Thread synchronization is used to verify that no two or more synchronous processes or threads simultaneously executes some particular program block. When one thread executes in the critical section then it does not allow the another thread to execute in critical section until previous thread will not complete its task in critical section. To do the thread synchronization we use a locking techniques.
Basically, we use a two kinds of locking techniques:-
1. Exclusive Lock
2. Non-Exclusive Lock
Semaphore uses a Non-Exclusive Lock. This lock notifies the other users that we are considering changing the file. Other users can modify the file, if we have a non-exclusive lock on that file. Semaphore does not allow the number of simultaneous users to access the shared resources when the maximum limit reaches.
Semaphore has a certain capacity, once this capacity reached to its maximum limit then no more users can enters and wait in a queue until, one of the user leave its access on the shared resources.
Example:
In this example we uses a semaphore which allow the thread up-to its maximum capacity 5 but there are 8 threads which tried to enter and execute its task in the critical section.
using System;
using System.Text;
using System.Threading.Tasks;
using System.Threading;
namespace SemaphoreExample
{
public class SempahoreProgram
{
static Semaphore semaphore = new Semaphore(1,5);
public static void ExecutionStart()
{
for (int i = 1; i <= 8; i++)
{
new Thread(EnterInCriticalSection).Start(i);
}
}
private static void EnterInCriticalSection(object id)
{
Console.WriteLine("{0} wants to enter", id);
semaphore.WaitOne();
Console.WriteLine("Id {0} is in!", id);
Thread.Sleep(1000 * (int)id);
Console.WriteLine("{0} is leaving", id);
semaphore.Release();
}
}
}
class program
{
public static void main()
{
SempahoreProgram.ExecutionStart();
Console.ReadKey();
}
}
Output:
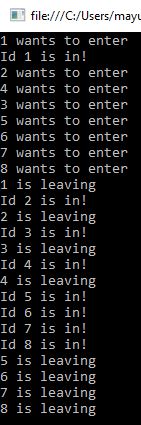
0 Comment(s)