This is a basic how-to tutorial on adding single or multiple images to PDF using JSPDF framework. JSPDF framework is a framework which helps to convert an html document into PDF format. To achieve this, we need to first download JSPDF framework.
Here, we need to first convert the image into image data and then initialize the JSPDF framework. (Javascript PDF) is the client side solution for generating PDFs. JSPDF is helpful for event ticket, reports and certificated. In order to use JSPDF you need to include its library.
So, today in this tutorial, I am going to tell you how to add single or multiple image(s) to pdf using JSPDF
. Write the following javascript to add images to pdf.
You might also be interested in a tutorial which explains How to Create PDF from HTML using JSPDF. Hope this tutorial will help you also.
Step 1: Include the javascript files in the header before running the code.
<header>
<script src="https://code.jquery.com/jquery-3.1.1.min.js"></script>
<script type="text/javascript" src="jspdf.js"></script>
</header>
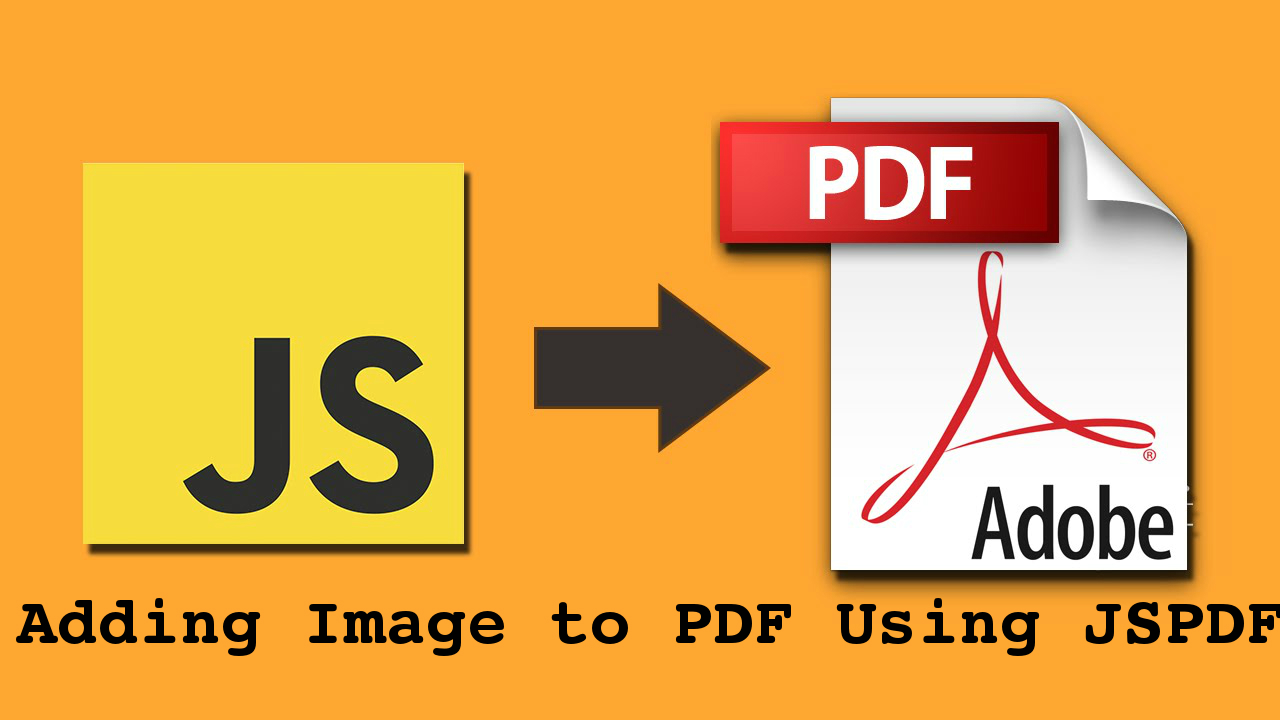
Step 2: Write the following code to add images to pdf file
<script type="text/javascript">
$(document).ready(function() {
var getImageFromUrl = function(url, callback) {
var img = new Image();
img.onError = function() {
alert('Cannot load image: "'+url+'"');
};
img.onload = function() {
callback(img);
};
img.src = url;
}
var createPDF = function(imgData) {
var doc = new jsPDF('p', 'pt', 'a4');
var width = doc.internal.pageSize.width;
var height = doc.internal.pageSize.height;
var options = {
pagesplit: true
};
doc.text(10, 20, 'Crazy Monkey');
var h1=50;
var aspectwidth1= (height-h1)*(9/16);
doc.addImage(imgData, 'JPEG', 10, h1, aspectwidth1, (height-h1), 'monkey');
doc.addPage();
doc.text(10, 20, 'Hello World');
var h2=30;
var aspectwidth2= (height-h2)*(9/16);
doc.addImage(imgData, 'JPEG', 10, h2, aspectwidth2, (height-h2), 'monkey');
doc.output('datauri');
}
getImageFromUrl('thinking-monkey.jpg', createPDF);
});
</script>
You can download jspdf.js file by clicking on this link: jspdf.js .
About the code:
1) addImage: addImage will write image to pdf and convert images to Base64. Following parameters are required to add an image.
- imageData: Pass image
- format: extension of image
- x-axis: position of image from left
- y-axis: position of image from top
- width: width of image
- height: height of image
- alias: Name the image alias which is comfortable for you.
Syntax: doc.addImage(imgData, 'image format', x, y, w, h, 'alias');
2) addPage: To add a new page to PDF, addPage is used.
3) output: Output will generate the PDF document.
4) doc.internal.pageSize.width: Get width of pdf
5) doc.internal.pageSize.height: Get height of pdf
After executing the code on the browser you can see the following output on the browser. Image has been added to pdf.
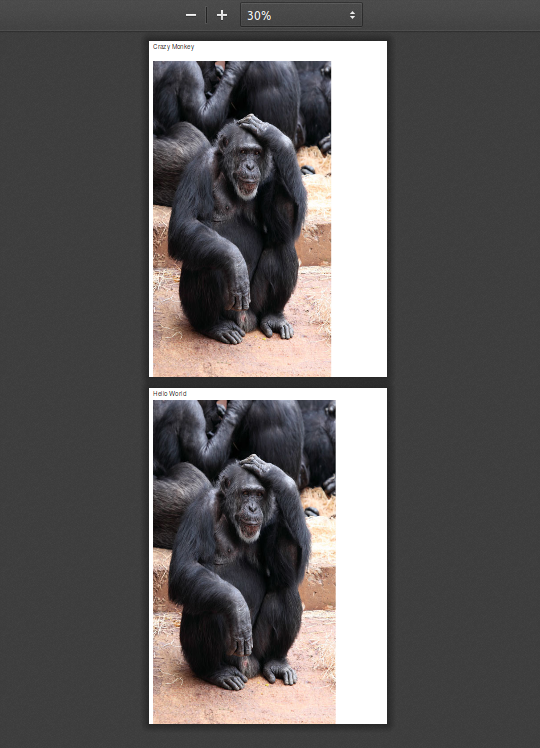
All done!
Thanks for reading the blog.
1 Comment(s)