This tutorial will help user to create basic "Registration Form" using JavaScript. Here we will learn
creating HTML elements such as form, label, input etc. using JavaScript, setting attributes
for HTML elements, and inserting the elements into the DOM (Document Object Model) as per requirement.The use of an id-selector is done for mapping created form to the required position in the page.A Jquery datepicker widget is used to make the registration form more user friendly.
- The HTML file we used is jscriptform.html:
<html>
<head>
<title>Register</title>
<script type="text/javascript" src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.3/jquery.min.js"></script>
<script src="//code.jquery.com/ui/1.11.4/jquery-ui.js"></script>
<link rel="stylesheet" href="//code.jquery.com/ui/1.11.4/themes/smoothness/jquery-ui.css">
<!--This line includes the .css file-->
<link href="http://localhost/register/formjscript.css" rel="stylesheet">
</head>
<body>
<div id="main">
<h2>Registration Form using JavaScript</h2>
<div id="form_sample">
</div> <!--This line includes the .js file-->
<script src="http://localhost/register/formjscript.js"></script>
</div>
</body>
</html>
- The JavaScript file we used is formjscript.js:
var x = document.getElementById("form_sample");
var createform = document.createElement('form'); // Create New Element Form
createform.setAttribute("action", ""); // Setting Action Attribute on Form
createform.setAttribute("method", "post"); // Setting Method Attribute on Form
x.appendChild(createform);
var heading = document.createElement('h2'); // Heading of Form
heading.innerHTML = "Registration Form ";
createform.appendChild(heading);
var line = document.createElement('hr'); // Giving Horizontal Row After Heading
createform.appendChild(line);
var linebreak = document.createElement('br');
createform.appendChild(linebreak);
var namelabel = document.createElement('label'); // Create Label for Name Field
namelabel.innerHTML = "Your Name : "; // Set Field Labels
createform.appendChild(namelabel);
var inputelement = document.createElement('input'); // Create Input Field for Name
inputelement.setAttribute("type", "text");
inputelement.setAttribute("name", "dname");
createform.appendChild(inputelement);
var linebreak = document.createElement('br');
createform.appendChild(linebreak);
var usernamelabel = document.createElement('label'); // Create Label for Address Field
usernamelabel.innerHTML = "User Name : "; // Set Field Labels
createform.appendChild(usernamelabel);
var inputelement = document.createElement('input'); // Create Input Field for Name
inputelement.setAttribute("type", "text");
inputelement.setAttribute("name", "usrname");
createform.appendChild(inputelement);
var linebreak = document.createElement('br');
createform.appendChild(linebreak);
var pswdlabel = document.createElement('label'); // Create Label for Password Field
pswdlabel.innerHTML = "Password : "; // Set Field Labels
createform.appendChild(pswdlabel);
var inputelement = document.createElement('input'); // Create Input Field for Password
inputelement.setAttribute("type", "password");
inputelement.setAttribute("name", "psswd");
createform.appendChild(inputelement);
var linebreak = document.createElement('br');
createform.appendChild(linebreak);
var doblabel = document.createElement('label'); // Create Label for date of birth
doblabel.innerHTML = "Your Date of Birth : "; // Set Field Labels
createform.appendChild(doblabel);
var inputelement = document.createElement('input'); // Create Input Field for date of birth
inputelement.setAttribute("type", "text");
inputelement.setAttribute("name", "dob");
createform.appendChild(inputelement);
//code for date picker:
$(inputelement).datepicker({minDate: new Date(),
beforeShow: function(input, inst)
{
inst.dpDiv.css({marginTop: -input.offsetHeight + 'px', marginLeft: input.offsetWidth + 'px'});
}
});
var linebreak = document.createElement('br');
createform.appendChild(linebreak);
var contactlabel = document.createElement('label'); // Create Label for contact number
contactlabel.innerHTML = "Your contact number : "; // Set Field Labels
createform.appendChild(contactlabel);
var inputelement = document.createElement('input'); // Create Input Field for contact number
inputelement.setAttribute("type", "text");
inputelement.setAttribute("name", "contno");
createform.appendChild(inputelement);
var linebreak = document.createElement('br');
createform.appendChild(linebreak);
var emaillabel = document.createElement('label'); // Create Label for E-mail Field
emaillabel.innerHTML = "Your Email : ";
createform.appendChild(emaillabel);
var emailelement = document.createElement('input'); // Create Input Field for E-mail
emailelement.setAttribute("type", "text");
emailelement.setAttribute("name", "demail");
createform.appendChild(emailelement);
var emailbreak = document.createElement('br');
createform.appendChild(emailbreak);
var addresslabel = document.createElement('label'); // Append Textarea
addresslabel.innerHTML = "Your Address : ";
createform.appendChild(addresslabel);
var texareaelement = document.createElement('textarea');
texareaelement.setAttribute("name", "daddress");
createform.appendChild(texareaelement);
var messagebreak = document.createElement('br');
createform.appendChild(messagebreak);
var submitelement = document.createElement('input'); // Append Submit Button
submitelement.setAttribute("type", "submit");
submitelement.setAttribute("name", "dsubmit");
submitelement.setAttribute("value", "Submit");
createform.appendChild(submitelement);
- The CSS file we used is formjscript.css:
@import "http://fonts.googleapis.com/css?family=Ubuntu";
div#main{
width:430px;
height:550px;
margin:0 auto;
font-family:'Ubuntu',sans-serif
}
div#form_sample{
text-align:center;
border:3px solid #ccc;
width:300px;
padding:0 50px 15px;
margin-top:20px;
box-shadow:0 0 15px;
border-radius:6px;
float:left
}
#main h1{
margin-top:40px
}
hr{
margin-top:-5px
}
label{
float:left;
font-size:16px
}
input[type="text"]{
width:100%;
margin-top:5px;
margin-bottom:10px;
padding:10px;
border:3px solid #2BC1F2
}
input[type="password"]{
width:100%;
margin-top:1px;
margin-bottom:15px;
padding:10px;
border:3px solid #2BC1F2
}
textarea{
width:100%;
border:3px solid #2BC1F2;
padding:10px;
margin-bottom:25px;
margin-top:10px;
height:100px;
resize:none
}
input[type="submit"]{
width:100%;
padding:10px 45px;
background-color:#2BC1F2;
border:none;
color:#fff;
font-size:18px;
font-weight:700;
cursor:pointer;
font-family:'Ubuntu',sans-serif
}
- For more information use the link below:
http://www.formget.com/javascript-contact-form/
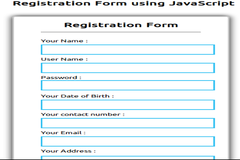
0 Comment(s)