Hi friends, this blog is targeting those readers who are keen to know about a very common method of partial updating specific region in asp.net page without reloading the whole page again and again, it is also meant for targeting readers who are just beginning their journey in this huge and exciting world of programming and have little or no knowledge about partial-page updates in asp.net applications.
Prerequisites:
Visual Studio or Visual Web Developer Express
To have a better understanding for the need of UpdatePanel control in ASP.NET let's go through some scenarios first.
Scenario 1:
Understanding why we need UpdatePanel control
To make this point more practical, let's start with an example.
Suppose we are using an application (not using any partial updates) where we have a form to add details. There are different sections in the form like Personal Information, Work Experience, Activities and so on. We are assuming the form to be lengthy and need mouse scroll to check all the sections. Okay so we have scrolled our page and at the last we find following sections.
Personal Information:
......
......
|
Qualification:
......
......
|
Work Experience:
......
......
|
and so on.
Now as you can see, we have a section named as "Qualification" in between "Personal Information" and "Work Experience", where user needs to add his/her educational qualifications using a text box (to enter qualification name like 10th, 12th and so on.). There is also a button with text "Add Qualification" that will add the provided qualification name in the Grid just below it.
In following screenshot we have already added "10 standard" in the below grid.

Now we have entered a new qualification "BCA" as shown in above screenshot and click on "Add Qualification" button, whole page suddenly postback and we are taken to the beginning of the form. Is it OK, when we still have 2 or 3 other qualifications to be added in that section or we have to continue with next section?
Well I am assuming it's NO for sure, from your side. Well then what should be the behavior of that form. If you have some idea that's great but if you are not sure then don't worry as we are about to get into it.
For the above issue we would need some sort of solution where instead of posting the whole page data to server, we could have posted data for "Qualification" section only, in other words instead of updating whole page we could have partially updated the "Qualification" section only. If this can be done then while adding qualification as we did earlier we wouldn't get full postback but will be viewing the same section after clicking "Add Qualification", results in adding the entered qualification getting added in the following grid.
Obviously it will enhance user experience and will also reduce the amount of data transfer between server and client. So the main idea is that we will encapsulate the region with UpdatePanel control, which needs to be partially updated.
So now it's time to look at a practical example, let's move on to next section which will describe the practical implementation.
Scenario 2:
Adding an UpdatePanel control in asp.net web site
Let's open up Visual Studio installed version. Select File -> New -> Project. (For this tutorial, I am using Visual Studio 2015)
Now you will get New Project window, where you can give name to your Project and select location where you want to create this project.
Note: Please make sure "Web" is selected under "Visual C#" which is further categorized under "Templates" category on the left side.
New Project Window
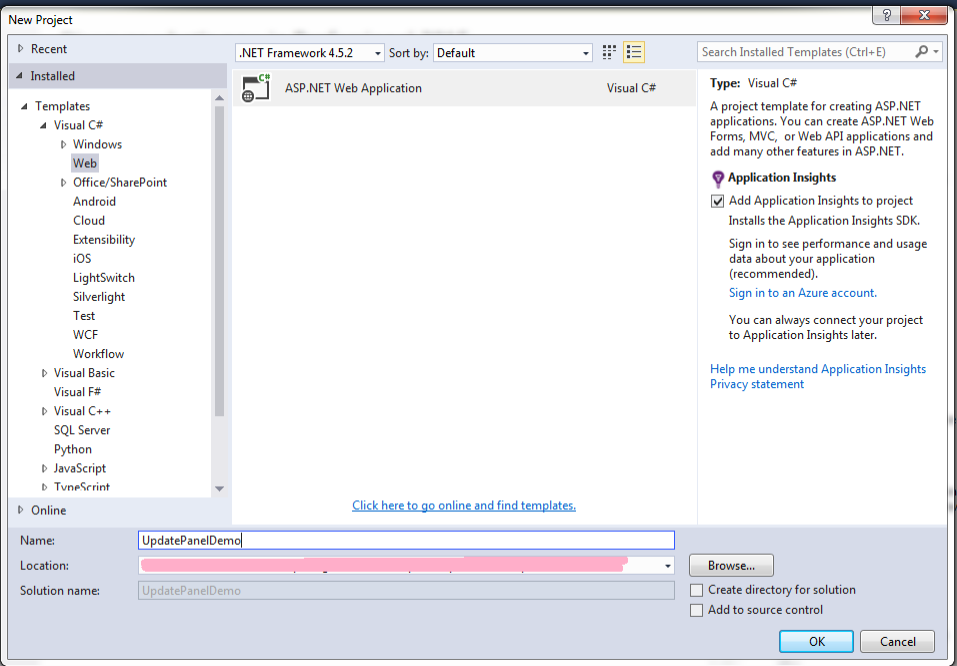
For our example I have named project as "UpdatePanelDemo".
Click on OK button and you will be presented with a new pop up window where you can select from the available templates. To keep things very simple let's select "Empty" template.
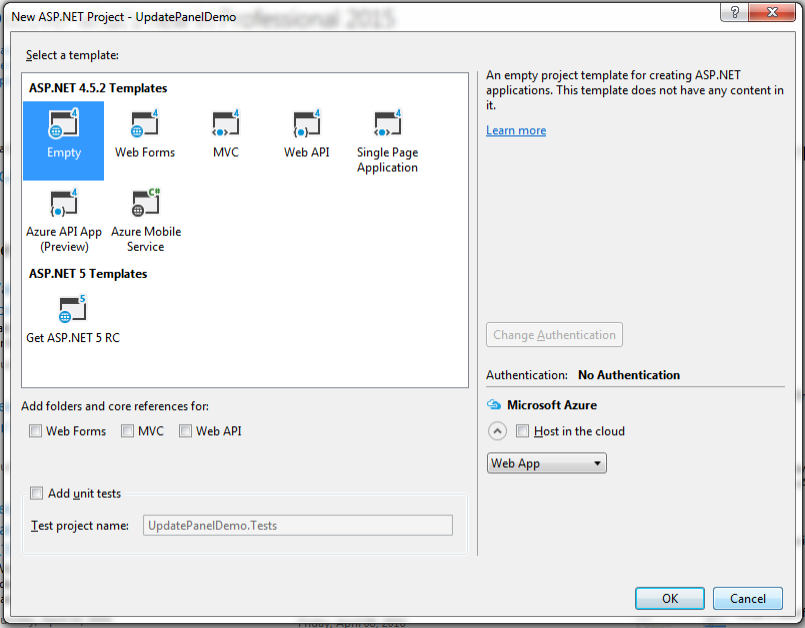
Clicking OK button will create a new project for you. Remember we had selected "Empty" template for this project so you will only get necessary file structure where you will add required components manually as and when required.
To implement our example we would need a web form that will get rendered in browser. So let's add a new web form which will be used to show how to add UpdatePanel in web page. Right click on the project in Solution Explorer on right side, select Add -> New Item.
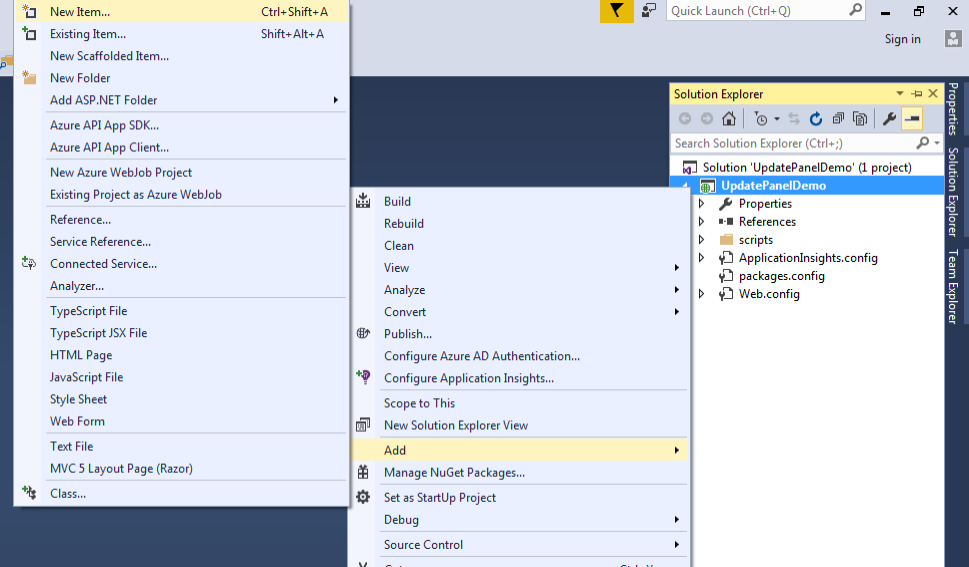
Select New Item and you will get Add New Item window as shown below. Give it a name as Default.aspx and click on Add.
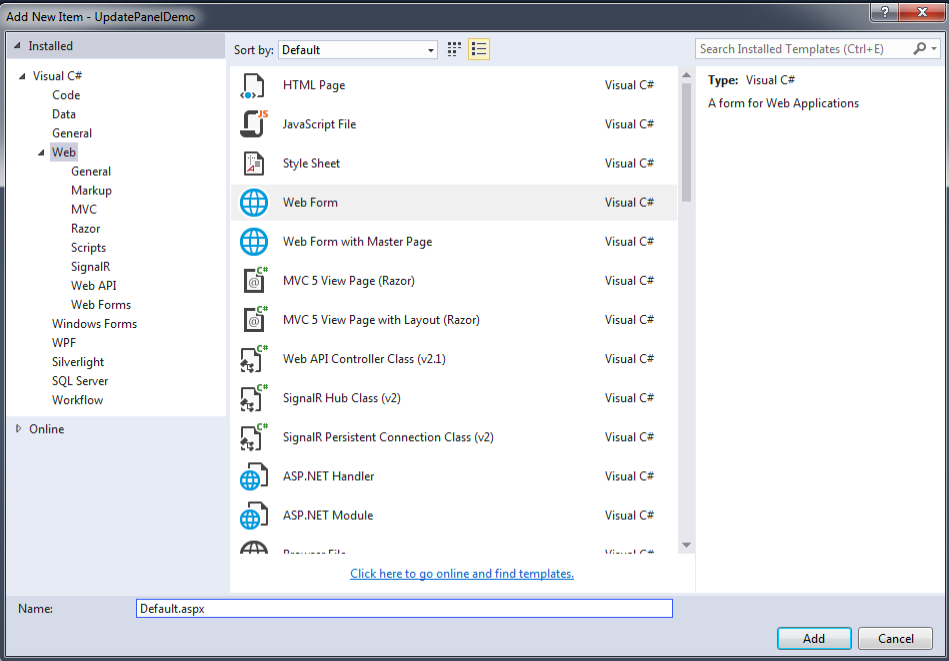
Now change the Default.aspx file with following markup
<%@ Page Language="C#" AutoEventWireup="true" CodeBehind="Default.aspx.cs" Inherits="UpdatePanelDemo.Default" %>
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title></title>
</head>
<body>
<form id="form1" runat="server">
<div>
DateTime1:
<asp:Label ID="lblDateTime1" runat="server"></asp:Label>
</div>
<div>
DateTime2:
<asp:Label ID="lblDateTime2" runat="server"></asp:Label>
</div>
<div>
<asp:Button ID="btnGetCurrentDateTime" runat="server"
Text="Get Current DateTime"
OnClick="btnGetDateTime_Click" />
</div>
</form>
</body>
</html>
And change the Default.aspx.cs content with following code
using System;
namespace UpdatePanelDemo
{
public partial class Default : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
lblDateTime1.Text = DateTime.Now.ToString();
lblDateTime2.Text = DateTime.Now.ToString();
}
protected void btnGetDateTime_Click(object sender, EventArgs e)
{
lblDateTime2.Text = DateTime.Now.ToString();
}
}
}
You must be thinking what's our motive by applying above code and markup?
Let me answer this so obvious question. We actually want to check the behaviour of the page when we are not using any partial updates. After getting the initial information of this behaviour, we will compare it with the behavior when partial updates will be implemented using UpdatePanel control.
We already know that first time application will run, the Page_Load event gets called which will initialize both labels (lblDateTime1 and lblDateTime2) with the current date and time value. That's fine as we have coded it in such manner. You'll see labels initialized with current date time values as following:
Screen after application run for the first time

Now let's click on "Get Current DateTime" button and see what happens. Click on button and you will notice that the whole page post back and both labels get refreshed with the current date time value.
Screen after we click on the "Get Current DateTime" button

Sequence in which label updates after clicking a button:
Sequence 1:
Inside page load event
- DateTime1 gets current date time
- DateTime2 gets current date time
Sequence 2:
Inside button click event
- DateTime2 gets current date time
Here we want to set both labels with current date time value for the first time application get started and want to update only "DateTime2" label after clicking on "Get Current DateTime" button. One thing to note down here is that every time button is clicked Page_Load event gets called before calling btnGetDateTime_Click event of button hence resulting in updation of both labels with current date time value. Hence finally we didn't achieve what we aimed for.
Let's try with following updates now which used partial rendering concept and see if we get the expected result.
Replace Default.aspx file content with following markup
<%@ Page Language="C#" AutoEventWireup="true" CodeBehind="Default.aspx.cs" Inherits="UpdatePanelDemo.Default" %>
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title></title>
</head>
<body>
<form id="form1" runat="server">
<asp:ScriptManager ID="ScriptManager1" runat="server"></asp:ScriptManager>
<div>
DateTime1:
<asp:Label ID="lblDateTime1" runat="server"></asp:Label>
</div>
<asp:UpdatePanel ID="UpdatePanel1" runat="server">
<ContentTemplate>
<div>
DateTime2:
<asp:Label ID="lblDateTime2" runat="server"></asp:Label>
</div>
<br />
<div>
<asp:Button ID="btnGetCurrentDateTime" runat="server"
Text="Get Current DateTime"
OnClick="btnGetDateTime_Click" />
</div>
</ContentTemplate>
</asp:UpdatePanel>
</form>
</body>
</html>
Note: No change needed in Default.aspx.cs file.
Now let's discuss these changes that are added to get an idea about partial updates using UpdatePanel.
ScriptManager control
ScriptManager control is available in Visual Studio toolbox window under AJAX Extensions category. ScriptManager control registers and provide support for client side AJAX features available in Microsoft AJAX Library. Once AJAX features from Microsoft AJAX Library are available in web page, we can take advantage of partial page rendering and other ajax related features. It must be placed inside form tag whenever we will use UpdatePanel control.
UpdatePanel control
UpdatePanel control is also available in Visual Studio toolbox under AJAX Extensions category.
It helps in building web applications with rich client side experience. It allows us to enclosed area inside UpdatePanel control that needs to be partially updated. By default whenver form is posted by any control inside UpdatePanel then only enclosed region gets updated asynchronously.
Let's run our application with these updated changes and see what we get now.
Like before when first time application run, the Page_Load event gets called which will initialize both labels with the current date and time value.
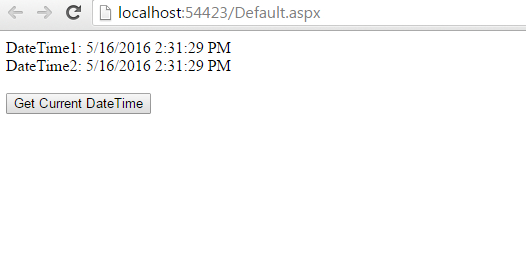
Now click on the "Get Current DateTime" button and you'll notice that page doesn't post back like before, also instead of updating both label only "DateTime2" label inside UpdatePanel server tag gets updated. So we are now successfully able to update the specific region in ASP.NET web form without updating the other regions and also without getting full page post back.
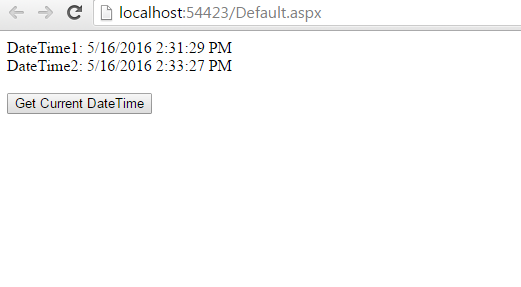
Let's get more idea about this behaviour before that let's have a look at the following part updated mark up.
<asp:UpdatePanel ID="UpdatePanel1" runat="server">
<ContentTemplate>
<div>
DateTime2:
<asp:Label ID="lblDateTime2" runat="server"></asp:Label>
</div>
<br />
<div>
<asp:Button ID="btnGetCurrentDateTime" runat="server"
Text="Get Current DateTime"
OnClick="btnGetDateTime_Click" />
</div>
</ContentTemplate>
</asp:UpdatePanel>
In the above markup we have wrapped up a label "lblDateTime2" and button "Get Current DateTime" inside UpdatePanel control, so we have actually specified area that will get updated without refreshing the whole page. As you have an idea that clicking on asp.net button control post back the form, but when "Get Current DateTime" button is clicked inside an UpdatePanel, it will cause asynchronous post back resulting in partial update of only region enclosed inside this UpdatePanel.
Asynchronous post back refers to process of transfering only selected area in web form between server and client (browser) without affecting other parts of the web form. In our example we can say that "Get Current DateTime" button acts as a asynchronous post back trigger as it triggered the asynchronous post back.
In case you have any query or any suggestion, please feel free to drop your valuable comments. Hope now beginners can have a basic idea of how partial updates are achieved in ASP.NET web form using UpdatePanel control.
0 Comment(s)