The C Preprocessor not the compiler part, but it executes as compilation occurs.
C Preprocessor is just a text substitution tool that tells the compiler to do required pre-processing before the actual compilation.
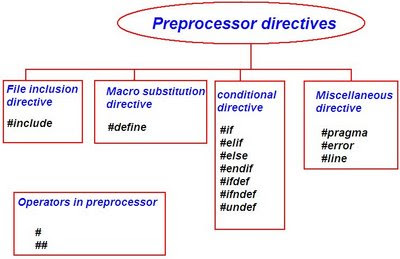
#define MAX_ARRAY_LENGTH 20
This will tell the compiler to replace Max_Array_length with 20.
#include <stdio.h>
#include "myheader.h"
The first line will include file from global library and from the set of header file defined for C compiler.
The second line will tell the compiler to include local library file from the location where header files are placed.
#ifndef MESSAGE
#define MESSAGE "You wish!"
#endif
This is simple conditional statement that is used to do operation or display message based on some condition.
Predefined Macros
Macros are the group of statements that are used when we want to use it again and again.
Macro |
Description |
__DATE__ |
The current date as a character literal in "MMM DD YYYY" format. |
__TIME__ |
The current time as a character literal in "HH:MM:SS" format. |
__FILE__ |
This contains the current filename as a string literal. |
__LINE__ |
This contains the current line number as a decimal constant. |
__STDC__ |
Defined as 1 when the compiler complies with the ANSI standard. |
#include <stdio.h>
main() {
printf("File :%s\n", __FILE__ );
printf("Date :%s\n", __DATE__ );
printf("Time :%s\n", __TIME__ );
printf("Line :%d\n", __LINE__ );
printf("ANSI :%d\n", __STDC__ );
}
0 Comment(s)