Pointer in C are used to store the reference of the variable not its value.
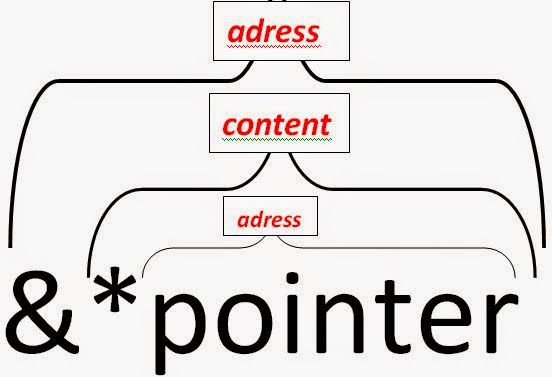
Everytime we declare a variable its reference is created in the memory somewhere and when it is accessed that variable memory address is fetched for retrieving it.
Declaring a pointer is very simple like declaring a simple variable
int *p;
int a =20;
p=&a;
#include <stdio.h>
int main () {
int var1;
char var2[10];
printf("Address of var1 variable: %x\n", &var1 );
printf("Address of var2 variable: %x\n", &var2 );
return 0;
}
Pointer is defined by its type at the time of declaration .
You will provide a data type at declaration time that makes a pointer of that kind.
int *ip; /* pointer to an integer */
double *dp; /* pointer to a double */
float *fp; /* pointer to a float */
char *ch /* pointer to a character */
#include <stdio.h>
int main () {
int var = 20; /* actual variable declaration */
int *ip; /* pointer variable declaration */
ip = &var; /* store address of var in pointer variable*/
printf("Address of var variable: %x\n", &var );
/* address stored in pointer variable */
printf("Address stored in ip variable: %x\n", ip );
/* access the value using the pointer */
printf("Value of *ip variable: %d\n", *ip );
return 0;
}
0 Comment(s)