Hello Readers,
To add a toolbar above the keyboard we may use the following code snippet.
We will create a toolbar with the needed items and set as the input accessory View of the keyboard. In the below code we have added a done button on top of the keyboard, clicking on the done button dismisses the keyboard.
ObjectiveC
- (BOOL)textViewShouldBeginEditing:(UITextView *)textView{
UIToolbar *toolbar = [[UIToolbar alloc]init];
[toolbar setBarStyle:UIBarStyleDefault];
[toolbar setTranslucent:YES];
UIBarButtonItem *doneBtn = [[UIBarButtonItem alloc]initWithTitle:@"Done" style:UIBarButtonItemStyleDone target:self action:@selector(resignKeyboard)];
[toolbar setItems:@[doneBtn]];
[toolbar setUserInteractionEnabled:YES];
[toolbar sizeToFit];
[textView setDelegate:self];
[textView setInputAccessoryView:toolbar];
return YES;
}
- (void)resignKeyboard{
[self.view endEditing:YES];
}
Swift
func textViewShouldBeginEditing(textView: UITextView) -> Bool {
let keyboardToolBar = UIToolbar()
keyboardToolBar.barStyle = UIBarStyle.Default
keyboardToolBar.translucent = true;
let doneBtn = UIBarButtonItem(title: "Done", style: UIBarButtonItemStyle.Done, target:self, action: "resignKeyboard")
keyboardToolBar.setItems([doneBtn], animated: true)
keyboardToolBar.sizeToFit()
textView.inputAccessoryView = keyboardToolBar
return true;
}
func resignKeyboard(){
self.view.endEditing(true)
}
Output:
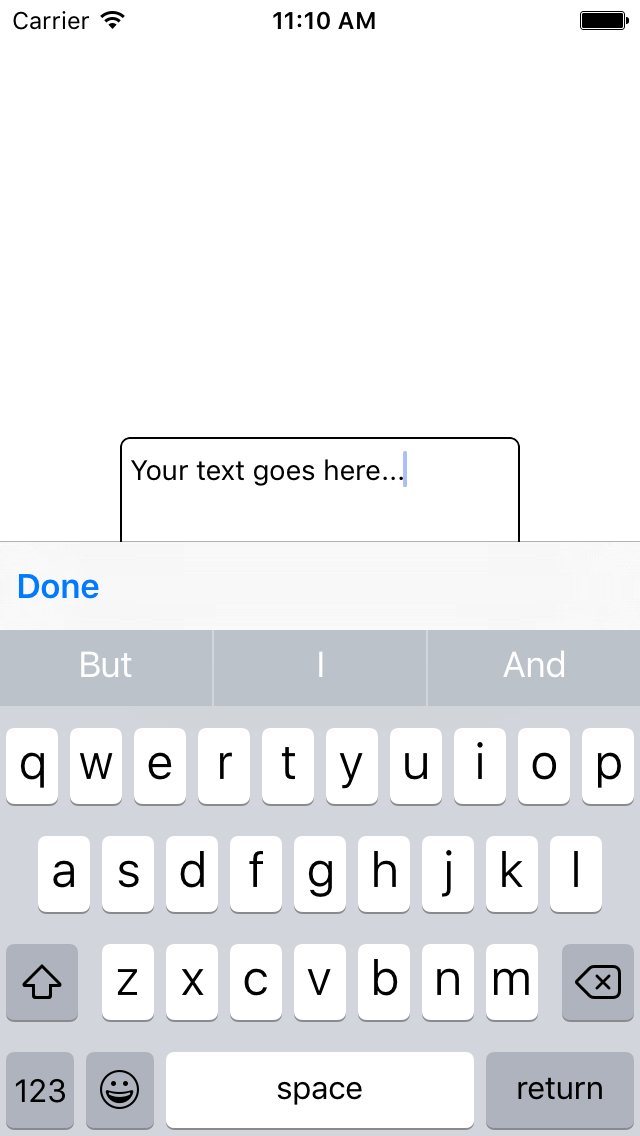
0 Comment(s)