Partial view in asp.net mvc provides the reusability feature in the application. We can use our partial view in layout page which works as a master page for all the views of our application.
To understand it better, First we take the Example of default given Partial view in asp.net MVC application.
Go to your share folder and open _Layout.cshtml page.
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>@ViewBag.Title - My ASP.NET Application</title>
@Styles.Render("~/Content/css")
@Scripts.Render("~/bundles/modernizr")
</head>
<body style="background-color:#DCEBF9">
<div class="navbar navbar-inverse navbar-fixed-top">
<div class="container">
<div class="navbar-header">
<button type="button" class="navbar-toggle" data-toggle="collapse" data-target=".navbar-collapse">
<span class="icon-bar"></span>
<span class="icon-bar"></span>
<span class="icon-bar"></span>
</button>
</div>
<div class="navbar-collapse collapse">
<ul class="nav navbar-nav">
<li>@Html.ActionLink("Home", "Index", "Home")</li>
<li>@Html.ActionLink("About", "About", "Home")</li>
<li>@Html.ActionLink("Contact", "Contact", "Home")</li>
</ul>
@Html.Partial("_LoginPartial")
</div>
</div>
</div>
<div class="container body-content">
@RenderBody()
<hr />
<footer>
<p>© @DateTime.Now.Year - My ASP.NET Application</p>
</footer>
</div>
@Scripts.Render("~/bundles/jquery")
@Scripts.Render("~/bundles/bootstrap")
@RenderSection("scripts", required: false)
</body>
</html>
You can check here,
@Html.Partial("_LoginPartial")
@Html.Partial("_LoginPartial") is the login Partial view,you can check in share folder with the name _LoginPartial.cshtml
@using Microsoft.AspNet.Identity
@if (Request.IsAuthenticated)
{
using (Html.BeginForm("LogOff", "Account", FormMethod.Post, new { id = "logoutForm", @class = "navbar-right" }))
{
@Html.AntiForgeryToken()
<ul class="nav navbar-nav navbar-right">
<li>
@Html.ActionLink("Hello " + User.Identity.GetUserName() + "!", "Index", "Manage", routeValues: null, htmlAttributes: new { title = "Manage" })
</li>
<li><a href="javascript:document.getElementById('logoutForm').submit()">Log off</a></li>
</ul>
}
}
else
{
<ul class="nav navbar-nav navbar-right">
<li>@Html.ActionLink("Register", "Register", "Account", routeValues: null, htmlAttributes: new { id = "registerLink" })</li>
<li>@Html.ActionLink("Log in", "Login", "Account", routeValues: null, htmlAttributes: new { id = "loginLink" })</li>
</ul>
}
Here Register and Login Functionalities are created because it can be used in the project for more than one time. So wherever we need Login functionality we can use the partialview using @html.partial(“Partialviewname”) in any of the view.
We can also use this partial view in _Layout.cshtml if we want to display it in header or footer part as it's done by MVC in its default functionality.
So you can use layout in your view via using:
@{
Layout = "~/Views/Shared/_Layout.cshtml";
}
It will render that common layout on your view with that partial login shown above.
I have created an action result Index in the controller and add a view for this using Layout:
@model MvcPracticeWebApplication.Models.Students
@{
Layout = "~/Views/Shared/_Layout.cshtml";
ViewBag.Title = "Index";
}
@using (Html.BeginForm("Index", "Students", FormMethod.Post, new { @id = "SubmitForm" }))
{
@Html.AntiForgeryToken()
<div class="form-horizontal">
<hr />
@Html.ValidationSummary(true, "", new { @class = "text-danger" })
<div class="form-group">
@Html.LabelFor(model => model.FirstName, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.FirstName, new { htmlAttributes = new { @class = "form-control" } })
@Html.ValidationMessageFor(model => model.FirstName, "", new { @class = "text-danger" })
</div>
</div>
<div class="form-group">
@Html.LabelFor(model => model.LastName, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.LastName, new { htmlAttributes = new { @class = "form-control" } })
@Html.ValidationMessageFor(model => model.LastName, "", new { @class = "text-danger" })
</div>
</div>
<div class="form-group">
@Html.LabelFor(model => model.EmailId, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.EmailId, new { htmlAttributes = new { @class = "form-control" } })
@Html.ValidationMessageFor(model => model.EmailId, "", new { @class = "text-danger" })
</div>
</div>
<div class="form-group">
@Html.LabelFor(model => model.Phones, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.Phones, new { htmlAttributes = new { @class = "form-control" } })
@Html.ValidationMessageFor(model => model.Phones, "", new { @class = "text-danger" })
<input id="btnAdd" type="button" value="Add More +" />
<div class="form-group">
<div class="col-md-offset-2 col-md-10">
<input type="submit" value="Create" class="btn btn-default" id="btnCreate" />
</div>
</div>
</div>
}
<div>
@Html.ActionLink("Back to List", "Index")
</div>
Debug your App and see the result: Default MVC layout is used in index view of student controller. so in any of the view of our application,we can use this layout for reusability purpose as we can See the image for reference
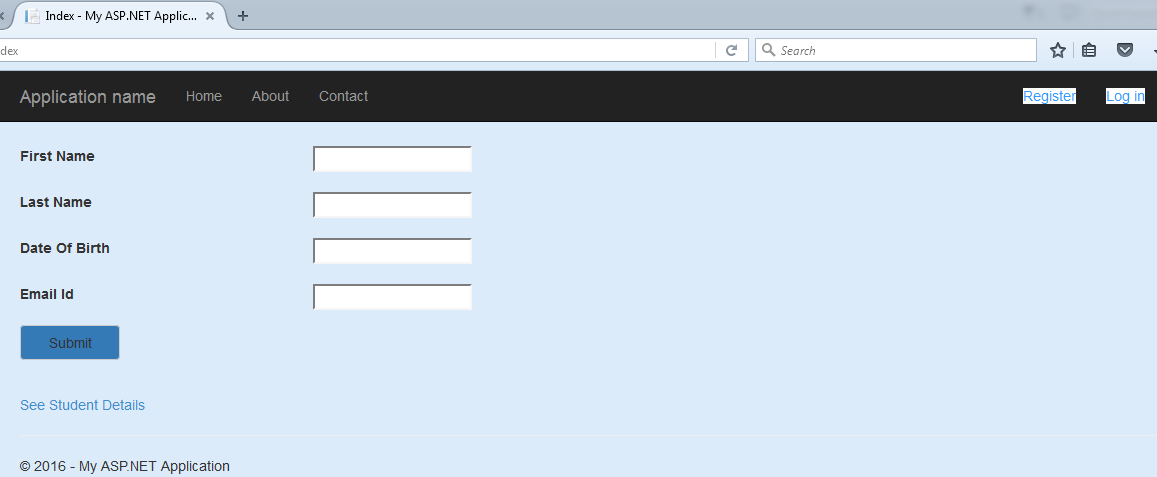
we have seen Partial view is used in _Layout.cshtml to render it with header/footer/common part for every view. But Partial view can also be used in any of the views where the functionality of that partial view is required.
See I add a partial view:Go to your share folder,right click,Add new item,add view.
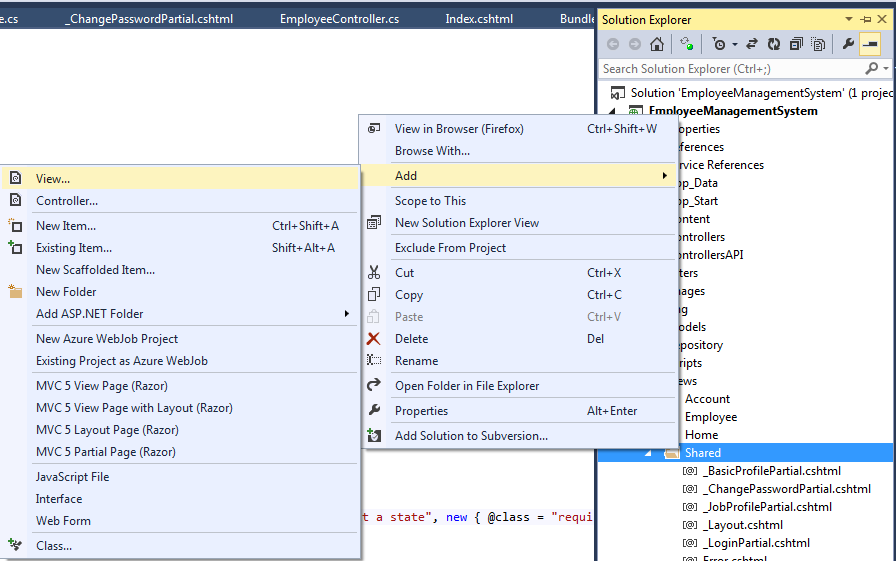
Select radiobutton Create as a partial view. You can also create a strongly bind partial view,so select your model class from your application.See the image for reference:
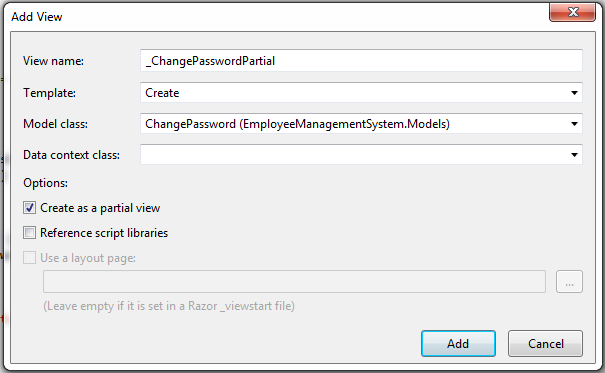
I have created a _changepasswordpartialview which has the functionality to change the old password.
@model EmployeeManagementSystem.Models.ChangePassword
@using (Html.BeginForm("ChangePassword", "Employee", FormMethod.Post))
{
@Html.AntiForgeryToken()
@Html.ValidationSummary(true)
<fieldset>
<legend>Reset Password Form</legend>
<br /><br /><br />
<ol>
<li>
@Html.LabelFor(m => m.NewPassword)
@Html.PasswordFor(m => m.NewPassword, new { @style = "width:310px;height:31px" })
@Html.ValidationMessageFor(m => m.NewPassword)
</li>
<li>
@Html.LabelFor(m => m.ConfirmPassword)
@Html.PasswordFor(m => m.ConfirmPassword, new { @style = "width:310px;height:31px" })
@Html.ValidationMessageFor(m => m.ConfirmPassword)
</li>
</ol>
<input type="submit" value="Reset" style="margin-left: 3%;"/>
</fieldset>
}
So wherever in the application we need changepassword functionality we can reuse this partialview with a single line code as:
@html.partial(_changepasswordpartial)
See I have used the same in my project on the main profile page,there are three partial views basicprofile, jobprofile, and changepassword which are used in project multiple times,so all these functionalities are created in partialviews,so it provides reusability and saves the time and effort of the user.
By using _Layout.cshtml we are also using @html.partail(“_loginpartial”).
@{
Layout = "~/Views/Shared/_Layout.cshtml";
}
<div class="container">
<ul class="nav nav-tabs">
<li class="active"><a data-toggle="tab" href="#menu1">Basic Profile</a></li>
<li><a data-toggle="tab" href="#menu2">Job Profile </a></li>
<li><a data-toggle="tab" href="#menu3">Change Password</a></li>
</ul>
<div class="tab-content">
<div id="home" class="tab-pane fade in active">
</div>
<div id="menu1" class="tab-pane fade in active">
@Html.Partial("_BasicProfilePartial", emp)
</div>
<div id="menu2" class="tab-pane fade">
@Html.Partial("_JobProfilePartial", job)
</div>
<div id="menu3" class="tab-pane fade">
@Html.Partial("_ChangePasswordPartial", changePassword)
<div id="PwdConfirmation">
</div>
</div>
</div>
</div>
See the image for reference:
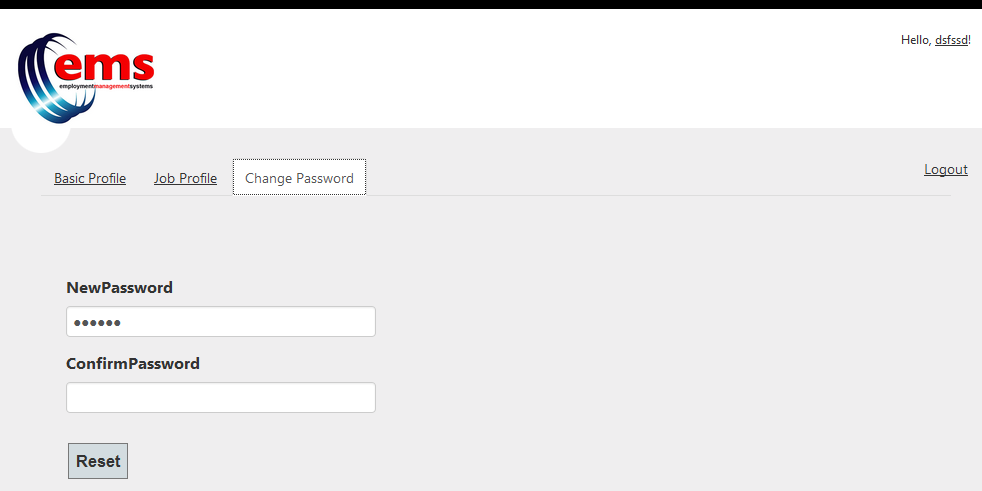
0 Comment(s)