Sometimes you need to adding dynamic fields in a form as per client requirement. The dynamic field is new input field which will be created whenever we click + button in our script. Here, in this tutorial, I have provided an easy way to add dynamic field using JQuery. I have created a script where you can add and remove multiple fields.
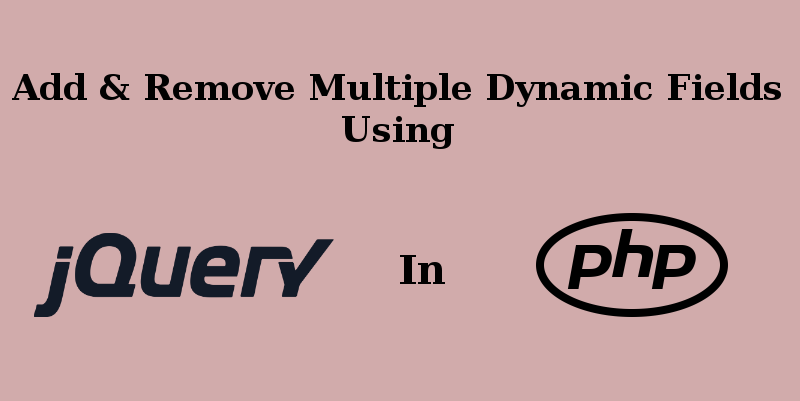
Let's suppose, you want to add 5 field dynamically. For that put maxfield variable value equal to 5.
You can also add more fields as per need. Lets see how can we do it.
JavaScript code:
First step is to include the jQuery library.
<script src="//ajax.googleapis.com/ajax/libs/jquery/1.11.2/jquery.min.js"></script>
<script type="text/javascript">
$(document).ready(function(){
var maxField = 5;
var addButton = $('.add_button');
var wrapper = $('.field_wrapper');
var fieldHTML = '<div><input type="text" name="field_name[]" value=""/><a href="javascript:void(0);" class="remove_button" title="Remove field">-</a></div>';
var x = 1;
$(addButton).click(function(){
if(x < maxField){
x++;
$(wrapper).append(fieldHTML);
}
});
$(wrapper).on('click', '.remove_button', function(e){
e.preventDefault();
$(this).parent('div').remove();
x--;
});
});
</script>
Code Explanation:
In the above code, maxField variable is set to 5 & x < maxField will check maximum number of input fields.
If x is less than maxField & add button (+) is clicked, then new input field will be append to the parent div & x will be incremented.
If remove button (-) is clicked then input field will be removed & x will be decremented.
HTML Code:
<div class="field_wrapper">
<div>
<input type="text" name="field_name[]" value=""/>
<a href="javascript:void(0);" class="add_button" title="Add field">+</a>
</div>
</div>
PHP code to get these fields value in your page.
<?php
print '<pre>';
print_r($_REQUEST['field_name']);
print '</pre>';
//output
Array
(
[0] => value1
[1] => value2
[2] => value3
[3] => value4
)
?>
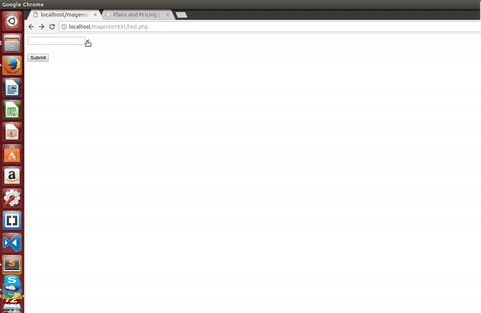
0 Comment(s)