View Encapsulation is a way that defines whether components styles will affect the whole application or not i.e it has control over usage of component styles, use styles globally or should be limited to a particular Angular component (scoped styles).
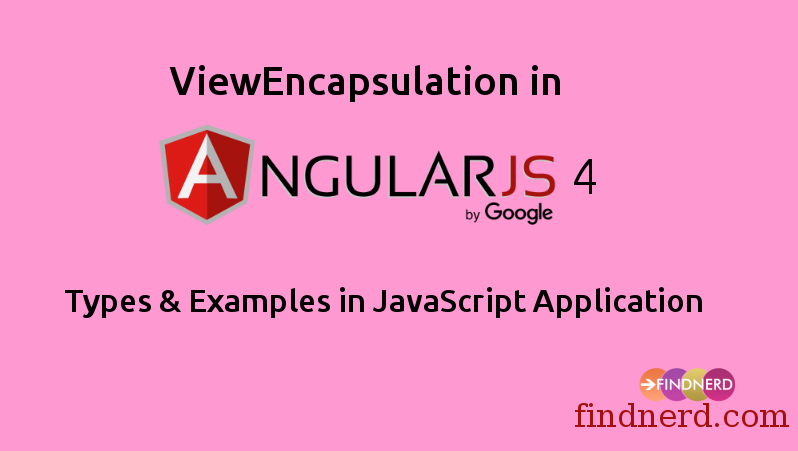
Angular Components can be styled via inline styles through styles property or via external styles through styleUrls which are the property of @Component decorator.
Example
In default root component "app.component.ts" adding style
- Inline styles through styles property.
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styles: [
`h1 {
color: #369;
font-family: Arial, Helvetica, sans-serif;
font-size: 250%;
}
`]
})
export class AppComponent {
title = 'Angular 4 app';
}
- External styles through styleUrls property.
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
title = 'Angular 4 app';
}
Above mentioned component styles are appended to document head. But by using native Shadow DOM the component styles will be included in component’s template.
What is Shadow DOM?
Shadow DOM can be defined as Web Components standard part which is capable of enabling DOM tree and style encapsulation i.e it allows elements in the DOM to have scoped styles without letting styles to leak outside of component scope.
Shadow DOM in Angular
In Angular, components are built. The component can be defined as a controller class in possession of template and styles. Components are shareable i.e reusable across the application. But these angular components are not web components but they can take advantage of or say are inspired by web components. Angular components can have scoped styles which are controlled through encapsulation property.
View Encapsulation Types
There are three view encapsulation types:
- ViewEncapsulation.None
- ViewEncapsulation.Emulated
- ViewEncapsulation.Native
Whenever an Angular component is created, Angular puts the component template inside shadowRoot, which can be considered as shadow DOM of that particular component. Thus doing this DOM tree and style encapsulation can be obtained but what if the browser does not have shadow DOM. In such cases, Angular does not create shadowRoot for component until native shadow DOM i.e "ViewEncapsulation.Native" is used. In fact, Angular uses "ViewEncapsulation.Emulated" by default.
Example of View Encapsulation Types
1) ViewEncapsulation.None: Shadow DOM is not supported at all, therefore, no style encapsulation. Therefore whatever styles applied to an angular component are kept within document head.
Create a new component student using "ng g component student" command.
student.component.ts
import { Component, OnInit } from '@angular/core';
import {ViewEncapsulation} from '@angular/core';
@Component({
selector: 'app-student',
templateUrl: './student.component.html',
styles: [
`h1 {
color: #367;
font-family: Arial, Helvetica, sans-serif;
font-size: 250%;
}
`],
encapsulation: ViewEncapsulation.None
})
export class StudentComponent implements OnInit {
constructor() { }
ngOnInit() {
}
}
student.component.html (student template)
<h1>Inside student Component</h1>
Root component "app.component.ts"
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
template: '<app-student></app-student>'
})
export class AppComponent {
title = 'Angular 4 app';
}
The page "index.html" which is rendered:
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>AngularDemo</title>
<base href="/">
<meta name="viewport" content="width=device-width, initial-scale=1">
<link rel="icon" type="image/x-icon" href="favicon.ico">
</head>
<body>
<h1>Welcome to Angular 4 Demo</h1>
<app-root></app-root>
</body>
</html>
When this application runs it will create following DOM
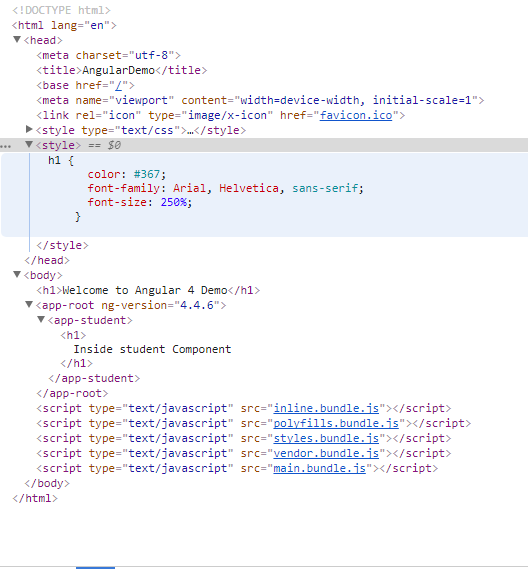
It can be seen that no shadow DOM is created as well as student component style are kept within document head. Therefore style for the h1 element is not scoped and thereby applied to entire application i.e for student template as well as for index.html.
Output
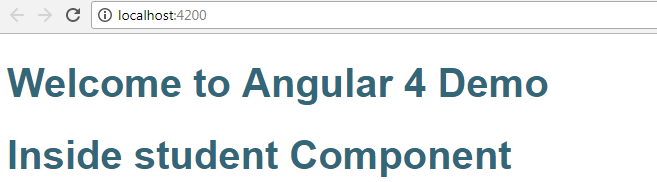
ViewEncapsulation.Emulated
Does not support Shadow DOM but support style encapsulation. It is the default value of angular components styles.
Change encapsulation type in "student.component.ts" to "ViewEncapsulation.Emulated" rest remain same.
@Component({
selector: 'app-student',
templateUrl: './student.component.html',
styles: [
`h1 {
color: #367;
font-family: Arial, Helvetica, sans-serif;
font-size: 250%;
}
`],
encapsulation: ViewEncapsulation.Emulated
})
export class StudentComponent implements OnInit {
constructor() { }
ngOnInit() {
}
}
When this application runs it will create following DOM
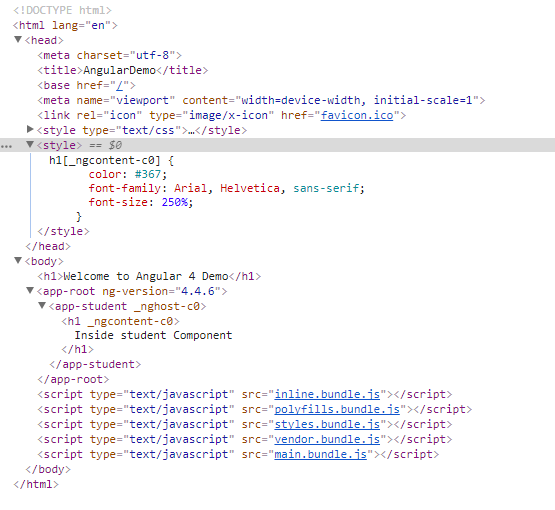
It can be seen that no Shadow DOM is created and student component style is kept within document head. But Angular created h1[_ngcontent-c0] selector changing component's style. For scoped styles, Angular extended CSS selector's so that they have higher specificity and collision with other same selectors before they do not happen as a result component’s style selectors only match required particular component and nothing else. To match these selectors template elements are also extended.
Output
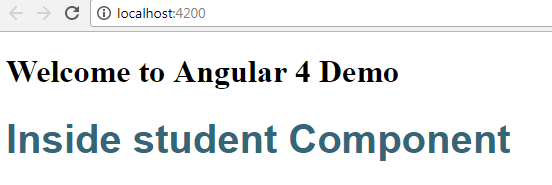
The styling for h1 element is only applied to student component template.
ViewEncapsulation.Native
It not only supports Shadow DOM but also supports style encapsulation.
Change encapsulation type in "student.component.ts" to "ViewEncapsulation.Native" rest remain same.
import { Component, OnInit } from '@angular/core';
import {ViewEncapsulation} from '@angular/core';
@Component({
selector: 'app-student',
templateUrl: './student.component.html',
styles: [
`h1 {
color: #367;
font-family: Arial, Helvetica, sans-serif;
font-size: 250%;
}
`],
encapsulation: ViewEncapsulation.Native
})
export class StudentComponent implements OnInit {
constructor() { }
ngOnInit() {
}
}
When this code runs in browser create following DOM
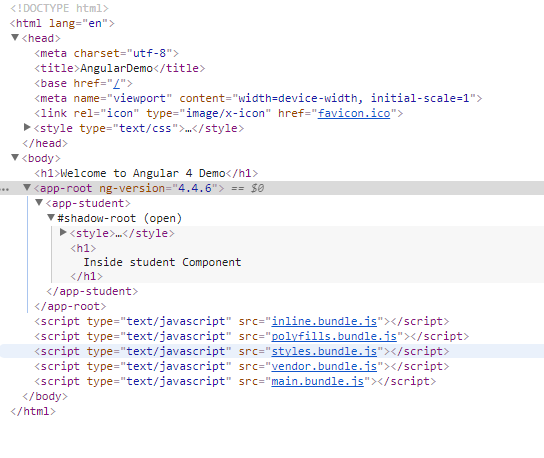
It can be seen that student component style are not within document head instead it appears within shadowRoot in the component template.
0 Comment(s)