IComparable interface is used for sorting of list of objects. It can be similar to Array.Sort() method but the difference is that it provides customization on sorting of objects.
We can sort a list of string or integer by simply calling List.Sort() method. In this case, we don`t need IComparable interface but when we sort a list of objects on basis of a property then we will need to implement the IComparable interface.
Let’s understand it by the help of examples.
Suppose we have a class Item with following properties
class Item
{
public string Name { get; set; }
public int Price { get; set; }
}
Now we will create a list of Items and will try to sort it.
List<Item> itemList = new List<Item>()
{
new Item { Name = "Item1", Price = 1000 },
new Item { Name = "Item2", Price = 2000 },
new Item { Name = "Item3", Price = 1500 }
};
try
{
itemList.Sort();
foreach (var item in itemList)
{
Console.WriteLine($"{nameof(item.Name)} : {item.Name}, {nameof(item.Price)} : {item.Price}");
}
}
catch (Exception ex)
{
Console.Write(ex);
}
Console.Read();
This code will throw an error
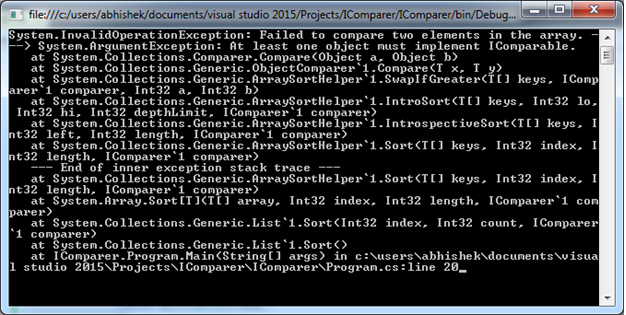
As we can see in above error, List.Sort() will only work on the objects of those classes who implement IComparable interface. Most of the default types in c# implements IComparable. But for custom class objects, we must implement IComparable interface. When we implement IComparable interface, we must define a function CompareTo in the implementing class as this method will be used for comparison.
Now, let`s implement the IComparable interface in class Item.
class Item : IComparable<Item>
{
public string Name { get; set; }
public int Price { get; set; }
public int CompareTo(Item other)
{
if (this.Price > other.Price) return 1;
else if (this.Price < other.Price) return -1;
else return 0;
}
}
In above code, we have implemented IComparable interface and we have also added the CompareTo method. In CompareTo method, we are comparing the item object by their price.
Here is the final output of the program

0 Comment(s)