This is a small sample of how we can split an image into multiple pieces/tiles and store these small pieces into an array and further we will show, how these pieces are rearranged to form an image.
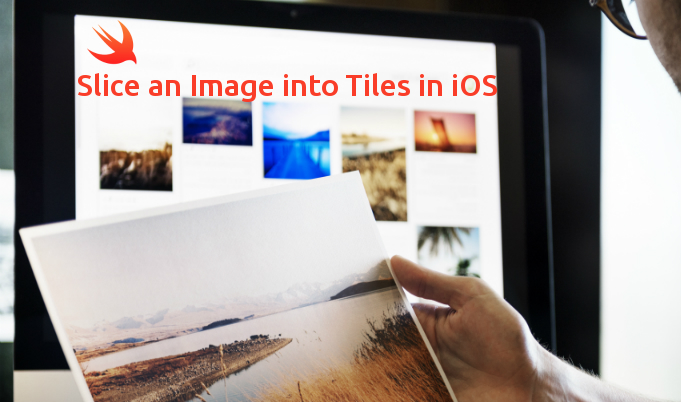
So let start with slicing our image into small pieces or tiles.
Here we will split an image into n X m pieces. Pass number of rows and column you want to slice your image in splitImage function.
func splitImage(row : Int , column : Int){
let oImg = imgView.image
let height = (imgView.image?.size.height)! / CGFloat (row) //height of each image tile
let width = (imgView.image?.size.width)! / CGFloat (column) //width of each image tile
let scale = (imgView.image?.scale)! //scale conversion factor is needed as UIImage make use of "points" whereas CGImage use pixels.
imageArr = [[UIImage]]() // will contain small pieces of image
for y in 0..<row{
var yArr = [UIImage]()
for x in 0..<column{
UIGraphicsBeginImageContextWithOptions(
CGSize(width:width, height:height),
false, 0)
let i = oImg?.cgImage?.cropping(to: CGRect.init(x: CGFloat(x) * width * scale, y: CGFloat(y) * height * scale , width: width * scale , height: height * scale) )
let newImg = UIImage.init(cgImage: i!)
yArr.append(newImg)
UIGraphicsEndImageContext();
}
imageArr.append(yArr)
}
}
Once done with the above code we will get an array of image tiles now its time to rearrange these small tiles into an image. You can also shuffle the array of tiles image to make it an image puzzle. So here is the code for the same:
func createNewImage(imgArr : [[UIImage]]){
let row = imageArr.count
let column = imageArr[0].count
let height = (imgView.frame.size.height) / CGFloat (row )
let width = (imgView.frame.size.width) / CGFloat (column )
UIGraphicsBeginImageContext(CGSize.init(width: imgView.frame.size.width , height: imgView.frame.size.height))
for y in 0..<row{
for x in 0..<column{
let newImage = imgArr[y][x]
newImage.draw(in: CGRect.init(x: CGFloat(x) * width, y: CGFloat(y) * height , width: width - 1 , height: height - 1 ))
}
}
let originalImg = UIGraphicsGetImageFromCurrentImageContext()
UIGraphicsEndImageContext();
imgView.image = originalImg
}
Thanks
1 Comment(s)