RecyclerView is works just like listview but more well organised, felexible to customize and optimize to work and in rendering the view of large data set. So in this tutorial we will see how to add items Dynamically in RecyclerView.
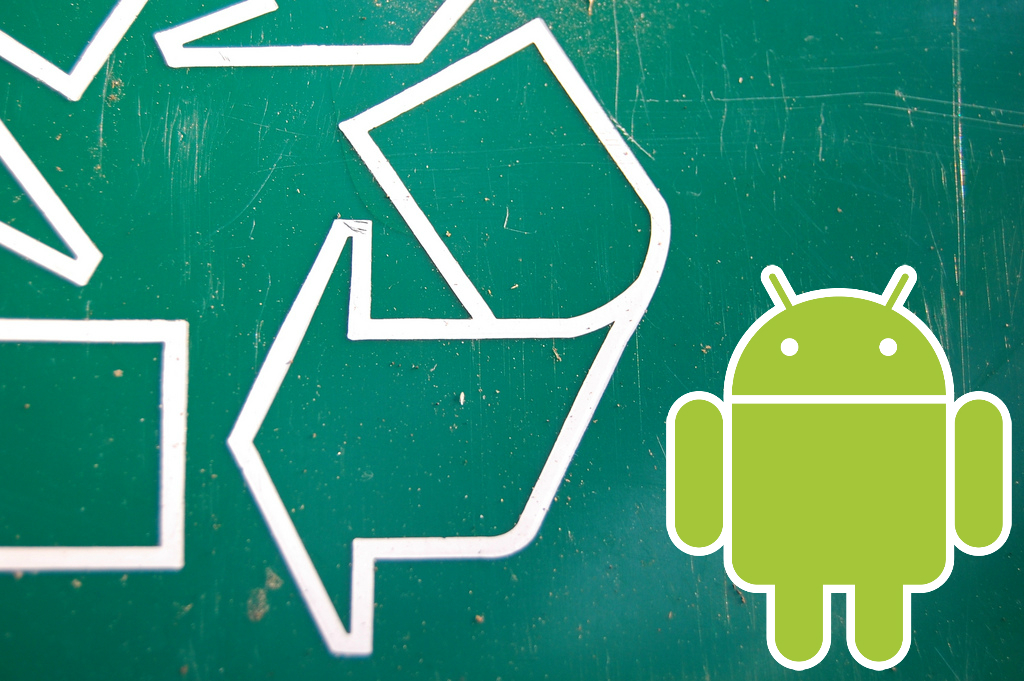
For that first, I have created recycler view in which items are added and removed Dynamically. In this app we can add items in Recyclerview and when we click on the item in RecyclerView it will be deleted based on the positon of the item.
Step(1)-I have added first RecyclerView, CardView and Design dependency to Grandle file.
compile 'com.android.support:design:23.1.1'
compile 'com.android.support:recyclerview-v7:21.0.+'
compile 'com.android.support:cardview-v7:21.0.+'
Step(2)-activity_main xml.layout-
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:padding="16dp"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context=".MainActivity">
<EditText
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="Title"
android:id="@+id/etTitle" />
<EditText
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="Description"
android:id="@+id/etDescription" />
<Button
android:id="@+id/btnAddItem"
android:text="Add Item"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
<android.support.v7.widget.RecyclerView
android:id="@+id/recycler_view"
android:scrollbars="vertical"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="#dbfffe" />
</LinearLayout>
Step(3)-Created a new row.xml layout-
<android.support.v7.widget.CardView xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="horizontal"
android:layout_width="match_parent"
android:layout_margin="8dp"
android:id="@+id/card_view"
android:layout_height="match_parent">
<LinearLayout
android:orientation="vertical"
android:id="@+id/mainLayout"
android:layout_width="match_parent"
android:layout_height="wrap_content">
<TextView
android:id="@+id/txtTitle"
android:padding="12dp"
android:layout_width="match_parent"
android:textColor="@android:color/black"
android:layout_height="wrap_content" />
<TextView
android:id="@+id/txtDescription"
android:padding="12dp"
android:layout_width="match_parent"
android:textColor="@android:color/black"
android:layout_height="wrap_content" />
<ImageView
android:id="@+id/crossImage"
android:layout_width="65dp"
android:layout_height="65dp"
android:visibility="visible"
android:layout_marginLeft="285dp"
android:paddingLeft="10dp"
android:background="@drawable/cross" />
</LinearLayout>
</android.support.v7.widget.CardView>
Step(4)MainActivity-
public class MainActivity extends Activity implements RemoveClickListner{
private RecyclerView mRecyclerView;
private RecyclerAdapter mRecyclerAdapter;
private RecyclerView.LayoutManager mLayoutManager;
Button btnAddItem;
ArrayList<RecyclerData> myList = new ArrayList<>();
EditText etTitle, etDescription;
String title = "",description = "";
ImageView crossImage;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
mRecyclerView = (RecyclerView) findViewById(R.id.recycler_view);
mRecyclerAdapter = new RecyclerAdapter(myList,this);
final LinearLayoutManager layoutManager = new LinearLayoutManager(this);
layoutManager.setOrientation(LinearLayoutManager.VERTICAL);
mRecyclerView.setLayoutManager(layoutManager);
mRecyclerView.setAdapter(mRecyclerAdapter);
etTitle = (EditText) findViewById(R.id.etTitle);
etDescription = (EditText) findViewById(R.id.etDescription);
btnAddItem = (Button) findViewById(R.id.btnAddItem);
btnAddItem.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
title = etTitle.getText().toString();
description = etDescription.getText().toString();
if (title.matches("")) {
Toast.makeText(v.getContext(), "You did not enter a Title", Toast.LENGTH_SHORT).show();
return;
}
if (description.matches("")) {
Toast.makeText(v.getContext(), "You did not enter a description", Toast.LENGTH_SHORT).show();
return;
}
RecyclerData mLog = new RecyclerData();
mLog.setTitle(title);
mLog.setDescription(description);
myList.add(mLog);
mRecyclerAdapter.notifyData(myList);
etTitle.setText("");
etDescription.setText("");
}
});
}
@Override
public void OnRemoveClick(int index) {
myList.remove(index);
mRecyclerAdapter.notifyData(myList);
}
}
Step(5)-Created a new RecyclerData class
public class RecyclerData {
String title;
String description;
RecyclerView data;
public String getTitle() {
return title;
}
public void setTitle(String title) {
this.title = title;
}
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public void setCrossImage(ImageView crossImage){
setCrossImage(crossImage);
}
}
Step(6)-Created a new RecyclerAdapter class-
public class RecyclerAdapter extends RecyclerView.Adapter<RecyclerAdapter.RecyclerItemViewHolder> {
private ArrayList<RecyclerData> myList;
int mLastPosition = 0;
private RemoveClickListner mListner;
public RecyclerAdapter(ArrayList<RecyclerData> myList,RemoveClickListner listner) {
this.myList = myList;
mListner=listner;
}
public RecyclerItemViewHolder onCreateViewHolder(ViewGroup parent, int viewType) {
View view = LayoutInflater.from(parent.getContext()).inflate(R.layout.row, parent, false);
RecyclerItemViewHolder holder = new RecyclerItemViewHolder(view);
return holder;
}
@Override
public void onBindViewHolder(RecyclerItemViewHolder holder, final int position) {
Log.d("onBindViewHoler ", myList.size() + "");
holder.etTitleTextView.setText(myList.get(position).getTitle());
holder.etDescriptionTextView.setText(myList.get(position).getDescription());
holder.crossImage.setImageResource(R.drawable.cross);
mLastPosition =position;
}
@Override
public int getItemCount() {
return(null != myList?myList.size():0);
}
public void notifyData(ArrayList<RecyclerData> myList) {
Log.d("notifyData ", myList.size() + "");
this.myList = myList;
notifyDataSetChanged();
}
public class RecyclerItemViewHolder extends RecyclerView.ViewHolder {
private final TextView etTitleTextView;
private final TextView etDescriptionTextView;
private LinearLayout mainLayout;
public ImageView crossImage;
public RecyclerItemViewHolder(final View parent) {
super(parent);
etTitleTextView = (TextView) parent.findViewById(R.id.txtTitle);
etDescriptionTextView = (TextView) parent.findViewById(R.id.txtDescription);
crossImage = (ImageView) parent.findViewById(R.id.crossImage);
mainLayout = (LinearLayout) parent.findViewById(R.id.mainLayout);
mainLayout.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Toast.makeText(itemView.getContext(), "Position:" + Integer.toString(getPosition()), Toast.LENGTH_SHORT).show();
}
});
crossImage.setOnClickListener(new AdapterView.OnClickListener() {
@Override
public void onClick(View view) {
mListner.OnRemoveClick(getAdapterPosition()
);
}
});
}
}
}
Step(7)-Created a RemoveClickListner interface -
public interface RemoveClickListner {
void OnRemoveClick(int index);
}
6 Comment(s)