Array is a homogenous container of items for storing and retrieving data. Most of data structure use array for its implementation. Following are important terms to understand the concepts of Array.
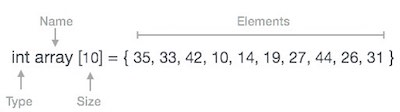
Basic Operations
Following are the basic operations supported by an array.
-
Traverse − print all the array elements one by one.
-
Insertion − add an element at given index.
-
Deletion − delete an element at given index.
-
Search − search an element using given index or by value.
-
Update − update an element at given index.
Insertion
1. Start
2. Set J=N
3. Set N = N+1
4. Repeat steps 5 and 6 while J >= K
5. Set LA[J+1] = LA[J]
6. Set J = J-1
7. Set LA[K] = ITEM
8. Stop
#include <stdio.h>
main() {
int LA[] = {1,3,5,7,8};
int item = 10, k = 3, n = 5;
int i = 0, j = n;
printf("The original array elements are :\n");
for(i = 0; i<n; i++) {
printf("LA[%d] = %d \n", i, LA[i]);
}
n = n + 1;
while( j >= k){
LA[j+1] = LA[j];
j = j - 1;
}
LA[k] = item;
printf("The array elements after insertion :\n");
for(i = 0; i<n; i++) {
printf("LA[%d] = %d \n", i, LA[i]);
}
}
0 Comment(s)