate with that table and fetch results for the application. In the app/Model directory, create a new file named employee.php
<?php
App::uses('AppModel', 'Model');
class Employee extends AppModel {
public $name = 'Employee';
}
Copy and paste the above code into your employee.php file. Variable $name will be used from our Controller to access the model's functions.
Step 3: Create controllers and views for Read data
In the app/Controllers folder, create a new file for the employees table in the database. Controllers, by default, link up to the table after which they are named. In this case, we have created an employee table. So, create a file name EmployeesController.php in app/Controllers and paste this code.
<?php
App::uses('Controller', 'Controller');
class EmployeesController extends AppController {
public $components = array('Session');
public function index()
{
if ($this->request->is('post'))
{
$this->Employee->save($this->request->data);
$this->Session->setFlash('The employee details has been saved.');
}
}
}
?>
We are extending AppController class, declaring $name variable. This time I have added functions called index. The controller functions are called actions. By defining function index() in our EmployeesController, users can now access the logic there by requesting http://localhost/employees/index.
Create View File – index.ctp
In the app/View folder, Create a new folder named Employees in this folder create file index.ctp .ctp files extensions are Cakephp template files. Copy and paste this code in your index.ctp file.
<?php
echo $this->Session->flash();
echo $this->Form->create('Employee',array('controller' => 'employees','url'=>'index',));
echo $this->Form->input('emp_name',array( 'label'=>'Name','placeholder'=>'Enter your name','required'));
echo $this->Form->input('emp_dept',array('label'=>'Department','placeholder' => 'Enter your Department','required'));
echo $this->Form->input('emp_number',array('placeholder' => ''));
echo $this->Form->input('emp_address',array('placeholder' => ''));
echo $this->Form->input('emp_salary',array('placeholder' => ''));
echo $this->Form->end('SUBMIT');
//echo $this->HTML->link('display', array('url'=>'display')) ;
echo $this->Html->link(
'Display',
array(
'controller' => 'employees',
'action' => 'display',
'full_base' => true
)
);
?>
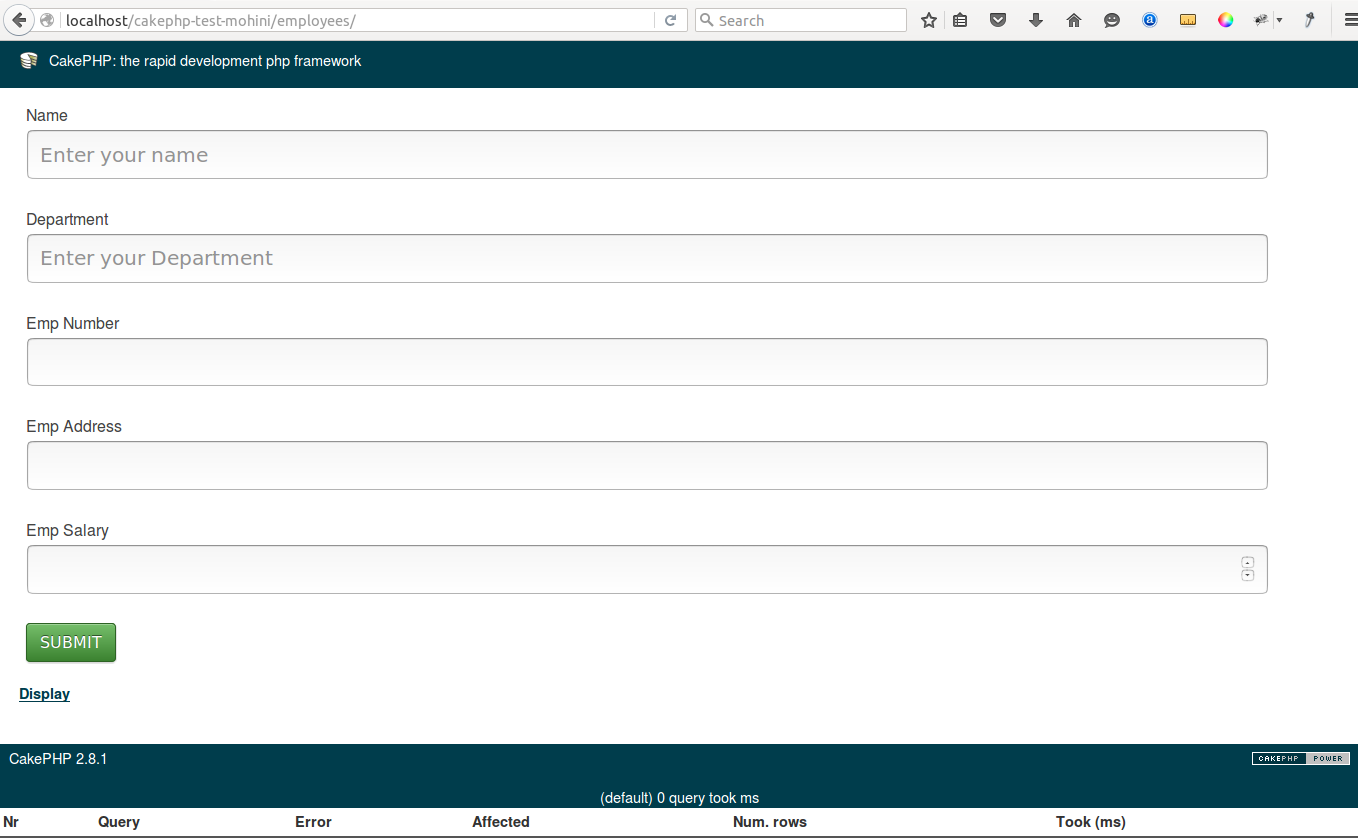
Step 4: Create controllers and views for display data
Add some more lines of code to your EmployeesController.php. Copy and paste the display() function.
public function display()
{
$All = $this->Employee->find('all');
$this->set('Employee',$All);
}
This function is used to view the contents of the table.
Create View file for display() function – display.ctp
In the app/Views/Employees folder, create a new file named display.ctp and paste the below code.
<?php echo $this->Session->flash();
?>
<h1 style="font-weight:800;font-size:28px"> Evon Employees List: </h1>
<table>
<tr>
<th>ID</th>
<th>Name</th>
<th>Department</th>
<th>Number</th>
<th>Address</th>
<th>Salary</th>
</tr>
<?php foreach($Employee as $employees): ?>
<tr>
<td><?php echo $employees['Employee']['id'];?></td>
<td><?php echo $employees['Employee']['emp_name'];?></td>
<td><?php echo $employees['Employee']['emp_dept'];?></td>
<td><?php echo $employees['Employee']['emp_number'];?></td>
<td><?php echo $employees['Employee']['emp_address'];?></td>
<td><?php echo $employees['Employee']['emp_salary'];?></td>
<td><?php echo $this->Html->link('Edit',array('action'=>'edit/'.$employees['Employee']['id']));?></td>
<td><?php echo $this->Html->link('Delete', array('action' => 'delete', $employees['Employee']['id']), null, 'Are you sure?' )?></td>
</tr>
<?php endforeach; ?>
</table>
<?php echo $this->Html->link('Add New Employee Details',array('action'=>'index')); ?>
It will look alike.
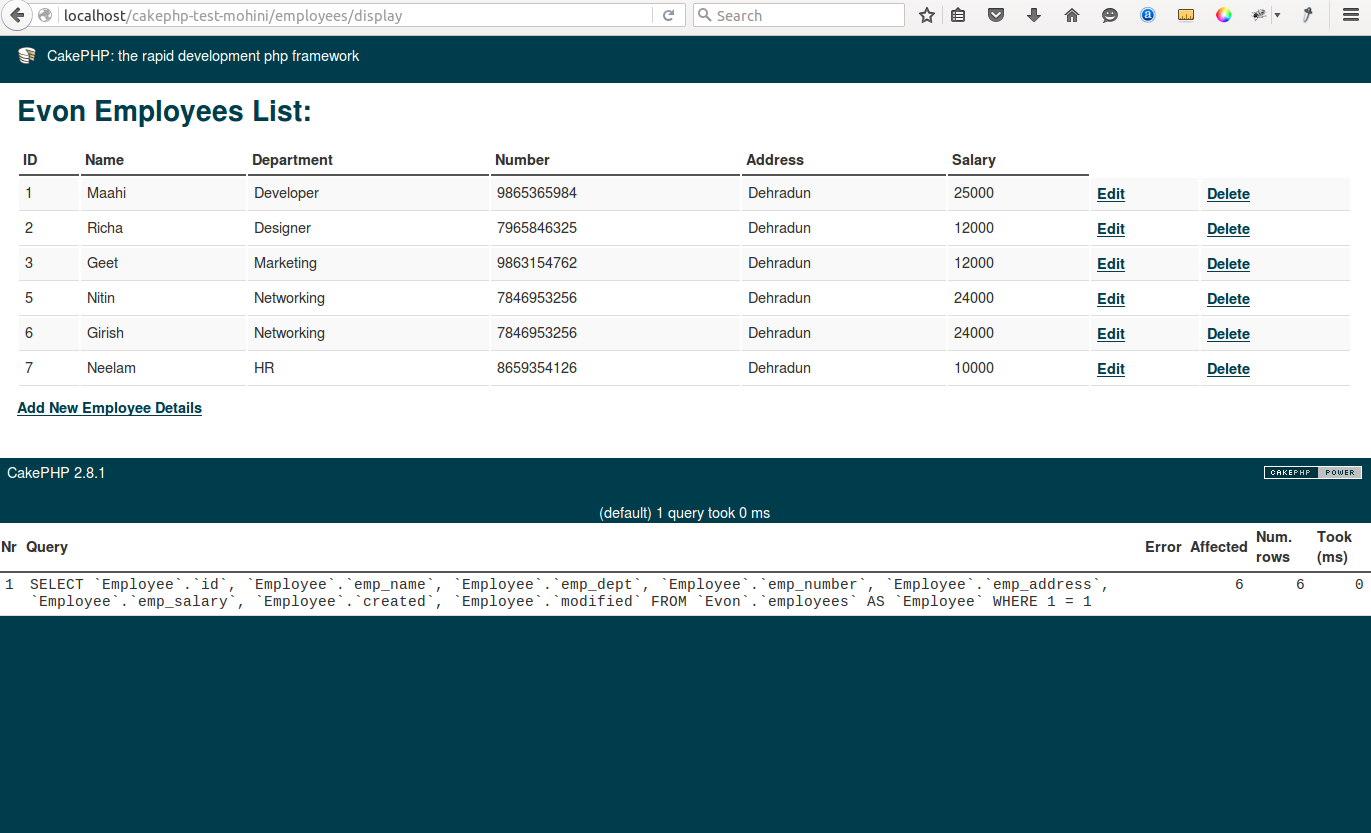
Step 5: Create controller and views for edit data
create a function for editing employees in controller. Open your /app/controllers/EmployeesController.php file and add this new function edit().
function edit($id=null)
{
if(!empty($id)){
$get_data = $this->Employee->find('first',array('conditions'=>array('Employee.id'=>$id)));
$this->set('abc',$get_data);
}
if($this->request->data){
//pr($this->request->data);die;
if($this->Employee->save($this->request->data)){
$this->Session->setFlash("Edit saved.");
$this->redirect(array('controller'=>'employees','action'=>'display'));
}
else{
$this->Session->setFlash("Wrong.");
$this->redirect(array('controller'=>'employees','action'=>'display'));
}
}
}
Create View file for edit() function – edit.ctp
create a new file named edit.ctp and copy the below code to it.
<?php //pr($abc);die;?>
<h1>Edit Profile</h1>
<?php
echo $this->Form->create('Employee',array('url'=>array('controller'=>'employees','action'=>'edit')));
echo $this->Form->input('Employee.id',array('type'=>'hidden','value'=>$abc['Employee']['id']));
echo $this->Form->input('Employee.emp_name',array('type'=>'text','placeholder'=>'Edit Your name','value'=>$abc['Employee']['emp_name']));
echo $this->Form->input('Employee.emp_dept',array('type'=>'text','placeholder'=>'Edit Department','value'=>$abc['Employee']['emp_dept']));
echo $this->Form->input('Employee.emp_number',array('type'=>'text','placeholder'=>'Edit Your number','value'=>$abc['Employee']['emp_number']));
echo $this->Form->input('Employee.emp_address',array('type'=>'text','placeholder'=>'Edit Your address','value'=>$abc['Employee']['emp_address']));
echo $this->Form->input('Employee.emp_salary',array('type'=>'text','placeholder'=>'Edit Your salary','value'=>$abc['Employee']['emp_salary']));
echo $this->Form->end('Save Changes');
?>
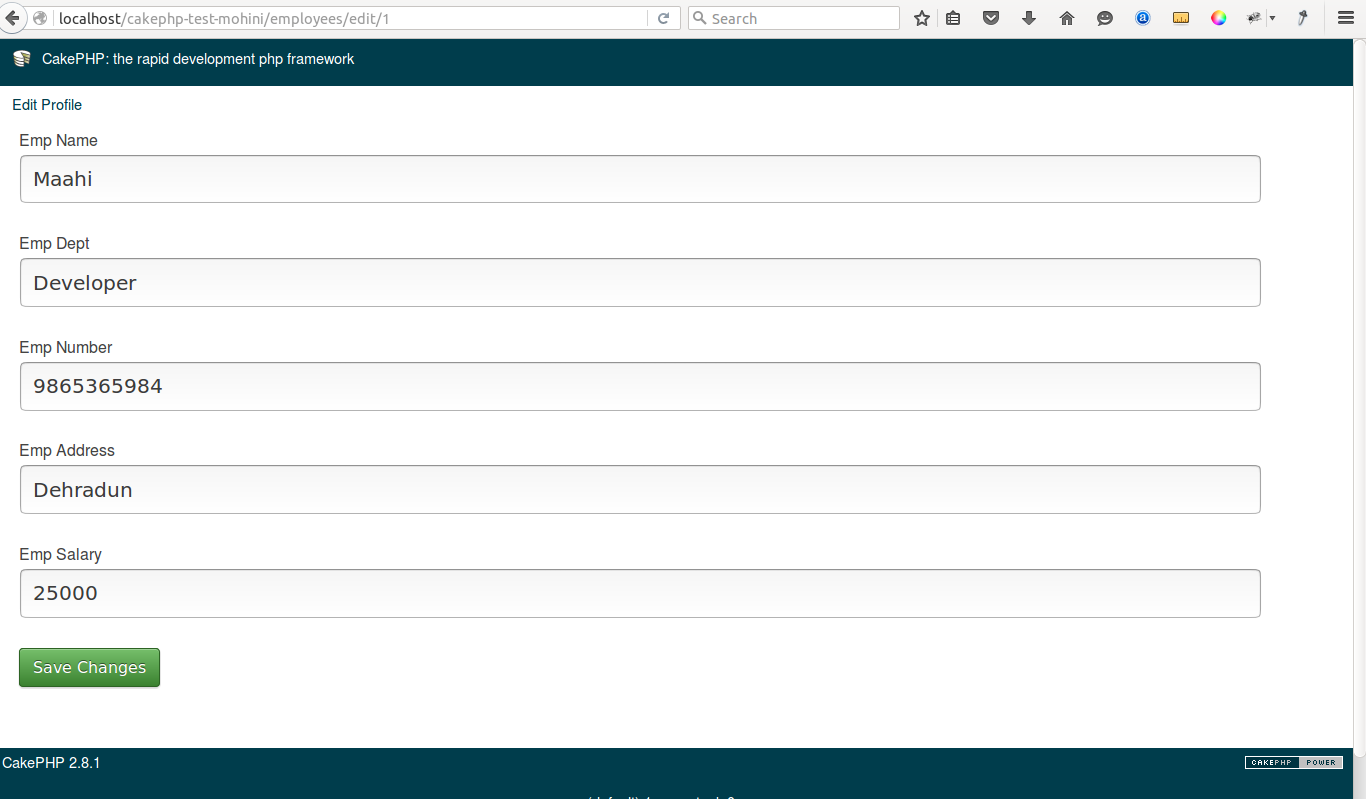
Step 6: Create controllers and views for delete data.
Create new function delete() in EmployeesController.php file
public function delete($id)
{
$this->Employee->delete($id);
$this->Session->setFlash('The employee with id: '.$id.' has been deleted.');
$this->redirect(array('action'=>'display'));
}
This function only deletes the employee data and redirects back to display action of the Employees controller, no need to create a view for this function.
0 Comment(s)