In Angular Router is like an object that can be used in our app component to describe the routes we want to use.
In Angular, there are three components that can be used to configure routing:
* Routes: It describes routes of the application
* RouterOutlet: It is a “placeholder” component that gets expanded to each route’s content
* RouterLink: It is used to get link to the routes
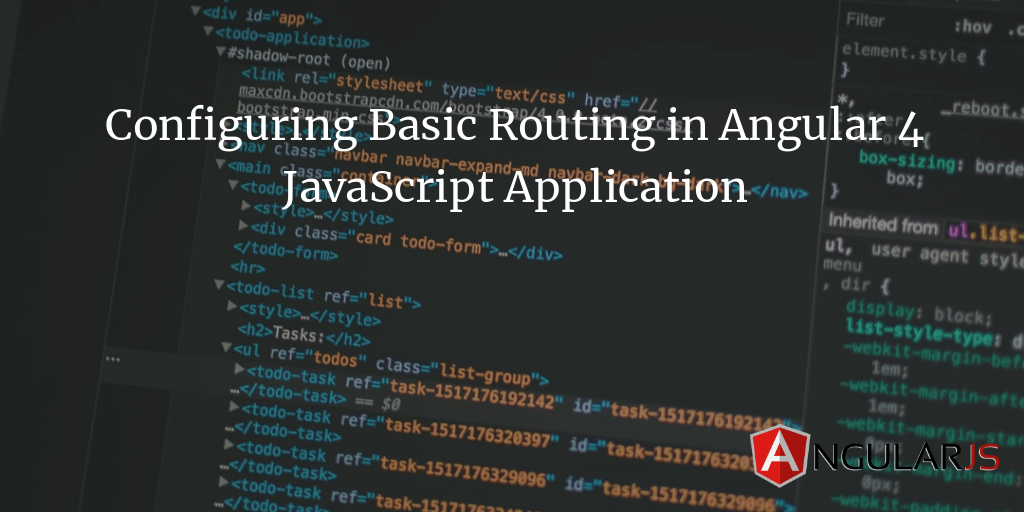
For example:
const routes: Routes = [
{ path: '', redirectTo: login, pathMatch: 'full' },
{ path: dashboard, component: DashboardComponent },
{ path: edit-profile, component: EditProfileComponent },
{ path: settings, component: SettingsComponent }
];
here we have used:
* path to give a specific URL.
* component in which we want to go for that path.
* we can redirect using the redirectTo option
Providing Router:
To install router in the application we use the RouterModule.forRoot() function in the imports key of our NgModule:
@NgModule({
declarations: [
RoutesDemoApp,
HomeComponent,
LoginComponentComponent,
DashboardComponent
],
imports: [
BrowserModule,
RouterModule.forRoot(routes) // <-- installs Router routes, components and services
],
bootstrap: [ RoutesDemoApp ],
providers: [
provide(LocationStrategy, {useClass: HashLocationStrategy})
]
})
class RoutesDemoAppModule {}
// bootstrap our app
platformBrowserDynamic().bootstrapModule(RoutesDemoAppModule)
.catch((err: any) => console.error(err));
It's important to see here that our routes serve as the argument to RouterModule.forRoot(). By putting RouterModule in our imports, we ensure the utilization of the RouterOutlet and RouterLink components in this module.
The RouterOutlet directive tells router where to render the content in the view.
For example, if we have a view:
@View({
template: `
<div>
Stuff in the outer template here
<router-outlet></router-outlet>
</div>
`
})
RouterLink:
For navigating between routes, we need to use RouterLink directive. For example, if we want to perform the task of linking to our login and dashboard page from a navigation, it becomes possible to change our view something like showed below.
@View({
template: `
<div>
<nav>
<a>Navigation:</a>
<ul>
<li><a [router-link]="['home']">Home</a></li>
<li><a [router-link]="['login']">Login</a></li>
<li><a [router-link]="['dashboard']">Dashboard</a></li>
</ul>
</nav>
<router-outlet></router-outlet>
</div>
`
})
Locating the imports:
To find all of the constants we used above, we use the following:
import {
NgModule,
provide,
Component
} from '@angular/core';
import {BrowserModule} from '@angular/platform-browser';
import {platformBrowserDynamic} from '@angular/platform-browser-dynamic';
import {
RouterModule,
Routes,
} from '@angular/router';
import {LocationStrategy, HashLocationStrategy} from '@angular/common';
0 Comment(s)