To capture a signature, a custom view is created. This view works like a signature pad and you can get the signature drawn over the view by calling getImage() method.
The image is retrieved in bitmap format and can be further processed like storing or converting it into the file.
Here is the code to create a signature view:
public class SignatureView extends View {
private final String TAG = "SignatureView";
private Path mPath;
private Paint mPaint;
// private Paint bgPaint = new Paint(Color.RED);
private Bitmap mBitmap;
private Canvas mCanvas;
private boolean isSignatureEmpty = true;
private float curX, curY;
private static final int TOUCH_TOLERANCE = 0;
private static final int STROKE_WIDTH = 4;
private int defaultCanvasColor = Color.WHITE;
private int defaultSignatureColor= Color.BLACK;
public SignatureView(Context context) {
super(context);
init();
}
public SignatureView(Context context, AttributeSet attrs) {
super(context, attrs);
init();
}
public SignatureView(Context context, AttributeSet attrs, int defStyle) {
super(context, attrs, defStyle);
init();
}
private void init() {
setFocusable(true);
mPath = new Path();
mPaint = new Paint(Paint.ANTI_ALIAS_FLAG);
mPaint.setColor(defaultSignatureColor);
mPaint.setStyle(Paint.Style.STROKE);
mPaint.setStrokeWidth(STROKE_WIDTH);
}
public void setSigColor(int color) {
mPaint.setColor(color);
}
public void setSigColor(int a, int red, int green, int blue) {
mPaint.setARGB(a, red, green, blue);
}
public boolean clearSignature() {
isSignatureEmpty = true;
if (mCanvas != null) {
mCanvas.drawColor(defaultCanvasColor);
mPath.reset();
invalidate();
}
else {
Bitmap newBitmap = Bitmap.createBitmap( getWidth(),getHeight(),Bitmap.Config.ARGB_4444);
Canvas newCanvas = new Canvas();
newCanvas.setBitmap(newBitmap);
if (mBitmap != null)
newCanvas.drawBitmap(mBitmap, 0, 0, null);
mBitmap = newBitmap;
mCanvas = newCanvas;
mCanvas.drawColor(defaultCanvasColor);
mPath.reset();
invalidate();
return false;
}
return true;
}
public Bitmap getImage() {
return this.mBitmap;
}
public boolean isSignatureEmpty() {
Log.d(TAG, "isSignatureEmpty: "+isSignatureEmpty);
return isSignatureEmpty;
}
public void setImage(Bitmap bitmap) {
this.mBitmap = bitmap;
isSignatureEmpty = false;
this.invalidate();
}
@Override
protected void onSizeChanged(int width, int height, int oldWidth, int oldHeight) {
int bitmapWidth = mBitmap != null ? mBitmap.getWidth() : 0;
int bitmapHeight = mBitmap != null ? mBitmap.getWidth() : 0;
if (bitmapWidth >= width && bitmapHeight >= height)
return;
if (bitmapWidth < width)
bitmapWidth = width;
if (bitmapHeight < height)
bitmapHeight = height;
Bitmap newBitmap = Bitmap.createBitmap(bitmapWidth, bitmapHeight, Bitmap.Config.ARGB_4444);
Canvas newCanvas = new Canvas();
newCanvas.setBitmap(newBitmap);
if (mBitmap != null)
newCanvas.drawBitmap(mBitmap, 0, 0, null);
mBitmap = newBitmap;
mCanvas = newCanvas;
mCanvas.drawColor(defaultCanvasColor);
}
@Override
protected void onDraw(Canvas canvas) {
System.out.print("onDraw is called");
canvas.drawColor(defaultCanvasColor);
canvas.drawBitmap(mBitmap, 0, 0, mPaint);
canvas.drawPath(mPath, mPaint);
}
@Override
public boolean onTouchEvent(MotionEvent event) {
float x = event.getX();
float y = event.getY();
switch (event.getAction()) {
case MotionEvent.ACTION_DOWN:
touchDown(x, y);
break;
case MotionEvent.ACTION_MOVE:
touchMove(x, y);
break;
case MotionEvent.ACTION_UP:
touchUp();
break;
}
invalidate();
return true;
}
private void touchDown(float x, float y) {
mPath.reset();
mPath.moveTo(x, y);;
curX = x;
curY = y;
touchMove(x,y);
}
private void touchMove(float x, float y) {
x=x+1;
y=y+1;
float dx = Math.abs(x - curX);
float dy = Math.abs(y - curY);
if (dx >= TOUCH_TOLERANCE || dy >= TOUCH_TOLERANCE) {
mPath.quadTo(curX, curY, (x + curX)/2, (y + curY)/2);
curX = x;
curY = y;
}
}
private void touchUp() {
mPath.lineTo(curX, curY);
if (mCanvas == null) {
mCanvas = new Canvas();
mCanvas.setBitmap(mBitmap);
}
mCanvas.drawPath(mPath, mPaint);
mPath.reset();
isSignatureEmpty = false;
}
}
And paste this code in your xml file:
<SignatureView
android:id="@+id/signatureView"
android:layout_width="match_parent"
android:layout_height="match_parent"
/>
You can call clearSignature() method to clear a signature and isSignatureEmpty() method to determine whether the signature is drawn on the view or not.
Here is the screens of the project that I have made:
Click on the box to open a Signature Box (SognatureFragment)
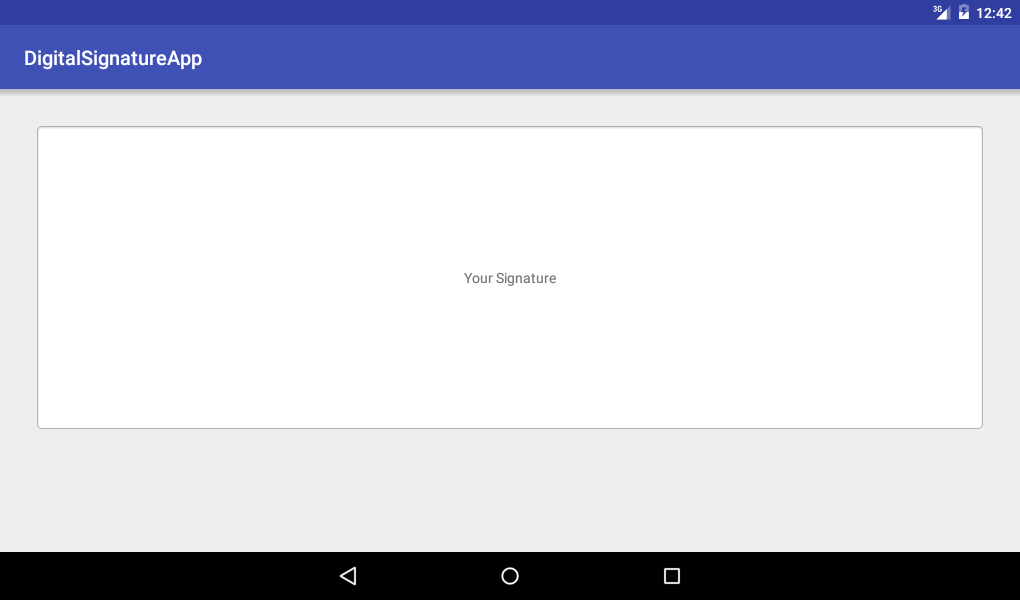
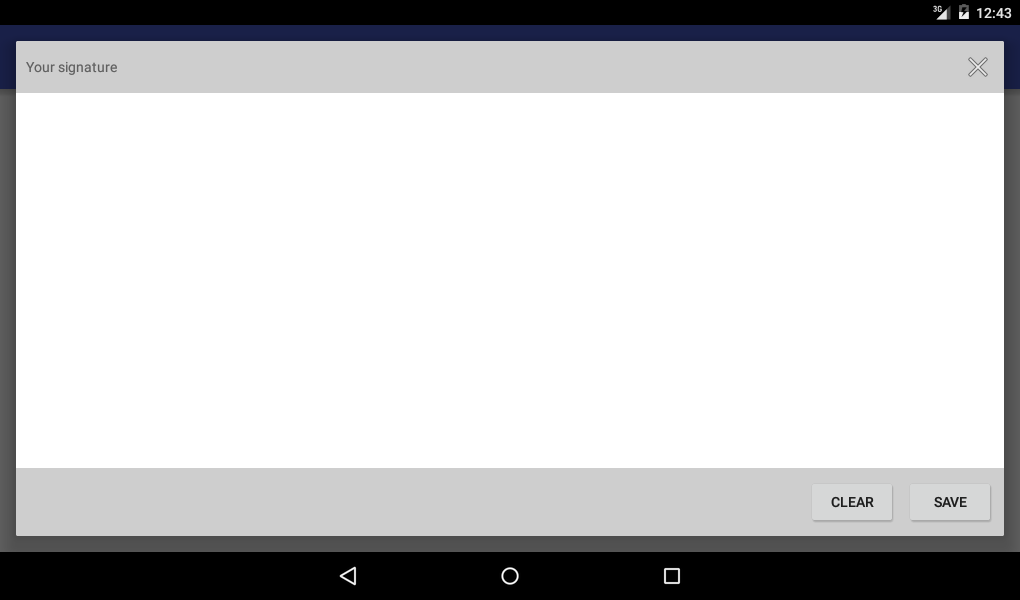
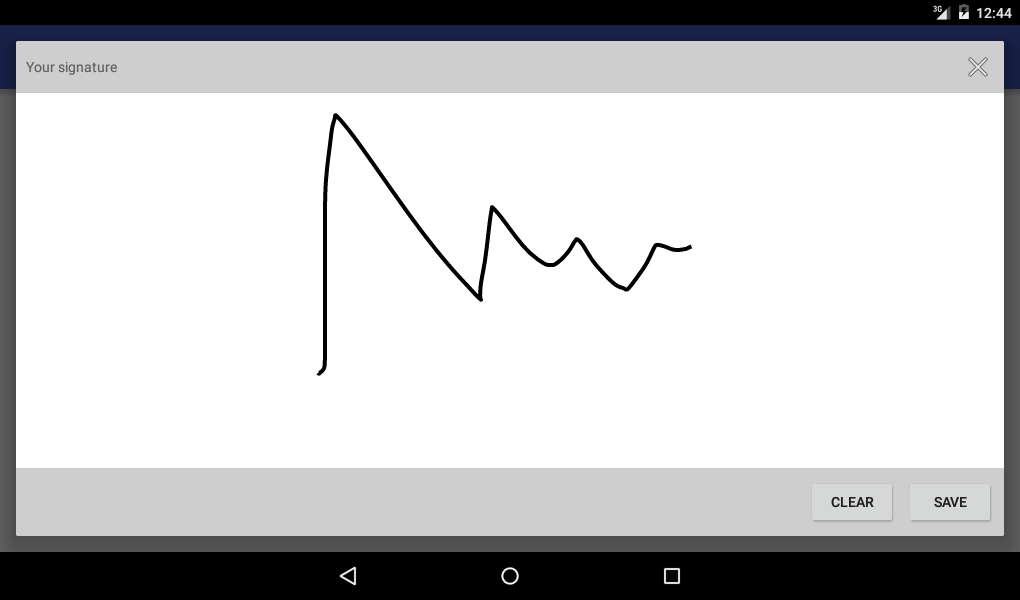
Click save to get the Bitmap and pase it into ImageView inside Activity
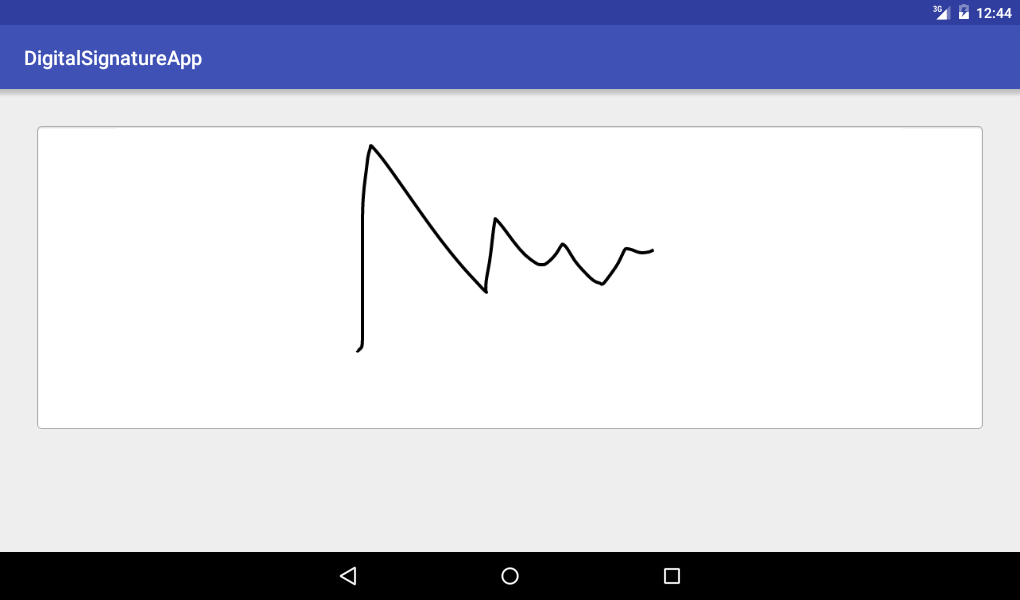
You can download the project from the file attached.
0 Comment(s)