Hash Map is a type of Array list containing special key for every element within it, while Shared Preference is being used to save data like String, Boolean, Integer and other primitive data type.
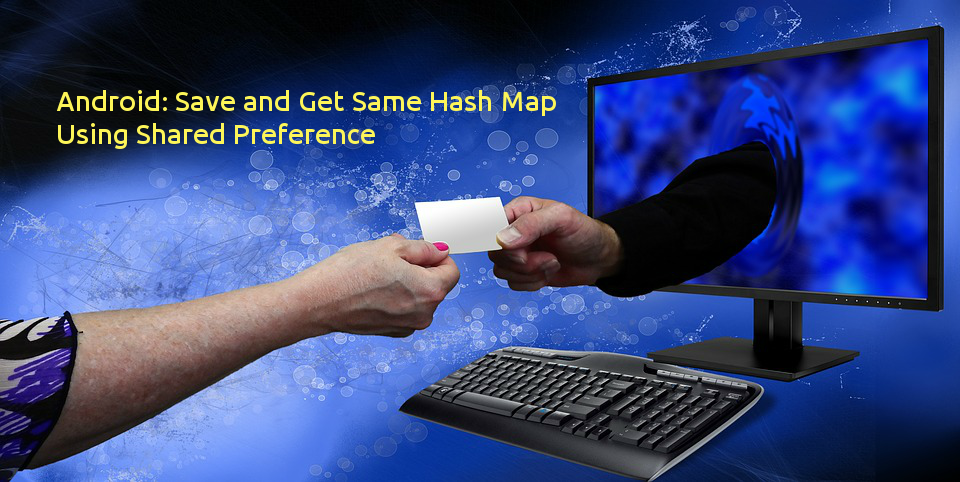
So, in this tutorial, I'm saving the Hash Map in Shared Preference by making use of Gson. Let us see how to do that:
Gson first convert the Hash Map to Jason and then to a simple String then this string is being saved to Shared Preference.
Add the following code snippet to your sharedPreference class
//To save Hash Map
public static void saveMap(Context context,String key, Map<Double,AlarmDataBean> inputMap){
SharedPreferences pSharedPref = context.getSharedPreferences(USERDATA, Context.MODE_PRIVATE);
if (pSharedPref != null){
Gson gson = new Gson();
String hashMapString = gson.toJson(inputMap);
//save in shared prefs
pSharedPref.edit().putString(key, hashMapString).apply();
}
}
Using above code, we are saving the Hash Map in SharedPreference, we are passing three parameters to the method, first one is the context, second one is the unique Key of String type and third one is the Hash Map that we are going to save to our SharedPreference. The AlarmDataBean is a simple Bean class getter setter class.
Now to get the same hash map add following code snippet to your sharedPreference class
public static Map<Double,AlarmDataBean> loadMap(String key,Context context){
Map<Double,AlarmDataBean> outputMap = new HashMap<Double,AlarmDataBean>();
SharedPreferences pSharedPref = context.getSharedPreferences(USERDATA, Context.MODE_PRIVATE);
try{
//get from shared prefs
String storedHashMapString = pSharedPref.getString(key, (new JSONObject()).toString());
java.lang.reflect.Type type = new TypeToken<HashMap<Double, AlarmDataBean>>(){}.getType();
Gson gson = new Gson();
return gson.fromJson(storedHashMapString, type);
}catch(Exception e){
e.printStackTrace();
}
return outputMap;
}
In the above code, we are loading the Hash Map from the SharedPreference, here two parameters have been send, the first one is the String type that is key witch is unique and the second one is context. We first take out the String formatted data from the sharedpreference then convert it into json and then defines the Hash Map type and when all this process was done, we returns the obtained Hash Map to the place from where this method is being called.
0 Comment(s)